Unlocking the Power of Gatsby: Advanced Techniques and Best Practices
Gatsby has emerged as one of the leading static site generators, providing developers with a powerful toolset for building high-performance websites. While Gatsby offers a range of features out of the box, there are advanced techniques and best practices that can further enhance your development workflow and unlock the full potential of this framework. In this article, we will explore some of these techniques and best practices, providing you with the knowledge to take your Gatsby projects to the next level.
1. Optimizing Performance
1.1 Asset Optimization
Gatsby provides various ways to optimize your site’s assets and improve performance. One effective technique is to utilize the gatsby-image plugin, which optimizes images and lazy loads them to enhance loading times. Here’s an example of using gatsby-image in a Gatsby project:
javascript import { graphql, useStaticQuery } from 'gatsby' import Img from 'gatsby-image' const MyComponent = () => { const data = useStaticQuery(graphql` query { myImage: file(relativePath: { eq: "my-image.jpg" }) { childImageSharp { fluid(maxWidth: 800) { ...GatsbyImageSharpFluid } } } } `) return <Img fluid={data.myImage.childImageSharp.fluid} alt="My Image" /> }
1.2 Code Splitting
Code splitting is a technique that enables you to load only the necessary JavaScript and CSS for a specific page, reducing the initial load time. Gatsby achieves code splitting automatically for you. However, you can further optimize it by utilizing the @loadable/component package. Here’s an example of using @loadable/component in a Gatsby project:
javascript import loadable from '@loadable/component' const DynamicComponent = loadable(() => import('./DynamicComponent')) const MyPage = () => { return ( <div> <h1>Welcome to My Page</h1> <DynamicComponent /> </div> ) }
2. Utilizing Plugins
Gatsby’s plugin ecosystem is extensive, offering a wide range of plugins to extend the functionality of your site. Let’s explore a couple of plugins that can enhance your Gatsby projects.
2.1 Offline Support with gatsby-plugin-offline
The gatsby-plugin-offline plugin enables your Gatsby site to work offline, providing a seamless experience for users even when they have limited or no internet connectivity. To use this plugin, install it and add it to your gatsby-config.js file:
javascript module.exports = { plugins: [ 'gatsby-plugin-offline', ], }
2.2 SEO Optimization with gatsby-plugin-react-helmet
The gatsby-plugin-react-helmet plugin simplifies the process of adding meta tags, title tags, and other SEO-related elements to your Gatsby site. Here’s an example of adding metadata to a Gatsby project using this plugin:
javascript // In your gatsby-config.js module.exports = { plugins: [ 'gatsby-plugin-react-helmet', ], } // In your component import { Helmet } from 'react-helmet' const MyComponent = () => ( <div> <Helmet> <title>My Page Title</title> <meta name="description" content="This is my page description." /> </Helmet> {/* Rest of the component */} </div> )
3. Creating Dynamic Content
Gatsby allows you to create dynamic content by integrating with various data sources. Let’s explore a couple of techniques for creating dynamic content in Gatsby.
3.1 Querying Data with GraphQL
Gatsby utilizes GraphQL to query and retrieve data from various sources. You can create GraphQL queries in your components to fetch data and render it dynamically. Here’s an example of querying and rendering data in a Gatsby component:
javascript import { graphql, useStaticQuery } from 'gatsby' const MyComponent = () => { const data = useStaticQuery(graphql` query { allPosts { edges { node { title excerpt } } } } `) return ( <div> {data.allPosts.edges.map(({ node }) => ( <div key={node.title}> <h2>{node.title}</h2> <p>{node.excerpt}</p> </div> ))} </div> ) }
3.2 Using Gatsby Node APIs
Gatsby’s Node APIs allow you to programmatically create pages and transform data during the build process. You can leverage these APIs to generate dynamic content and customize your Gatsby site. Here’s an example of creating pages dynamically using the createPages API:
javascript exports.createPages = async ({ graphql, actions }) => { const { createPage } = actions const result = await graphql(` query { allPosts { edges { node { slug } } } } `) result.data.allPosts.edges.forEach(({ node }) => { createPage({ path: `/posts/${node.slug}`, component: path.resolve('./src/templates/post.js'), context: { slug: node.slug, }, }) }) }
Conclusion
By implementing these advanced techniques and following best practices, you can unlock the true power of Gatsby, delivering blazing-fast, feature-rich websites. Experiment with these techniques, explore the extensive plugin ecosystem, and keep optimizing your Gatsby projects to create outstanding user experiences. Happy coding!
Table of Contents
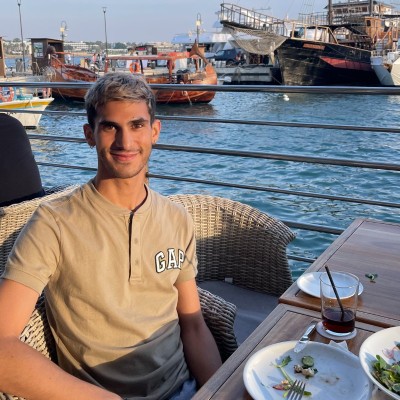
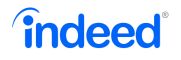