Building E-commerce Websites with Gatsby and Shopify
In today’s digital age, e-commerce websites play a crucial role in reaching customers and driving sales for businesses of all sizes. To succeed in the competitive online marketplace, it’s essential to have a fast, reliable, and flexible e-commerce platform. In this blog, we’ll explore the winning combination of Gatsby and Shopify for building high-performance e-commerce websites that provide a seamless user experience.
Why Choose Gatsby and Shopify?
Gatsby and Shopify are two powerful technologies, each bringing unique advantages to the table. Gatsby is a modern, fast, and highly performant static site generator built on React. It leverages the power of GraphQL to fetch data efficiently and pre-renders pages, resulting in blazing-fast load times and excellent SEO. On the other hand, Shopify is a leading e-commerce platform that provides a robust backend for managing products, orders, and payments, making it a popular choice among online retailers.
Combining Gatsby and Shopify allows developers to create e-commerce websites that offer the best of both worlds: the performance and flexibility of Gatsby’s static site generation and the powerful e-commerce capabilities of Shopify.
Setting Up Your Development Environment
Before diving into the code, you’ll need to set up your development environment. Ensure that you have Node.js and npm (Node Package Manager) installed. Additionally, create accounts on both Gatsby and Shopify platforms to access the necessary APIs and functionalities.
Once your development environment is ready, you can start building your e-commerce website with Gatsby and Shopify.
Step 1: Creating a New Gatsby Project
First, let’s create a new Gatsby project. Open your terminal and run the following commands:
bash # Install Gatsby CLI globally if you haven't already npm install -g gatsby-cli # Create a new Gatsby project gatsby new my-ecommerce-website cd my-ecommerce-website
Step 2: Install Dependencies
Next, let’s install the required dependencies for integrating Gatsby with Shopify. Run the following commands in your project directory:
bash npm install gatsby-source-shopify npm install dotenv
The gatsby-source-shopify plugin allows Gatsby to fetch data from your Shopify store, and dotenv helps manage environment variables securely.
Step 3: Setting Up Shopify API
To access data from your Shopify store, you need to generate private API credentials. Follow these steps:
- Login to your Shopify admin.
- Navigate to Settings > API keys.
- Under the Private app section, click on “Create a new private app.”
- Fill in the required details, and once the app is created, you’ll see your API credentials.
Copy the API credentials, as you’ll need them in the next step.
Step 4: Configuring Environment Variables
It’s essential to keep sensitive information like API credentials secure. We’ll use environment variables to store this information. Create a .env file in your project’s root directory and add the following:
dotenv SHOPIFY_SHOP_NAME=your-shopify-shop-name SHOPIFY_ACCESS_TOKEN=your-shopify-access-token
Replace your-shopify-shop-name and your-shopify-access-token with your actual Shopify shop name and the access token obtained from the previous step.
Step 5: Configuring Gatsby to Use Shopify Source Plugin
In your Gatsby project, open the gatsby-config.js file and add the following configuration:
javascript module.exports = { siteMetadata: { title: "My E-commerce Website", description: "Amazing products at fantastic prices!", }, plugins: [ { resolve: "gatsby-source-shopify", options: { shopName: process.env.SHOPIFY_SHOP_NAME, accessToken: process.env.SHOPIFY_ACCESS_TOKEN, }, }, ], };
Here, we’re using the gatsby-source-shopify plugin and providing the shop name and access token using environment variables.
Step 6: Fetching Data from Shopify
With the Shopify source plugin configured, Gatsby can now fetch data from your Shopify store. You can use GraphQL queries to retrieve the data you need. For example, to fetch all products, create a new file named products.js in the src/pages directory with the following content:
jsx import React from "react"; import { graphql } from "gatsby"; const Products = ({ data }) => { const products = data.allShopifyProduct.nodes; return ( <div> <h1>Products</h1> <ul> {products.map((product) => ( <li key={product.id}> <h3>{product.title}</h3> <p>{product.description}</p> <p>Price: {product.price}</p> </li> ))} </ul> </div> ); }; export const query = graphql` { allShopifyProduct { nodes { id title description price } } } `; export default Products;
In this example, we’re fetching product data using GraphQL and displaying a list of products with their titles, descriptions, and prices.
Step 7: Styling Your E-commerce Website
While Gatsby provides a solid foundation for building your e-commerce site, you’ll likely want to customize the design to match your brand. You can use various styling options, such as CSS-in-JS libraries like Emotion or Styled-components, or import your CSS files.
Step 8: Implementing Shopping Cart and Checkout
To turn your static e-commerce website into a fully functional online store, you’ll need to add a shopping cart and checkout functionality. Shopify provides JavaScript Buy SDKs that you can use to implement these features.
For example, to add a simple shopping cart, create a new file named Cart.js and add the following code:
jsx import React from "react"; import { useCart } from "gatsby-theme-shopify-manager"; const Cart = () => { const cart = useCart(); return ( <div> <h2>Cart</h2> {cart.lineItems.map((item) => ( <div key={item.id}> <h4>{item.title}</h4> <p>Quantity: {item.quantity}</p> <p>Price: {item.variant.price}</p> </div> ))} </div> ); }; export default Cart;
In this example, we’re using the gatsby-theme-shopify-manager package, which simplifies working with Shopify’s JavaScript Buy SDK. The useCart hook provides access to the user’s cart data, and we display the cart items, quantities, and prices.
Step 9: Deploying Your E-commerce Website
After building your e-commerce website, you’re ready to take it live! Gatsby websites are well-suited for various hosting options, including popular platforms like Netlify, Vercel, or deploying to your server.
Conclusion
Building e-commerce websites with Gatsby and Shopify is a powerful combination that offers the best of both worlds: the speed and flexibility of Gatsby’s static site generation and the robust e-commerce capabilities of Shopify. By following the steps outlined in this blog and using the provided code samples, you can create a high-performance online store that delivers a seamless shopping experience for your customers. Happy coding!
Table of Contents
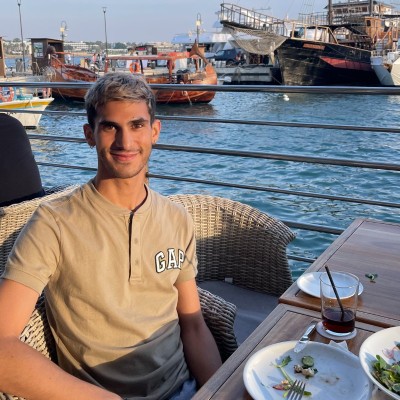
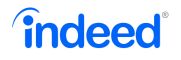