Creating Dynamic Web Apps with Gatsby and React
In today’s digital landscape, creating dynamic web applications with exceptional performance and user experience is crucial. Gatsby, a powerful static site generator, combined with the flexibility of React, offers a compelling solution. In this blog post, we will explore how to leverage Gatsby and React to create dynamic web apps that load quickly, respond swiftly, and deliver a seamless user experience. From setting up a Gatsby project to implementing dynamic data fetching and handling user interactions, we’ll cover all the essentials you need to know. Let’s dive in!
What is Gatsby?
Gatsby is a powerful open-source framework built on top of React that allows you to create blazing-fast websites and web applications. It leverages the concept of static site generation to pre-build your pages and assets, resulting in lightning-fast loading times. Gatsby also excels at handling dynamic data by utilizing GraphQL, a query language for APIs. With its vast ecosystem of plugins and themes, Gatsby provides a robust foundation for creating scalable and performant web apps.
Setting up a Gatsby Project
To get started with Gatsby, you’ll need to have Node.js installed on your machine. Once installed, you can use the Gatsby CLI to set up a new project. Run the following command in your terminal:
bash npx gatsby new my-gatsby-app
This command creates a new Gatsby project in a directory named “my-gatsby-app.” Navigate into the project directory by running:
bash cd my-gatsby-app
Next, start the development server by executing:
bash npm run develop
Creating React Components
Gatsby allows you to build your web application using React components. Start by creating a new folder called src at the root of your project. Inside the src folder, create a new file called index.js and add the following code:
jsx import React from "react" const IndexPage = () => { return ( <div> <h1>Welcome to my Gatsby app!</h1> <p>This is a dynamic web application built with Gatsby and React.</p> </div> ) } export default IndexPage
This code defines a simple React component that renders a heading and a paragraph. You can create additional components and organize them within the src directory based on your application’s structure.
Implementing Dynamic Data Fetching
Gatsby leverages GraphQL to fetch and manage data from various sources. To implement dynamic data fetching, create a new file called gatsby-node.js in the root of your project and add the following code:
javascript exports.createPages = async ({ actions, graphql }) => { const { data } = await graphql(` query { allPosts { edges { node { id title } } } } `) data.allPosts.edges.forEach(({ node }) => { actions.createPage({ path: `/posts/${node.id}`, component: require.resolve("./src/templates/post.js"), context: { postId: node.id, }, }) }) }
In this code snippet, we use the createPages API provided by Gatsby to fetch data using GraphQL and create dynamic pages for each post. The createPage function generates a new page at the specified path and associates it with a React component, which in this case is post.js. You can customize this code to fetch data from your desired source and create dynamic pages based on your application’s requirements.
Routing and Navigation
Gatsby provides built-in routing and navigation capabilities to help users navigate your web app seamlessly. To implement navigation, install the gatsby-plugin-react-router package:
bash npm install gatsby-plugin-react-router
Next, open the gatsby-config.js file at the root of your project and add the following code:
javascript module.exports = { plugins: [ "gatsby-plugin-react-router", ], }
Now you can use React Router components such as Link and Switch to create links and define routes within your React components.
Handling User Interactions
With Gatsby and React, you can easily handle user interactions to create dynamic and responsive web applications. React’s event handling system allows you to capture and respond to user actions. For example, you can add an event listener to a button click and update the component state accordingly. Here’s an example:
jsx import React, { useState } from "react" const Counter = () => { const [count, setCount] = useState(0) const increment = () => { setCount(count + 1) } return ( <div> <h1>Counter: {count}</h1> <button onClick={increment}>Increment</button> </div> ) } export default Counter
In this code snippet, we use the useState hook to create a state variable count and a function setCount to update it. The increment function updates the count when the button is clicked, and the component re-renders to reflect the updated state.
Optimizing Performance
To ensure optimal performance, Gatsby offers various optimization techniques. You can implement lazy loading for images using the gatsby-image package, which loads images only when they come into view. Additionally, Gatsby optimizes image assets automatically by generating multiple versions with different resolutions.
Another crucial aspect of performance optimization is code splitting. Gatsby automatically applies code splitting, generating separate bundles for each page. This ensures that only the necessary code is loaded, resulting in faster initial load times.
Deployment and Continuous Integration
Gatsby makes deployment and continuous integration seamless. You can deploy your Gatsby app to popular hosting platforms such as Netlify, Vercel, or AWS Amplify. These platforms provide straightforward integrations with Git, allowing for easy deployment whenever you push changes to your repository.
You can also configure continuous integration pipelines to automate the build and deployment process. Services like Netlify and Vercel offer built-in CI/CD pipelines that trigger on every commit, ensuring your web app is always up to date.
Conclusion
With Gatsby and React, you have the power to create dynamic web applications that offer exceptional performance and user experience. By leveraging Gatsby’s static site generation and React’s flexibility, you can build fast-loading pages, handle dynamic data, and create engaging user interactions. Whether you’re building a personal blog, an e-commerce site, or a complex web app, Gatsby and React provide the tools you need to create remarkableexperiences for your users. So, start exploring the world of Gatsby and React and unlock the potential of dynamic web app development today!
Remember to optimize your app for performance, leverage dynamic data fetching with GraphQL, implement routing and navigation for seamless user flow, and handle user interactions using React’s event handling system. With these techniques, you’ll be well on your way to creating dynamic web apps that stand out from the crowd.
Happy coding!
Table of Contents
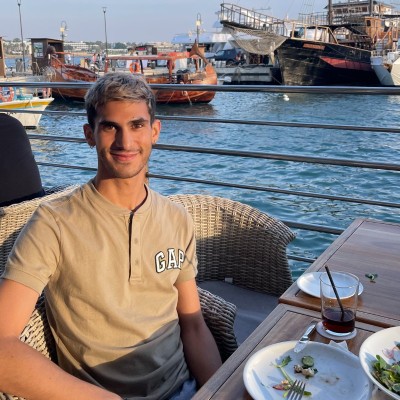
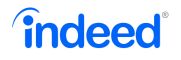