Gatsby and Firebase: Building Real-time Web Applications
In the modern web development landscape, building real-time web applications that deliver an exceptional user experience is a top priority. Gatsby and Firebase are two powerful tools that, when combined, can help developers achieve this goal effortlessly. Gatsby, a popular static site generator built on React, ensures high performance and fast-loading websites. Firebase, on the other hand, is a flexible and scalable platform that offers real-time data synchronization and a wide range of services for app development. In this blog post, we will explore how to leverage the strengths of Gatsby and Firebase to build real-time web applications that respond to user actions instantly.
1. What is Gatsby?
1.1 Introduction to Gatsby
Gatsby is a modern static site generator that uses React to build blazing-fast websites and applications. It follows the JAMstack architecture, where JavaScript, APIs, and Markup are decoupled, resulting in improved performance and security. Gatsby generates static HTML and assets at build time, making it easy to serve the content from a CDN and reducing server load. This approach enables near-instantaneous page loads and an excellent user experience.
1.2 Advantages of Gatsby
- Speed: Gatsby’s pre-rendered content and optimized loading processes ensure rapid page loads, reducing bounce rates and keeping visitors engaged.
- SEO-friendly: Gatsby produces SEO-friendly websites by delivering fully optimized HTML and offering plugins to handle metadata and sitemaps effortlessly.
- Developer Experience: Gatsby’s developer-friendly environment, hot-reloading, and vast plugin ecosystem make it enjoyable to work with.
1.3 Setting up a Gatsby project
To create a Gatsby project, you need Node.js and npm (Node Package Manager) installed on your machine. If you don’t have them, download and install them from the official website.
Once you have Node.js and npm ready, you can create a new Gatsby project using the Gatsby CLI (Command Line Interface). Open your terminal or command prompt and execute the following commands:
bash # Install the Gatsby CLI globally (if you haven't already) npm install -g gatsby-cli # Create a new Gatsby project gatsby new my-gatsby-app
This will scaffold a new Gatsby project in the “my-gatsby-app” directory. Navigate to the project folder using:
bash cd my-gatsby-app
Now, you can start the development server with the following command:
bash gatsby develop
Gatsby will compile your site and start a development server. By default, it will be available at http://localhost:8000. Open this URL in your browser, and you should see your Gatsby site up and running.
2. What is Firebase?
2.1 Introduction to Firebase
Firebase is a comprehensive platform for building web and mobile applications developed by Google. It offers various services that simplify and accelerate app development. One of its key features is the real-time database, which enables data synchronization across clients in real-time. This makes Firebase an excellent choice for applications that require instant updates, such as collaborative tools and chat applications.
2.2 Core features of Firebase
- Real-time Database: Firebase provides a NoSQL cloud database that syncs data in real-time across connected clients.
- Authentication: It offers built-in authentication services, including email/password, social login, and anonymous authentication.
- Hosting: Firebase Hosting allows developers to deploy web apps with a single command, backed by a global CDN for fast delivery.
- Cloud Functions: Firebase Cloud Functions enable serverless backend logic, reducing the need for a separate backend infrastructure.
- Storage: Firebase Storage offers secure cloud storage for user-generated content like images, videos, and files.
2.3 Setting up a Firebase project
To use Firebase in your Gatsby application, you need to create a Firebase project and obtain the necessary configuration details.
2.3.1 Create a Firebase Project:
- Go to the Firebase Console (https://console.firebase.google.com/) and log in with your Google account.
- Click on the “Add project” button and provide a name for your project.
- Choose your preferred options for Google Analytics and other settings, then click “Create project.”
2.3.2 Set up Firebase in Your Gatsby Project:
1. Install the Firebase SDK and related packages by running the following command in your Gatsby project directory:
bash npm install firebase
2. Next, navigate to your Firebase project’s settings in the Firebase Console and click on the “Project settings” gear icon.
3. In the “Your apps” section, click on the “</>” icon to add a new web app to your project.
4. Register the app by providing a nickname (e.g., “Gatsby App”) and click “Register app.”
5. Firebase will generate a configuration object with credentials. Copy the configuration details, which look like the following:
javascript const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_PROJECT_ID.firebaseapp.com", databaseURL: "https://YOUR_PROJECT_ID.firebaseio.com", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_PROJECT_ID.appspot.com", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID", };
6. Now, import the Firebase SDK in your Gatsby application’s entry point, typically gatsby-browser.js or gatsby-ssr.js:
javascript import firebase from "firebase/app"; import "firebase/firestore"; // Import additional Firebase services you plan to use
7. Initialize Firebase with the configuration object:
javascript // Make sure to call this only once in your app if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); }
With Firebase set up in your Gatsby project, you can now leverage Firebase’s real-time database and other services to create dynamic web applications.
3. Integrating Firebase with Gatsby
3.1 Creating a Firebase Project
We have already created a Firebase project in the previous section. If you haven’t done that, please refer to the instructions provided earlier.
3.2 Configuring Firebase Credentials
To secure sensitive data, Firebase credentials should not be hard-coded directly in the source code. Instead, we’ll use environment variables to keep them private. We’ll use a package called dotenv to manage these variables.
3.2.1 Install the dotenv package:
bash npm install dotenv
Create a new file in your Gatsby project root called .env. Add your Firebase configuration values to this file in the following format:
plaintext GATSBY_API_KEY=YOUR_API_KEY GATSBY_AUTH_DOMAIN=YOUR_AUTH_DOMAIN GATSBY_DATABASE_URL=YOUR_DATABASE_URL GATSBY_PROJECT_ID=YOUR_PROJECT_ID GATSBY_STORAGE_BUCKET=YOUR_STORAGE_BUCKET GATSBY_MESSAGING_SENDER_ID=YOUR_MESSAGING_SENDER_ID GATSBY_APP_ID=YOUR_APP_ID
Now, access these environment variables in your Gatsby app by modifying the Firebase configuration:
javascript const firebaseConfig = { apiKey: process.env.GATSBY_API_KEY, authDomain: process.env.GATSBY_AUTH_DOMAIN, databaseURL: process.env.GATSBY_DATABASE_URL, projectId: process.env.GATSBY_PROJECT_ID, storageBucket: process.env.GATSBY_STORAGE_BUCKET, messagingSenderId: process.env.GATSBY_MESSAGING_SENDER_ID, appId: process.env.GATSBY_APP_ID, };
3.3 Setting up Firebase Authentication
Firebase provides various authentication methods, such as email/password, Google, Facebook, Twitter, etc. In this example, we’ll implement email/password authentication.
1. Enable Email/Password Authentication:
- Go to the Firebase Console and navigate to “Authentication” in the left-hand menu.
- In the “Sign-in method” tab, enable the “Email/Password” provider.
2. Implement User Registration and Login:
- Create a new folder called “utils” in your Gatsby project’s src directory. Inside this folder, create a new file named firebase.js.
- In the firebase.js file, add the following code to set up Firebase and create functions for user registration and login:
javascript import firebase from "firebase/app"; import "firebase/auth"; // Initialize Firebase with your configuration if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); } // Function to register a new user with email and password export const registerWithEmailAndPassword = async (email, password) => { try { const userCredential = await firebase .auth() .createUserWithEmailAndPassword(email, password); return userCredential.user; } catch (error) { throw error; } }; // Function to log in an existing user with email and password export const loginWithEmailAndPassword = async (email, password) => { try { const userCredential = await firebase .auth() .signInWithEmailAndPassword(email, password); return userCredential.user; } catch (error) { throw error; } };
Now, you can import and use these functions in your application’s components to implement user registration and login.
3.4 Real-time Data Synchronization with Firebase
Firebase’s real-time database allows you to synchronize data across connected clients instantly. Let’s see how to integrate it into our Gatsby application.
3.4.1 Initialize Firebase Database:
- Create a new file called firebase.js inside the “utils” folder.
- Import and initialize Firebase with the same configuration:
javascript import firebase from "firebase/app"; import "firebase/database"; // Import the database module // Initialize Firebase with your configuration (if not already done) if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); }
3.4.2 Writing Data to the Firebase Database:
To write data to the database, call the ref function with the desired database path and use the set function to save data:
javascript // Function to add a new post to the database export const addPost = async (postContent) => { try { const newPostRef = firebase.database().ref("posts").push(); await newPostRef.set({ content: postContent }); return newPostRef.key; // Return the unique key generated for the new post } catch (error) { throw error; } };
Here, we are adding a new post to the “posts” node in the database. The push function generates a unique key for the new post, and we set the post’s content using the set function.
3.4.3 Reading Data from the Firebase Database:
To read data from the database, use the on function to listen for changes at a specific location:
javascript // Function to get all posts from the database export const getPosts = (callback) => { const postsRef = firebase.database().ref("posts"); postsRef.on("value", (snapshot) => { const posts = []; snapshot.forEach((childSnapshot) => { const post = { id: childSnapshot.key, ...childSnapshot.val(), }; posts.push(post); }); callback(posts); }); };
In this example, we use the on function to listen for changes at the “posts” node. Whenever the data changes, the provided callback will be executed, and we update our application’s state with the latest posts.
With Firebase integrated into your Gatsby application, you can now create real-time web applications that deliver instant updates to your users.
4. Building a Real-time Web Application
4.1 Designing the Application Structure
For our example, let’s build a real-time chat application where users can post messages, and all connected users can see the messages in real-time.
4.1.1 Create a New Component for the Chat Room:
1. In your Gatsby project’s “src” directory, create a new folder called “components.”
2. Inside the “components” folder, create a new file called ChatRoom.js.
3. Add the following code to create a basic chat room component:
jsx import React, { useState, useEffect } from "react"; import { addPost, getPosts } from "../utils/firebase"; const ChatRoom = () => { const [message, setMessage] = useState(""); const [posts, setPosts] = useState([]); const handlePostMessage = async () => { if (message.trim() !== "") { await addPost(message); setMessage(""); } }; useEffect(() => { getPosts((allPosts) => { setPosts(allPosts); }); }, []); return ( <div> <div> {posts.map((post) => ( <div key={post.id}> <p>{post.content}</p> </div> ))} </div> <input type="text" value={message} onChange={(e) => setMessage(e.target.value)} /> <button onClick={handlePostMessage}>Send</button> </div> ); }; export default ChatRoom;
4. In this component, we use the useState hook to manage the state of the message input and the list of posts.
5. The handlePostMessage function is called when the “Send” button is clicked. It adds a new post to the Firebase database and clears the message input.
6. The useEffect hook fetches the initial list of posts from the database and updates the application state when new posts are added.
4.1.2 Implementing User Authentication:
1. In your “components” folder, create a new file named Auth.js.
2. Add the following code to create an authentication component:
jsx import React, { useState } from "react"; import { registerWithEmailAndPassword, loginWithEmailAndPassword } from "../utils/firebase"; const Auth = () => { const [email, setEmail] = useState(""); const [password, setPassword] = useState(""); const [isRegistered, setIsRegistered] = useState(true); const handleAuthentication = async () => { try { if (isRegistered) { // Log in existing user await loginWithEmailAndPassword(email, password); } else { // Register a new user await registerWithEmailAndPassword(email, password); } } catch (error) { console.error("Authentication error:", error); } }; return ( <div> <input type="email" placeholder="Email" value={email} onChange={(e) => setEmail(e.target.value)} /> <input type="password" placeholder="Password" value={password} onChange={(e) => setPassword(e.target.value)} /> <button onClick={handleAuthentication}> {isRegistered ? "Log In" : "Register"} </button> <button onClick={() => setIsRegistered(!isRegistered)}> {isRegistered ? "Switch to Register" : "Switch to Log In"} </button> </div> ); }; export default Auth;
3. In this component, we use the useState hook to manage the state of the email, password, and the authentication mode (register or login).
4. The handleAuthentication function is called when the authentication button is clicked. It either registers a new user or logs in an existing user based on the selected mode.
4.1.3 Create the Main Page:
1. In your “components” folder, create a new file named MainPage.js.
2.Add the following code to create the main page component that combines the chat room and authentication components:
jsx import React from "react"; import ChatRoom from "./ChatRoom"; import Auth from "./Auth"; const MainPage = () => { // Assuming you have a state for user authentication status const isAuthenticated = true; return ( <div> {isAuthenticated ? <ChatRoom /> : <Auth />} </div> ); }; export default MainPage;
3. In this component, we render the ChatRoom component if the user is authenticated, and the Auth component if not.
4.2 Creating React Components in Gatsby
Now that we have our basic components ready, let’s create the main layout and routes for the application using Gatsby.
4.2.1 Create a Layout Component:
1. In your “components” folder, create a new file named Layout.js.
2. Add the following code to create a layout component with a header and content area:
jsx import React from "react"; import { Link } from "gatsby"; const Layout = ({ children }) => { return ( <div> <header> <nav> <ul> <li> <Link to="/">Home</Link> </li> </ul> </nav> </header> <main>{children}</main> </div> ); }; export default Layout;
3. In this component, we use the Link component from Gatsby to create a navigation link that leads to the home page.
4.2.2 Set Up Routing in Gatsby:
1. Open the gatsby-browser.js file in the root of your Gatsby project.
2. Import the Layout component and wrap it around the MainPage component:
jsx import React from "react"; import Layout from "./src/components/Layout"; import MainPage from "./src/components/MainPage"; export const wrapRootElement = ({ element }) => { return <Layout>{element}</Layout>; };
3. This ensures that the Layout component is applied to all pages of your application.
4.3 Implementing Real-Time Data Updates
With our components and layout in place, it’s time to display real-time updates from the Firebase database in the chat room.
4.3.1 Display Real-Time Updates in the Chat Room:
1. In your ChatRoom.js component, update the useEffect hook to listen for real-time updates:
jsx useEffect(() => { getPosts((allPosts) => { setPosts(allPosts); }); // Add a listener for real-time updates const postsRef = firebase.database().ref("posts"); postsRef.on("child_added", (snapshot) => { const newPost = { id: snapshot.key, ...snapshot.val(), }; setPosts((prevPosts) => [...prevPosts, newPost]); }); // Clean up the listener on unmount return () => { postsRef.off("child_added"); }; }, []);
2. Now, whenever a new post is added to the Firebase database, the child_added event listener will trigger, and we’ll update our application state with the new post.
4.3.2 Styling the Application with Gatsby and CSS-in-JS:
Gatsby offers various ways to style your application, and one popular approach is to use CSS-in-JS libraries like styled-components.
1. Install styled-components and the required plugins:
bash npm install styled-components gatsby-plugin-styled-components babel-plugin-styled-components
2. In your Gatsby project’s root directory, create a new file named gatsby-config.js.
3. Add the following code to configure the styled-components plugin:
javascript module.exports = { plugins: [ `gatsby-plugin-styled-components`, ], };
4. Now, you can style your components using styled-components:
jsx // In ChatRoom.js import styled from "styled-components"; const ChatWrapper = styled.div` max-width: 600px; margin: 0 auto; padding: 20px; `; const ChatMessage = styled.div` margin-bottom: 10px; `; const ChatRoom = () => { // ... return ( <ChatWrapper> <div> {posts.map((post) => ( <ChatMessage key={post.id}> <p>{post.content}</p> </ChatMessage> ))} </div> {/* ... */} </ChatWrapper> ); };
- Use styled-components to define the styles for your components, and apply them as shown in the example above.
5. Optimizing Performance and Security
Optimizing performance and ensuring security are critical aspects of building real-time web applications. Here are some tips to achieve both:
5.1 Code Splitting in Gatsby:
Gatsby automatically performs code splitting, meaning it loads only the required JavaScript for each page. This results in faster initial page loads. Avoid large dependencies and use dynamic imports for components that are not needed on every page.
5.2 Caching and Data Optimization:
Use Firebase’s caching mechanism to improve data access speed. Additionally, implement client-side data caching in Gatsby using plugins like gatsby-plugin-offline.
5.3 Securing Firebase Data:
Ensure that Firebase database rules are correctly set up to restrict access to sensitive data. Use Firebase authentication to control access to specific data based on the user’s role.
5.4 Implementing User Authentication Best Practices:
Enforce strong password policies and implement two-factor authentication (2FA) for enhanced security. Regularly review and revoke access for inactive users.
Conclusion
By combining the power of Gatsby and Firebase, developers can create real-time web applications that deliver outstanding performance and responsiveness. Gatsby’s static site generation ensures fast loading times, while Firebase’s real-time database allows seamless synchronization of data across connected clients. With the ability to implement real-time chat applications, collaborative tools, and much more, this dynamic duo empowers developers to build cutting-edge web experiences that keep users engaged and delighted.
The ease of setting up a Gatsby project and integrating Firebase’s real-time capabilities make this combination a compelling choice for developers seeking to build modern web applications with top-notch performance and real-time functionality. So, why wait? Start building your real-time web application with Gatsby and Firebase today!
Table of Contents
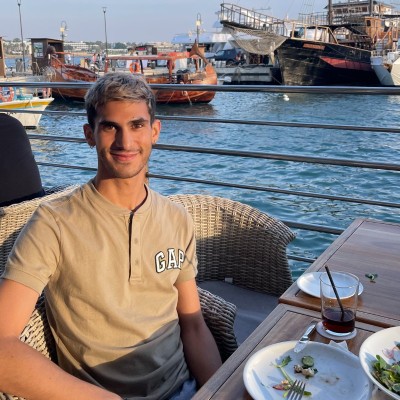
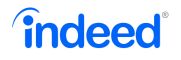