Mastering Gatsby: A Comprehensive Guide for Web Developers
In the ever-evolving world of web development, staying up to date with the latest frameworks and tools is essential. Gatsby, a modern website generator built on React, has gained significant popularity among developers for its speed, scalability, and exceptional performance. Whether you’re a beginner or an experienced developer looking to enhance your skills, this comprehensive guide will take you on a journey to master Gatsby.
Getting Started with Gatsby
Before diving into Gatsby, it’s crucial to have a solid foundation. This section will guide you through the installation process, setting up a development environment, and understanding the core concepts of Gatsby. By the end of this chapter, you’ll have Gatsby up and running and be ready to embark on your journey to mastering this powerful framework.
Code Sample:
perl # Install Gatsby CLI globally npm install -g gatsby-cli # Create a new Gatsby site gatsby new my-gatsby-site # Navigate to the site's directory cd my-gatsby-site # Start the development server gatsby develop
Building a Basic Gatsby Site
In this section, we’ll walk through the process of building a basic Gatsby site. You’ll learn about the folder structure, creating pages using components, and managing site metadata. By the end of this chapter, you’ll have a solid understanding of how to structure and build a simple Gatsby site.
Code Sample:
jsx // src/pages/index.js import React from "react" const IndexPage = () => { return ( <main> <h1>Welcome to my Gatsby site!</h1> <p>Start building amazing websites with Gatsby.</p> </main> ) } export default IndexPage
Creating Dynamic Pages with GraphQL
One of the key features of Gatsby is its integration with GraphQL, which allows you to query and manipulate data with ease. In this section, we’ll explore how to leverage GraphQL to create dynamic pages, fetch data from various sources, and integrate it into your Gatsby site. By the end of this chapter, you’ll have a deep understanding of GraphQL and its role in building powerful Gatsby applications.
Code Sample:
jsx // src/templates/blog-post.js import React from "react" import { graphql } from "gatsby" const BlogPostTemplate = ({ data }) => { const post = data.markdownRemark return ( <article> <h1>{post.frontmatter.title}</h1> <div dangerouslySetInnerHTML={{ __html: post.html }} /> </article> ) } export const query = graphql` query($slug: String!) { markdownRemark(fields: { slug: { eq: $slug } }) { frontmatter { title } html } } ` export default BlogPostTemplate
Styling and Theming in Gatsby
A visually appealing website is crucial for engaging users. Gatsby provides flexible options for styling and theming your applications. In this section, we’ll explore different styling approaches using CSS modules, CSS-in-JS libraries, and popular styling frameworks like Tailwind CSS. By the end of this chapter, you’ll have the skills to create stunning designs and customizable themes for your Gatsby projects.
Code Sample:
jsx // src/components/Header.js import React from "react" import styles from "./header.module.css" const Header = () => { return ( <header className={styles.header}> <h1>My Awesome Gatsby Site</h1> </header> ) } export default Header
Working with Data Sources and APIs
Gatsby offers various ways to fetch data from different sources, including markdown files, headless CMSs, and RESTful APIs. In this section, we’ll explore how to integrate external data sources into your Gatsby site, using plugins, GraphQL, and other techniques. By the end of this chapter, you’ll be able to pull data from multiple sources and leverage it to create dynamic and data-driven applications.
Code Sample:
jsx // gatsby-config.js module.exports = { plugins: [ { resolve: "gatsby-source-graphql", options: { typeName: "MyCMS", fieldName: "mycms", url: "https://example.com/graphql", }, }, ], }
Optimizing Performance and SEO
Performance and SEO are crucial factors for any successful website. Gatsby’s optimization capabilities make it an excellent choice for creating lightning-fast and search engine-friendly applications. In this section, we’ll explore techniques for optimizing images, lazy loading, code splitting, and implementing SEO best practices with Gatsby. By the end of this chapter, you’ll have the knowledge to create high-performing websites that rank well in search engines.
Code Sample:
jsx // gatsby-config.js module.exports = { plugins: [ "gatsby-plugin-image", "gatsby-plugin-sharp", "gatsby-transformer-sharp", { resolve: "gatsby-plugin-manifest", options: { name: "My Gatsby Site", short_name: "Gatsby Site", start_url: "/", background_color: "#ffffff", theme_color: "#663399", display: "standalone", icon: "src/images/icon.png", }, }, "gatsby-plugin-react-helmet", "gatsby-plugin-sitemap", "gatsby-plugin-robots-txt", ], }
Adding Authentication and User Management
User authentication and management are essential for many web applications. In this section, we’ll explore how to integrate authentication providers like Auth0 or Firebase with Gatsby, allowing users to sign up, log in, and access protected content. By the end of this chapter, you’ll have the skills to add secure authentication and user management to your Gatsby projects.
Code Sample:
jsx // src/pages/dashboard.js import React from "react" import { navigate } from "gatsby" import { useAuth } from "../hooks/useAuth" const DashboardPage = () => { const { user, isAuthenticated, login } = useAuth() if (!isAuthenticated) { navigate("/login") return null } return ( <div> <h1>Welcome, {user.name}!</h1> <button onClick={login}>Log out</button> </div> ) } export default DashboardPage
Deploying Gatsby to Production
Once your Gatsby site is ready, it’s time to deploy it to a production environment. In this section, we’ll explore various deployment options, including static hosting platforms like Netlify and Vercel, as well as serverless deployments on AWS Lambda. By the end of this chapter, you’ll have a clear understanding of how to deploy your Gatsby projects and make them accessible to the world.
Advanced Techniques and Best Practices
To truly master Gatsby, it’s essential to explore advanced techniques and follow best practices. In this final section, we’ll dive into topics such as performance optimization, GraphQL schema customization, creating reusable components, and implementing internationalization (i18n). By leveraging these advanced techniques and adhering to best practices, you’ll be able to build scalable, maintainable, and robust Gatsby applications.
Conclusion
Congratulations! You have completed the comprehensive guide to mastering Gatsby. By now, you should have a solid understanding of the fundamental concepts, advanced techniques, and best practices for building powerful web applications with Gatsby. Remember to keep exploring, experimenting, and staying up to date with the latest updates in the Gatsby ecosystem. Happy coding!
Remember, mastering Gatsby is an ongoing journey, and practice is key. With each project, you’ll gain more confidence and expand your skill set8. Now go out there and create amazing websites with Gatsby!
Table of Contents
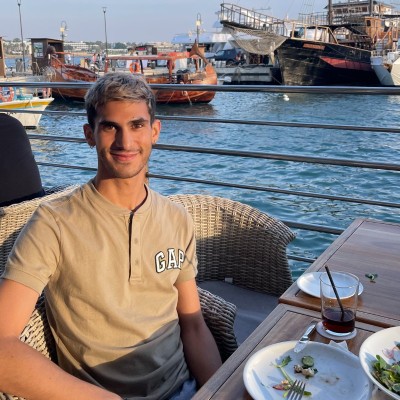
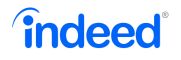