Scaling Gatsby: Strategies for Handling Large-Scale Projects
As web development projects grow in complexity and scale, managing the performance and build times becomes crucial. Gatsby, a popular React-based static site generator, offers an excellent solution for creating fast and efficient websites. However, as projects expand, developers might encounter challenges related to build times, data management, and overall performance. In this blog, we’ll explore essential strategies for scaling Gatsby and effectively handling large-scale projects. Let’s dive in!
1. Optimize Query Performance
Gatsby’s data layer is powered by GraphQL, providing an easy way to fetch data from various sources. However, fetching excessive data or using inefficient queries can lead to slow build times and decreased performance. To optimize query performance:
1.1 Use Static Queries
Static queries are resolved at build time, reducing the amount of data fetched during runtime. Use static queries for components that do not rely on dynamic data and can be generated during the build process. This approach minimizes the load on the client-side and significantly improves loading times.
jsx // Example of a static query in Gatsby import { graphql, useStaticQuery } from "gatsby" const MyComponent = () => { const data = useStaticQuery(graphql` query { allPosts { nodes { title date } } } `) // Use the data here... }
1.2 Limit Query Depth
Deeply nested GraphQL queries can lead to a large amount of data being fetched. Minimize the query depth and only request the required fields to optimize data retrieval. This reduces the time spent fetching unnecessary data and improves overall query performance.
1.3 Use gatsby-plugin-image
Instead of loading images directly, leverage Gatsby’s gatsby-plugin-image to optimize image loading. The plugin handles image transformations, lazy loading, and responsive image loading automatically, enhancing the overall website performance and user experience.
jsx // Example of using gatsby-plugin-image import { GatsbyImage, getImage } from "gatsby-plugin-image" const MyImageComponent = () => { const image = getImage(data.myImage) return <GatsbyImage image={image} alt="My Image" /> }
2. Implement Code Splitting
Large-scale projects often consist of numerous pages and components, leading to significant JavaScript bundle sizes. This can result in slower load times, especially for users on slower internet connections or less powerful devices. Implementing code splitting can help address this issue:
2.1 Use Dynamic Imports
Utilize dynamic imports for components or modules that are not required immediately on page load. This allows Gatsby to generate separate chunks for different sections of the website, which are loaded only when necessary.
jsx // Example of dynamic import const DynamicComponent = React.lazy(() => import("./DynamicComponent"))
2.2 Leverage @loadable/component
Gatsby offers support for @loadable/component, a library that simplifies code splitting. It allows you to load components asynchronously with minimal configuration, making it easier to manage code splitting across your project.
jsx // Example of using @loadable/component import loadable from "@loadable/component" const MyComponent = loadable(() => import("./MyComponent"))
3. Cache and Optimize Data
Caching and optimizing data can significantly improve the performance of large-scale Gatsby projects:
3.1 Implement Data Persistence
Gatsby’s data layer can benefit from data persistence techniques, like using Redis or other caching solutions. This ensures that expensive data queries are stored and reused, reducing the need for repeated expensive computations during build or runtime.
3.2 Use Gatsby’s Incremental Builds
Gatsby supports incremental builds, allowing you to update only the parts of the website affected by changes instead of regenerating the entire site. This feature drastically reduces build times, making it more manageable for large projects.
shell # Triggering an incremental build gatsby build --incremental
3.3 Optimize Asset Loading
Ensure that assets, such as JavaScript, CSS, and fonts, are efficiently loaded and cached by using cache-control headers and versioning techniques. This prevents unnecessary re-downloading of assets by the client and improves website performance.
4. Parallelize Build Tasks
Gatsby builds can be resource-intensive, especially for large projects. To speed up the build process and reduce the overall build time:
4.1 Use Worker Threads
Employing worker threads allows Gatsby to perform tasks concurrently, taking advantage of multi-core processors. This approach prevents bottlenecks and maximizes resource utilization during the build process.
javascript // Example of using worker threads in a Gatsby Node API const { Worker } = require("worker_threads") exports.onCreateWebpackConfig = ({ actions }) => { actions.setWebpackConfig({ plugins: [ new WorkerPlugin({ // Options... }), ], }) }
4.2 Enable Parallel Query Execution
Gatsby offers a feature called “parallel query execution,” which splits up data sourcing tasks across different cores, improving build performance. Enable this feature in the Gatsby configuration file:
javascript // gatsby-config.js module.exports = { flags: { PARALLEL_QUERY_RUNNING: true, }, }
5. Optimize Hosting and Deployment
Efficient hosting and deployment play a significant role in the overall performance of large-scale Gatsby projects:
5.1 Use a Content Delivery Network (CDN)
Leverage a CDN to distribute your website’s assets globally. CDNs cache and serve content from servers located closer to the user’s location, reducing latency and improving loading times.
5.2 Enable Compression
Enable Gzip or Brotli compression on your server to reduce the size of transferred data. Compressing assets before serving them to the client minimizes load times and improves the user experience.
5.3 Deploy to a High-Performance Server
Choose a high-performance hosting environment that can handle the demands of a large-scale Gatsby project. Consider using serverless hosting platforms or dedicated servers with sufficient resources to ensure optimal performance.
Conclusion
Scaling Gatsby for large-scale projects requires careful consideration of various aspects, from query optimization and code splitting to caching and deployment strategies. By following the strategies outlined in this blog, you can create blazing-fast, efficient, and highly performant websites that offer a seamless user experience. Embrace the power of Gatsby, and watch your project thrive even at scale!
Table of Contents
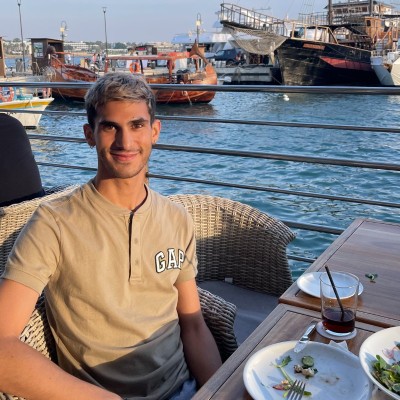
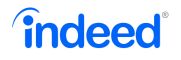