Building Lightning-Fast Websites with Gatsby
In today’s digital landscape, website performance is crucial for user satisfaction and search engine rankings. Slow-loading websites can lead to high bounce rates and diminished conversions. To address this issue, developers turn to static site generators like Gatsby, which combine the benefits of static websites with modern JavaScript frameworks. In this blog, we’ll explore how Gatsby can help you build lightning-fast websites, and we’ll provide code samples to guide you along the way.
1. What is Gatsby?
Gatsby is a popular open-source static site generator built on React.js. It combines the benefits of static websites, such as security and fast loading times, with the flexibility and interactivity of modern JavaScript frameworks. Gatsby leverages pre-rendering to generate static HTML, CSS, and JavaScript files that can be hosted on a Content Delivery Network (CDN) for fast delivery to end-users.
2. Benefits of Using Gatsby
- Blazing-fast loading times: Gatsby optimizes performance by generating static files that can be served directly from a CDN, ensuring lightning-fast loading times.
- Excellent SEO: With Gatsby, you can create SEO-friendly websites by leveraging server-side rendering and preloading techniques.
- Enhanced developer experience: Gatsby’s developer-friendly ecosystem provides numerous plugins, starters, and themes that make it easy to extend and customize your website.
- Seamless integration of data sources: Gatsby allows you to pull data from various sources, including CMS platforms, APIs, Markdown files, and databases, enabling dynamic content generation.
3. Getting Started with Gatsby
To get started with Gatsby, you need to have Node.js installed on your machine. Follow these steps:
- Install Gatsby globally by running npm install -g gatsby-cli.
- Create a new Gatsby project with gatsby new my-website.
- Change directory to your project folder with cd my-website.
- Start the development server using gatsby develop.
4. Optimizing Performance with Gatsby
Gatsby offers several features that help optimize website performance:
4.1 Code Splitting and Lazy Loading
Gatsby automatically code-splits your JavaScript bundles to ensure that only the necessary code is loaded for each page. Additionally, you can use dynamic imports to enable lazy loading for components that are not immediately visible. Here’s an example:
jsx import React, { lazy, Suspense } from 'react'; const LazyComponent = lazy(() => import('./LazyComponent')); const MyPage = () => ( <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> );
4.2 Image Optimization
Gatsby’s built-in image processing capabilities allow you to optimize images for various screen sizes and resolutions. The gatsby-image plugin is particularly useful for automatically generating responsive images. Here’s how you can use it:
jsx import { StaticImage } from 'gatsby-plugin-image'; const MyImage = () => ( <StaticImage src="../images/my-image.jpg" alt="My Image" placeholder="blurred" layout="fullWidth" /> );
4.3 Preloading and Prefetching
Gatsby enables you to optimize navigation by preloading or prefetching resources. Use the gatsby-plugin-preload-fonts and gatsby-plugin-preload-link-crossorigin plugins to preload fonts and external assets. You can also use the Link component to prefetch pages in advance:
jsx import { Link } from 'gatsby'; const MyLink = () => ( <Link to="/my-page" prefetch="true">Go to My Page</Link> );
5. Utilizing Gatsby Plugins
Gatsby’s plugin ecosystem provides a wealth of functionalities. Here are a few essential plugins that can enhance your website’s performance:
5.1 Gatsby Image Plugin
The ‘gatsby-plugin-image’ allows you to automatically optimize and load images with lazy loading and advanced image placeholders.
5.2 Gatsby Cache Plugin
The ‘gatsby-plugin-caching’ plugin helps cache your website’s assets, improving subsequent load times for returning visitors.
5.3 Gatsby Plugin Manifest
The ‘gatsby-plugin-manifest’ plugin generates a web app manifest file, enabling your website to function as a Progressive Web App (PWA).
6. Implementing Progressive Web Apps (PWAs) with Gatsby
By leveraging Gatsby’s PWA support and the ‘gatsby-plugin-offline’ plugin, you can transform your website into a fully functional Progressive Web App. PWAs offer offline capabilities, background sync, push notifications, and an app-like experience for users.
Conclusion
Gatsby is an excellent choice for developers aiming to build lightning-fast websites without sacrificing modern features. Its powerful ecosystem, optimization techniques, and support for PWAs make it a robust tool for delivering exceptional performance and user experiences. By following the guidelines and code samples provided in this blog, you’ll be well on your way to creating high-performing websites with Gatsby.
In summary, Gatsby’s static site generation, code splitting, image optimization, and plugin ecosystem enable developers to build websites that load quickly, rank well in search engines, and offer an exceptional user experience. Start leveraging Gatsby’s power today and revolutionize the performance of your web projects.
Table of Contents
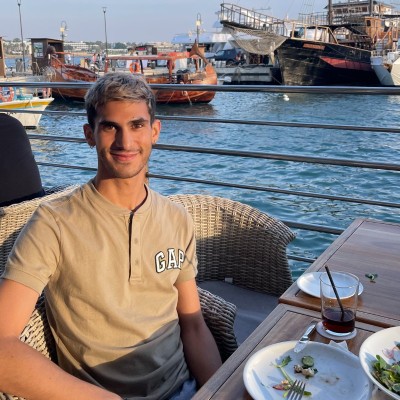
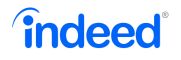