Supercharging Performance with Gatsby Image Optimization
In today’s fast-paced digital world, performance is key to the success of any website. Users expect fast load times and a smooth browsing experience, and if your site fails to meet those expectations, you risk losing valuable visitors and potential customers. One of the major factors that affect website performance is image optimization. Large, unoptimized images can slow down page load times, leading to higher bounce rates and lower search engine rankings.
Thankfully, with the advent of modern web development tools, we now have powerful solutions to tackle image optimization issues effectively. Gatsby, a popular React-based static site generator, offers a comprehensive and straightforward way to supercharge your website’s performance through its image optimization capabilities. In this blog post, we will explore the various techniques Gatsby provides to optimize images, ensuring blazing-fast load times and improved user experiences.
Why Image Optimization Matters
Before diving into Gatsby’s image optimization techniques, let’s briefly understand why image optimization is crucial for your website’s performance.
- Faster Load Times: Large image sizes can significantly increase page load times, especially on mobile devices or in regions with limited internet bandwidth. Optimizing images reduces their file sizes, leading to faster load times and a smoother user experience.
- SEO Benefits: Search engines consider page load speed as a ranking factor. By optimizing your images and improving page load times, you can positively influence your website’s search engine rankings, leading to higher organic traffic.
- Bandwidth Savings: Image optimization reduces the amount of data that needs to be transferred between the server and the user’s device. This helps save bandwidth costs, especially for websites with high traffic and large media content.
Gatsby Image Plugin: The Magic Wand
Gatsby’s Image plugin is the magic wand that enables seamless image optimization for your website. It automates various image-related tasks, such as responsive image generation, lazy loading, and quality optimization, while using cutting-edge techniques like the “blur-up” effect for smoother user experiences.
Setting Up Gatsby Image Plugin
To get started, make sure you have a Gatsby project set up. If you don’t have one yet, you can quickly create a new Gatsby project with the following commands:
bash npm install -g gatsby-cli gatsby new my-gatsby-project cd my-gatsby-project
Once you have a Gatsby project ready, installing and configuring the Gatsby Image plugin is a breeze. First, install the required dependencies:
bash npm install gatsby-plugin-image gatsby-plugin-sharp gatsby-transformer-sharp
Next, open your gatsby-config.js file and add the gatsby-plugin-image, gatsby-plugin-sharp, and gatsby-transformer-sharp plugins to the plugins array:
javascript // gatsby-config.js module.exports = { siteMetadata: { title: "My Gatsby Site", }, plugins: [ "gatsby-plugin-image", "gatsby-plugin-sharp", "gatsby-transformer-sharp", ], };
Using Gatsby Image in Your Project
With the Gatsby Image plugin set up, you can start optimizing images in your Gatsby project. The plugin provides a <GatsbyImage> component that you can use to render optimized images effortlessly.
To use the <GatsbyImage> component, import it from gatsby-plugin-image:
javascript import { GatsbyImage } from "gatsby-plugin-image";
Next, you’ll need to query for your images using GraphQL. Gatsby’s image processing relies on Sharp, a powerful image processing library. So, you must query for your images using the ImageSharp data type:
javascript import { graphql, useStaticQuery } from "gatsby"; const MyComponent = () => { const data = useStaticQuery(graphql` query { placeholderImage: file(relativePath: { eq: "my-image.jpg" }) { childImageSharp { gatsbyImageData(layout: FULL_WIDTH, quality: 90) } } } `); return ( <GatsbyImage image={data.placeholderImage.childImageSharp.gatsbyImageData} alt="My Optimized Image" /> ); };
In this example, we query for an image named “my-image.jpg” and apply the gatsbyImageData transformation to optimize the image. The layout property with the value FULL_WIDTH ensures the image will stretch to fill its container’s width.
Image Optimization Techniques
Now that you have the Gatsby Image plugin set up and have learned how to use it in your components, let’s explore some powerful image optimization techniques that Gatsby offers.
1. Responsive Images
In a mobile-first world, ensuring that your website looks great on various devices and screen sizes is essential. The Gatsby Image plugin makes it easy to generate responsive images that automatically adapt to different viewport sizes.
To create responsive images, use the gatsbyImageData transformation with different width and height options. This will generate multiple image sources with varying resolutions, and the browser will load the most appropriate one based on the user’s device.
javascript const data = useStaticQuery(graphql` query { responsiveImage: file(relativePath: { eq: "my-image.jpg" }) { childImageSharp { gatsbyImageData( width: 800 height: 400 layout: FULL_WIDTH quality: 90 formats: [AUTO, WEBP, AVIF] ) } } } `);
In this example, we are generating responsive images with a width of 800 pixels and a height of 400 pixels. The formats property includes the image formats we want to support: AUTO allows Gatsby to choose the best format for the browser (usually WebP or JPEG), and we also specify WEBP and AVIF for additional browser support.
2. Lazy Loading
Lazy loading is a technique that defers the loading of non-essential resources, such as images, until they are needed. With lazy loading, images below the fold or outside the viewport won’t be loaded until the user scrolls to them, saving precious bandwidth and speeding up initial page load times.
The Gatsby Image plugin automatically handles lazy loading for you, making it incredibly simple to implement. Just add the loading attribute to the <GatsbyImage> component:
javascript <GatsbyImage image={data.responsiveImage.childImageSharp.gatsbyImageData} alt="My Optimized Image" loading="lazy" />
With this single line of code, your images will be lazily loaded by the browser, providing a more efficient user experience.
3. Blur-Up Technique
The “blur-up” technique is an ingenious way to enhance user experience while loading images. Instead of displaying a blank space or a loading spinner, the technique shows a low-quality, heavily blurred version of the image first, which quickly loads due to its small size. As the higher-quality image loads, it gradually replaces the blurred version, creating a smooth and visually pleasing transition.
To implement the blur-up effect in Gatsby, set the placeholder attribute to “blurred” in the <GatsbyImage> component:
javascript <GatsbyImage image={data.responsiveImage.childImageSharp.gatsbyImageData} alt="My Optimized Image" placeholder="blurred" />
With this configuration, Gatsby will automatically generate the blurred version of the image and apply the blur-up effect during loading.
4. Quality Optimization
Balancing image quality and file size is crucial for an optimal user experience. Gatsby’s Image plugin allows you to control image quality easily using the quality parameter. The quality value can range from 0 to 100, with 100 being the highest quality.
javascript const data = useStaticQuery(graphql` query { optimizedImage: file(relativePath: { eq: "my-image.jpg" }) { childImageSharp { gatsbyImageData(layout: FULL_WIDTH, quality: 80) } } } `);
In this example, we set the quality parameter to 80, which strikes a good balance between image quality and file size. Experiment with different values to find the sweet spot for your images.
Conclusion
In this blog post, we’ve explored how Gatsby’s Image optimization techniques can significantly supercharge your website’s performance. By leveraging Gatsby’s powerful image processing capabilities, you can deliver faster load times, better SEO, and improved user experiences.
Remember, image optimization is not a one-time task; it requires continuous monitoring and refinement as your website grows and evolves. Regularly review your images, use appropriate formats, and fine-tune quality settings to ensure your website stays performant and delightful for your users.
Happy coding and optimizing!
Table of Contents
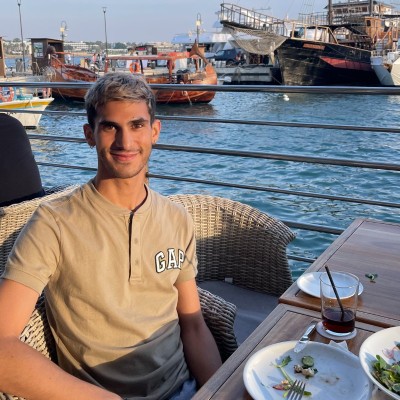
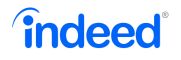