Securing Your Gatsby Site: Best Practices and Techniques
In today’s digital world, website security is of paramount importance. Cyber threats are constantly evolving, and developers must take proactive measures to safeguard their websites and the data they handle. Gatsby, a popular static site generator, offers numerous advantages in terms of performance, scalability, and user experience. However, like any web application, Gatsby sites are not exempt from security risks. In this blog, we will explore essential best practices and techniques to secure your Gatsby site effectively.
1. Keep Dependencies Updated
One of the fundamental steps to maintain a secure Gatsby site is to keep all dependencies up to date. This includes not only Gatsby itself but also the plugins and libraries used in the project. Developers regularly release updates to address security vulnerabilities and improve overall performance. Neglecting updates can lead to potential exploits, making your site susceptible to attacks.
To update Gatsby and its dependencies, open your project’s root directory and run the following commands:
bash # Update Gatsby npm install gatsby@latest # Update dependencies npm update
Make it a habit to check for updates regularly and automate the process whenever possible.
2. Secure Authentication and Authorization
Authentication and authorization are crucial aspects of web application security. Whether your Gatsby site has user accounts or not, implementing proper authentication measures is essential to protect sensitive data and prevent unauthorized access.
2.1 Use HTTPS
Ensure your Gatsby site is served over HTTPS. HTTPS encrypts the data transmitted between the user’s browser and the server, safeguarding it from eavesdropping and man-in-the-middle attacks. Most hosting providers offer free SSL/TLS certificates, making it easier than ever to enable HTTPS for your site.
To enable HTTPS on Gatsby, use the following snippet in your gatsby-config.js:
javascript module.exports = { // ... siteMetadata: { // ... }, plugins: [ // ... ], // Enable HTTPS for Gatsby site // Replace 'your-domain.com' with your actual domain // Set "process.env.NODE_ENV" to "production" when deploying to production flags: { PRESERVE_WEBPACK_CACHE: true, DEV_SSR: false, FAST_DEV: false, QUERY_ON_DEMAND: false, PARALLEL_SOURCING: false, FUNCTIONS: false, DEV_WEBPACK_CACHE: false, }, // ... };
2.2 Use Secure Authentication Methods
When implementing user authentication, avoid reinventing the wheel and opt for well-established authentication methods such as OAuth, OpenID Connect, or JWT (JSON Web Tokens). These methods have been thoroughly tested and are more secure than trying to create your own authentication system.
For instance, using the popular passport library in conjunction with OAuth for authentication:
javascript const passport = require('passport'); const { Strategy: OAuth2Strategy } = require('passport-oauth2'); passport.use( new OAuth2Strategy( { authorizationURL: 'https://provider.com/oauth2/authorize', tokenURL: 'https://provider.com/oauth2/token', clientID: 'YOUR_CLIENT_ID', clientSecret: 'YOUR_CLIENT_SECRET', callbackURL: '/auth/callback', }, (accessToken, refreshToken, profile, cb) => { // Save the user data to the database or use it for authorization return cb(null, profile); } ) );
2.3 Role-Based Access Control (RBAC)
If your Gatsby site has multiple user roles, implementing Role-Based Access Control (RBAC) is essential. RBAC restricts certain actions and access based on a user’s assigned role. This way, even if an attacker gains unauthorized access, they will have limited capabilities within the system.
javascript // Example RBAC middleware using Express.js const RBACMiddleware = (allowedRoles) => (req, res, next) => { if (allowedRoles.includes(req.user.role)) { return next(); } else { return res.status(403).json({ message: 'Forbidden' }); } }; // Usage of the middleware for specific routes const express = require('express'); const app = express(); app.get('/admin', RBACMiddleware(['admin']), (req, res) => { // Handle admin-specific tasks }); app.get('/user', RBACMiddleware(['user', 'admin']), (req, res) => { // Handle user-specific tasks });
3. Input Validation and Sanitization
User input is a common source of security vulnerabilities. Always validate and sanitize user inputs before processing them, as they may contain malicious code or attempts to exploit vulnerabilities.
3.1 Use Parameterized Queries
If your Gatsby site interacts with a database or third-party APIs, utilize parameterized queries or prepared statements to prevent SQL injection attacks.
javascript // Example parameterized query using SQLite3 in Node.js const sqlite3 = require('sqlite3').verbose(); const db = new sqlite3.Database('database.sqlite'); app.get('/products/:id', (req, res) => { const productId = req.params.id; db.get('SELECT * FROM products WHERE id = ?', [productId], (err, row) => { if (err) { return res.status(500).json({ error: 'Internal Server Error' }); } if (!row) { return res.status(404).json({ error: 'Product not found' }); } return res.json(row); }); });
3.2 Sanitize User-Generated Content
If your Gatsby site allows user-generated content, sanitize it before rendering to prevent cross-site scripting (XSS) attacks. Use libraries like dompurify to sanitize user input containing HTML markup.
javascript const createDOMPurify = require('dompurify'); const { JSDOM } = require('jsdom'); const window = new JSDOM('').window; const DOMPurify = createDOMPurify(window); const userInput = '<script>alert("XSS Attack!");</script>'; const sanitizedContent = DOMPurify.sanitize(userInput); console.log(sanitizedContent); // Output: ''
4. Implement Content Security Policy (CSP)
A Content Security Policy (CSP) is a security mechanism that helps mitigate the risk of cross-site scripting and other code injection attacks. By specifying which sources of content are allowed, CSP reduces the likelihood of unauthorized scripts executing on your Gatsby site.
To implement CSP, add the following meta tag to your site’s HTML template:
html <!-- Content Security Policy (CSP) meta tag --> <meta http-equiv="Content-Security-Policy" content="default-src 'self'; img-src https: data:; script-src 'self' 'unsafe-inline'; style-src 'self' 'unsafe-inline';">
The example policy above restricts all content to be loaded from the same origin (‘self’), allows images from https and data sources, and allows scripts and styles with ‘unsafe-inline’ attributes. Customize this policy according to your site’s specific requirements.
5. Regular Security Audits
Performing regular security audits is vital to identify and address potential vulnerabilities in your Gatsby site. Conduct code reviews, penetration testing, and vulnerability scanning to assess the security posture of your application.
5.1 Manual Code Reviews
Assign experienced developers to conduct thorough manual code reviews. Look for security flaws such as potential SQL injection points, improper input validation, insecure authentication methods, and the use of outdated or vulnerable libraries.
5.2 Penetration Testing
Hire ethical hackers or use automated tools to perform penetration testing on your Gatsby site. Penetration tests simulate real-world attacks to evaluate the system’s security under various scenarios. They can help uncover hidden vulnerabilities that might not be apparent during regular code reviews.
5.3 Vulnerability Scanning
Utilize automated vulnerability scanning tools to scan your Gatsby site and its dependencies for known security issues. These tools can quickly identify outdated libraries, insecure configurations, and other potential weaknesses.
Conclusion
Securing your Gatsby site is a continuous process that requires diligence and proactive measures. By keeping dependencies updated, implementing secure authentication and authorization, validating and sanitizing user input, using Content Security Policy, and conducting regular security audits, you can significantly reduce the risk of cyber threats and ensure a safe online experience for your users. Stay vigilant and always be on the lookout for emerging security best practices to keep your Gatsby site well-protected in an ever-changing digital landscape.
Table of Contents
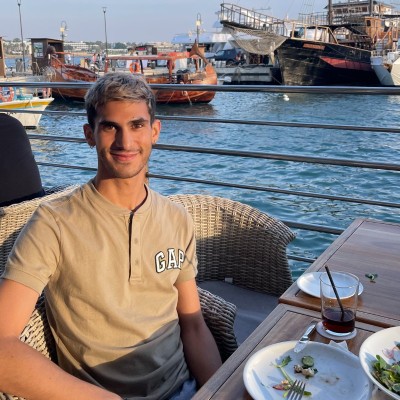
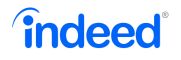