Gatsby and Serverless Functions: Integrating Backend Logic
In today’s web development landscape, building fast and dynamic websites is crucial for delivering a seamless user experience. Gatsby, a popular static site generator, has gained immense popularity due to its ability to create lightning-fast websites by leveraging React and GraphQL. However, there are times when you need to include backend logic for handling data processing, authentication, or other server-side functionalities. That’s where serverless functions come into play, allowing you to integrate backend logic into your Gatsby site without the need for complex server setups. In this tutorial, we’ll explore how to integrate serverless functions with Gatsby to create dynamic and personalized experiences for your users.
1. Prerequisites
Before we dive into the integration process, you should have a basic understanding of Gatsby, React, and GraphQL. Familiarity with serverless functions and JavaScript will also be beneficial. Make sure you have Node.js and npm (Node Package Manager) installed on your system.
2. Setting Up the Gatsby Project
If you already have a Gatsby project up and running, feel free to skip this step. Otherwise, let’s quickly set up a new Gatsby project:
1. First, install the Gatsby CLI by running the following command in your terminal:
bash npm install -g gatsby-cli
2. Create a new Gatsby project:
bash gatsby new my-gatsby-project cd my-gatsby-project
3. Start the development server:
bash gatsby develop
Now you should have your Gatsby project running locally at http://localhost:8000/. Let’s proceed to the next steps for integrating serverless functions.
3. Introducing Serverless Functions
Serverless functions, also known as serverless APIs or functions-as-a-service (FaaS), are individual units of backend logic that can be executed on demand without the need to manage server infrastructure. These functions are typically small, stateless, and short-lived, making them perfect for handling specific tasks.
3.1 Benefits of Serverless Functions
- Scalability: Serverless functions automatically scale based on demand, ensuring optimal performance during traffic spikes.
- Cost-Efficient: You pay only for the actual usage of the function, making it cost-efficient, especially for low-traffic websites.
- Simplified Backend Development: By using serverless functions, you can focus on writing code rather than managing servers.
- Easier Maintenance: Serverless platforms handle infrastructure updates and maintenance, reducing your operational overhead.
4. Serverless Function Providers
Several cloud providers offer serverless computing services. The two most popular ones are AWS Lambda and Netlify Functions. For this tutorial, we’ll use Netlify Functions due to its ease of use and seamless integration with Gatsby.
4.1 Setting Up Netlify Functions
If you don’t have a Netlify account, sign up for free at https://www.netlify.com and create a new site from your GitHub repository.
Once your site is set up, follow these steps to enable Netlify Functions:
1. In your Gatsby project, create a new folder called netlify at the root level:
bash mkdir netlify
2. Inside the netlify folder, create another folder named functions:
bash mkdir netlify/functions
3. Now, let’s install the Netlify CLI, which helps us deploy functions to Netlify:
bash npm install -g netlify-cli
4. Authenticate the Netlify CLI with your account:
bash netlify login
5. Link your Gatsby project to your Netlify site:
bash netlify init
6. Deploy your Gatsby site to Netlify:
bash gatsby build netlify deploy --prod
Your Gatsby site is now deployed on Netlify, and you’re ready to create serverless functions.
5. Creating a Simple Serverless Function
Let’s start by creating a basic serverless function that interacts with an external API. In this example, we’ll build a function that fetches random quotes using the They Said So Quotes API.
1. Create a new file named getQuote.js inside the netlify/functions directory.
javascript // netlify/functions/getQuote.js exports.handler = async (event, context) => { try { const response = await fetch('https://quotes.rest/qod.json'); const data = await response.json(); return { statusCode: 200, body: JSON.stringify(data.contents.quotes[0]), }; } catch (error) { return { statusCode: 500, body: JSON.stringify({ error: 'Failed to fetch the quote' }), }; } };
In this code, we use the fetch function to get a random quote from the They Said So Quotes API. The function returns the fetched quote along with a 200 status code in case of success or a 500 status code in case of an error.
2. Deploy the function to Netlify:
bash netlify deploy --prod
Now, your serverless function is live and accessible at https://your-netlify-site-url.netlify.app/.netlify/functions/getQuote. Replace your-netlify-site-url with your actual Netlify site URL.
6. Integrating the Serverless Function with Gatsby
Now that we have a serverless function up and running, let’s integrate it into our Gatsby site. We’ll create a simple React component that fetches the random quote and displays it on the homepage.
Create a new file named RandomQuote.js in the src/components folder:
jsx // src/components/RandomQuote.js import React, { useState, useEffect } from 'react'; const RandomQuote = () => { const [quote, setQuote] = useState(''); useEffect(() => { fetch('/.netlify/functions/getQuote') .then((response) => response.json()) .then((data) => setQuote(data.contents.quote)); }, []); return ( <div> <h2>Random Quote</h2> <blockquote>{quote}</blockquote> </div> ); }; export default RandomQuote;
In this code, we use React’s useState and useEffect hooks to fetch the quote from our serverless function when the component mounts. The fetched quote is then displayed inside a blockquote element.
Import and use the RandomQuote component in your index.js or any other page where you want to display the random quote:
jsx // src/pages/index.js import React from 'react'; import RandomQuote from '../components/RandomQuote'; const HomePage = () => { return ( <div> <h1>Welcome to My Gatsby Site!</h1> <RandomQuote /> </div> ); }; export default HomePage;
Now, when you visit your Gatsby site, you should see the homepage with a random quote fetched from the serverless function.
Conclusion
In this tutorial, we explored how to integrate serverless functions with Gatsby, allowing us to add backend logic to our static websites. We set up a Gatsby project, created a serverless function using Netlify Functions, and integrated it into a Gatsby page. This integration opens up a world of possibilities, enabling you to create dynamic and personalized experiences for your users without the need for complex server setups. With the power of Gatsby and serverless functions, you can build performant and scalable websites that delight your audience.
Remember, the examples provided here are just the tip of the iceberg. You can use serverless functions to handle forms, user authentication, database interactions, and much more. So, start experimenting and take your Gatsby projects to the next level with serverless functions! Happy coding!
Table of Contents
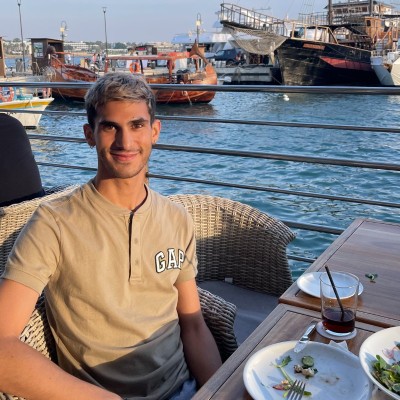
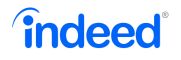