Designing Stunning User Interfaces with Gatsby and Styled Components
In the ever-evolving landscape of web development, creating visually stunning user interfaces has become a top priority. Users expect modern, sleek, and intuitive designs that not only captivate their attention but also enhance their overall experience. To achieve this, developers leverage the power of various tools and technologies. In this blog post, we’ll dive into how Gatsby, a popular React-based framework for building fast and efficient websites, combined with Styled Components, a powerful CSS-in-JS library, can help designers and developers create truly captivating user interfaces.
1. Understanding Gatsby and Styled Components:
Before we start exploring the potential synergy between Gatsby and Styled Components, let’s briefly introduce each of them.
- Gatsby: Gatsby is a blazing-fast static site generator built on top of React. It allows developers to create dynamic and content-rich websites while delivering an excellent performance. With its rich plugin ecosystem, Gatsby simplifies the process of integrating data sources and optimizing the site for SEO.
- Styled Components: Styled Components is a CSS-in-JS library that enables developers to write CSS within their JavaScript components. It provides a seamless way to manage styles, create reusable components, and implement dynamic styles based on component props.
2. Setting Up a Gatsby Project:
Before we dive into the UI design aspect, let’s set up a basic Gatsby project to get started. Ensure you have Node.js and npm installed, and then run the following commands:
bash npm install -g gatsby-cli gatsby new my-gatsby-site cd my-gatsby-site gatsby develop
3. Leveraging Styled Components:
Now that our Gatsby project is up and running, let’s integrate Styled Components to add some style and flair to our UI.
3.1 Basic Styling:
First, let’s create a simple styled component that renders a styled button:
jsx import React from 'react'; import styled from 'styled-components'; const StyledButton = styled.button` background-color: #007bff; color: #fff; font-size: 1rem; padding: 0.75rem 1.5rem; border: none; border-radius: 4px; cursor: pointer; &:hover { background-color: #0056b3; } `; const MyComponent = () => { return <StyledButton>Click Me</StyledButton>; }; export default MyComponent;
3.2 Theming with Styled Components:
Styled Components makes theming a breeze. Let’s set up a theme object and use it to create a themed button:
jsx import React from 'react'; import styled, { ThemeProvider } from 'styled-components'; const theme = { primaryColor: '#007bff', secondaryColor: '#0056b3', }; const StyledButton = styled.button` background-color: ${(props) => props.theme.primaryColor}; color: #fff; font-size: 1rem; padding: 0.75rem 1.5rem; border: none; border-radius: 4px; cursor: pointer; &:hover { background-color: ${(props) => props.theme.secondaryColor}; } `; const MyThemedComponent = () => { return ( <ThemeProvider theme={theme}> <StyledButton>Click Me</StyledButton> </ThemeProvider> ); }; export default MyThemedComponent;
4. Building a Stunning User Interface:
Now that we have a good understanding of how to use Styled Components within Gatsby, let’s focus on designing a visually appealing user interface.
4.1 Creating Responsive Layouts:
A key aspect of modern UI design is responsiveness. We want our website to adapt gracefully to different screen sizes. Gatsby and Styled Components make this process seamless.
jsx // components/Layout.js import React from 'react'; import styled from 'styled-components'; const Container = styled.div` max-width: 1200px; margin: 0 auto; padding: 0 1rem; `; const Header = styled.header` background-color: ${(props) => props.theme.primaryColor}; color: #fff; padding: 1rem; text-align: center; `; const Footer = styled.footer` background-color: ${(props) => props.theme.primaryColor}; color: #fff; padding: 1rem; text-align: center; `; const Layout = ({ children }) => { return ( <> <Header> <h1>My Stunning Gatsby Site</h1> </Header> <Container>{children}</Container> <Footer> <p>© 2023 My Gatsby Site. All rights reserved.</p> </Footer> </> ); }; export default Layout;
In the above example, we’ve created a simple layout component using Styled Components. The Container component sets a maximum width and centers the content. The Header and Footer components have a primary color from the theme, ensuring consistency across the UI. To use this layout in our pages, import it and wrap the page content as shown below.
jsx // pages/index.js import React from 'react'; import Layout from '../components/Layout'; const IndexPage = () => { return ( <Layout> <h2>Welcome to my stunning Gatsby site!</h2> <p>Here's some amazing content...</p> </Layout> ); }; export default IndexPage;
4.2 Animations and Transitions:
Adding subtle animations and transitions can greatly enhance the user experience. Styled Components allows us to apply animations easily.
jsx import React from 'react'; import styled, { keyframes } from 'styled-components'; const fadeIn = keyframes` from { opacity: 0; } to { opacity: 1; } `; const AnimatedText = styled.p` animation: ${fadeIn} 1s ease-in-out; `; const MyComponentWithAnimation = () => { return <AnimatedText>Hello, I'm animated!</AnimatedText>; }; export default MyComponentWithAnimation;
5. Optimizing for Performance:
As we continue to add more visual elements and interactivity to our UI, it’s crucial to keep performance in mind. Gatsby already provides several performance optimizations, such as code splitting and image optimization. Additionally, Styled Components generates unique class names for each styled component, resulting in efficient rendering.
To further optimize performance, consider lazy-loading large assets and minimizing the use of heavy animations. Always test the site’s performance using tools like Lighthouse or PageSpeed Insights.
Conclusion
In this blog post, we explored how Gatsby and Styled Components can be combined to design stunning user interfaces. We learned how to set up a Gatsby project and integrate Styled Components for powerful and flexible styling. Additionally, we discussed building responsive layouts, incorporating animations, and optimizing for performance.
With Gatsby and Styled Components at your disposal, you can create modern, visually appealing, and high-performing user interfaces that leave a lasting impression on your visitors. So, why wait? Start building your own stunning Gatsby site today!
Table of Contents
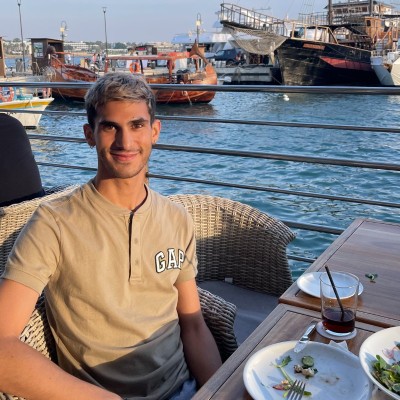
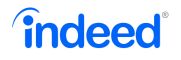