Advanced Concurrency Patterns in Go: Patterns Beyond Goroutines
Concurrency is a fundamental aspect of modern software development, enabling programs to efficiently execute multiple tasks simultaneously. Go (often referred to as Golang) has gained popularity for its simplicity and robust support for concurrency through Goroutines and channels. While Goroutines provide an excellent foundation for concurrent programming, there are more advanced patterns that can be employed to tackle complex concurrency scenarios, making your programs even more efficient, scalable, and resilient. In this article, we will dive into some of these advanced concurrency patterns that go beyond simple Goroutines.
1. Understanding Goroutines and Channels
Before diving into advanced concurrency patterns, let’s recap the basics of Goroutines and channels in Go. Goroutines are lightweight threads that enable concurrent execution of functions. Channels, on the other hand, are communication mechanisms that allow Goroutines to safely exchange data without race conditions. This foundation is essential for understanding the more complex patterns we will explore.
2. The Fan-Out, Fan-In Pattern
The Fan-Out, Fan-In pattern is particularly useful when dealing with I/O-bound tasks. It involves distributing tasks among multiple Goroutines (Fan-Out) and then collecting the results from those Goroutines (Fan-In). This approach maximizes parallelism and can significantly improve performance.
2.1. Implementing Fan-Out
go func worker(id int, tasks <-chan Task, results chan<- Result) { for task := range tasks { result := process(task) results <- result } } func fanOut(tasks []Task, workerCount int) <-chan Result { tasksChannel := make(chan Task, len(tasks)) resultsChannel := make(chan Result, len(tasks)) for i := 0; i < workerCount; i++ { go worker(i, tasksChannel, resultsChannel) } for _, task := range tasks { tasksChannel <- task } close(tasksChannel) return resultsChannel }
2.2. Implementing Fan-In
go func fanIn(resultsChannels []<-chan Result) <-chan Result { mergedResults := make(chan Result) var wg sync.WaitGroup for _, rc := range resultsChannels { wg.Add(1) go func(ch <-chan Result) { for result := range ch { mergedResults <- result } wg.Done() }(rc) } go func() { wg.Wait() close(mergedResults) }() return mergedResults }
2.3. Combining Fan-Out and Fan-In
go func main() { tasks := generateTasks() workerCount := 4 resultsChannels := make([]<-chan Result, workerCount) for i := 0; i < workerCount; i++ { resultsChannels[i] = fanOut(tasks[i*len(tasks)/workerCount:(i+1)*len(tasks)/workerCount], 2) } mergedResults := fanIn(resultsChannels) for result := range mergedResults { processResult(result) } }
The Fan-Out, Fan-In pattern can greatly enhance the efficiency of your program when dealing with concurrent I/O-bound operations, such as fetching data from multiple sources.
3. The Worker Pool Pattern
When facing CPU-bound tasks, creating a worker pool can help distribute the workload across a fixed number of Goroutines. This pattern prevents excessive resource consumption while maintaining a high level of parallelism.
3.1. Creating the Worker Pool
go type WorkerPool struct { workerCount int tasks chan Task results chan Result } func NewWorkerPool(workerCount, buffer int) *WorkerPool { return &WorkerPool{ workerCount: workerCount, tasks: make(chan Task, buffer), results: make(chan Result, buffer), } } func (wp *WorkerPool) Start() { for i := 0; i < wp.workerCount; i++ { go func(id int) { for task := range wp.tasks { result := process(task) wp.results <- result } }(i) } }
3.2. Dispatching Tasks
go func main() { tasks := generateTasks() workerCount := 4 buffer := 10 wp := NewWorkerPool(workerCount, buffer) wp.Start() for _, task := range tasks { wp.tasks <- task } close(wp.tasks) for result := range wp.results { processResult(result) } }
3.3. Managing Results
The Worker Pool pattern provides an organized way to manage and distribute CPU-bound tasks across a limited set of Goroutines, avoiding oversaturation of system resources.
4. The Context Pattern
Managing Goroutines across multiple stages of execution can become complex. The Context pattern helps with graceful Goroutine termination and propagation of deadlines and cancellations.
4.1. Introduction to Context
The context package provides a powerful way to pass deadlines, cancellations, and other values across Goroutines. It’s crucial for orchestrating concurrent processes and ensuring they are properly terminated when necessary.
4.2. Passing Context to Goroutines
go func workerWithContext(ctx context.Context, id int, tasks <-chan Task, results chan<- Result) { for { select { case <-ctx.Done(): return // Terminate the Goroutine on context cancellation case task, ok := <-tasks: if !ok { return } result := process(task) results <- result } } }
4.3. Handling Context Cancellation
go func main() { tasks := generateTasks() workerCount := 4 ctx, cancel := context.WithCancel(context.Background()) defer cancel() tasksChannel := make(chan Task, len(tasks)) resultsChannel := make(chan Result, len(tasks)) for i := 0; i < workerCount; i++ { go workerWithContext(ctx, i, tasksChannel, resultsChannel) } for _, task := range tasks { tasksChannel <- task } close(tasksChannel) for result := range resultsChannel { processResult(result) } }
The Context pattern ensures that Goroutines are aware of external events and can be gracefully terminated when their execution is no longer needed.
5. The Select Statement with Timeout
The select statement is a powerful construct for handling multiple channels concurrently. Combining select with a timeout can prevent Goroutines from blocking indefinitely.
5.1. Using Select for Multiple Channels
go func main() { taskChannel := make(chan Task) timeout := time.After(5 * time.Second) select { case task := <-taskChannel: processTask(task) case <-timeout: log.Println("Timed out while waiting for task") } }
5.2. Adding Timeout to Select
go func main() { taskChannel := make(chan Task) timeout := time.After(5 * time.Second) select { case task := <-taskChannel: processTask(task) case <-timeout: log.Println("Timed out while waiting for task") } }
The Select Statement with Timeout pattern ensures that Goroutines are not stuck indefinitely waiting for data from channels.
6. The Pub-Sub Pattern
The Publish-Subscribe (Pub-Sub) pattern is useful when you need to broadcast data to multiple subscribers. It facilitates loose coupling between components and can be valuable for scenarios like event-driven architectures.
6.1. Publisher Implementation
go type Publisher struct { subscribers map[string][]chan<- Event mu sync.RWMutex } func NewPublisher() *Publisher { return &Publisher{ subscribers: make(map[string][]chan<- Event), } } func (p *Publisher) Subscribe(eventType string, ch chan<- Event) { p.mu.Lock() defer p.mu.Unlock() p.subscribers[eventType] = append(p.subscribers[eventType], ch) } func (p *Publisher) Publish(event Event) { p.mu.RLock() defer p.mu.RUnlock() subscribers := p.subscribers[event.Type] for _, ch := range subscribers { go func(ch chan<- Event) { ch <- event }(ch) } }
6.2. Subscriber Implementation
go func main() { publisher := NewPublisher() eventType := "user_registered" userRegisteredCh := make(chan Event) publisher.Subscribe(eventType, userRegisteredCh) go func() { for event := range userRegisteredCh { processUserRegisteredEvent(event) } }() // Simulate a user registration event user := createUser("John Doe") event := Event{Type: eventType, Payload: user} publisher.Publish(event) }
6.3. Dynamic Subscriptions
The Pub-Sub pattern enables dynamic subscription and notification of events, promoting modularity and scalability.
7. Building Resilient Systems with Circuit Breaker Pattern
The Circuit Breaker pattern is crucial for building robust systems that can gracefully handle failures and prevent cascading failures in distributed environments.
7.1. Circuit Breaker Design and Implementation
go type CircuitBreaker struct { threshold int consecutiveFailures int state State mu sync.Mutex } func NewCircuitBreaker(threshold int) *CircuitBreaker { return &CircuitBreaker{ threshold: threshold, state: ClosedState{}, } } func (cb *CircuitBreaker) Execute(protectedFunc func() error) error { cb.mu.Lock() defer cb.mu.Unlock() if cb.state.ShouldAllowRequest() { err := protectedFunc() if err != nil { cb.consecutiveFailures++ if cb.consecutiveFailures >= cb.threshold { cb.state = OpenState{} } } else { cb.consecutiveFailures = 0 } return err } return fmt.Errorf("circuit breaker is open") }
7.2. Integration with Concurrency
go func main() { circuitBreaker := NewCircuitBreaker(3) var wg sync.WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go func() { defer wg.Done() err := circuitBreaker.Execute(func() error { return performNetworkRequest() }) if err != nil { log.Println("Error:", err) } }() } wg.Wait() }
7.3. Graceful Degradation and Recovery
The Circuit Breaker pattern helps systems gracefully degrade their functionality under duress and recover once the underlying issues are resolved.
Conclusion
Go’s concurrency primitives, such as Goroutines and channels, provide a solid foundation for building concurrent programs. However, when dealing with more complex concurrency scenarios, these advanced patterns can help you achieve even better performance, scalability, and robustness. Whether it’s efficiently distributing tasks, managing Goroutines with context, preventing deadlock with timeouts, building event-driven architectures, or ensuring system resilience with circuit breakers, these patterns provide a valuable toolkit for any Go developer. By mastering these patterns, you can create high-performance, reliable, and scalable concurrent applications that excel in today’s demanding software landscape.
Table of Contents
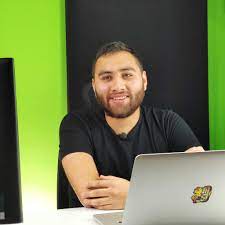
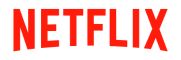