Advanced Error Handling in Go: Dealing with Panics and Recovering
Error handling is a critical aspect of any programming language, and Go (often referred to as Golang) is no exception. Go’s simplicity and concurrency support have made it a popular choice for building scalable and robust applications. However, to harness the full power of Go, you need to understand and implement advanced error handling techniques, especially when dealing with panics and recoveries.
In this comprehensive guide, we’ll explore advanced error handling in Go, with a focus on panics and recoveries. We’ll cover the basics, dive deep into the intricacies, and provide you with practical examples to master this essential aspect of Go programming.
1. Understanding Errors in Go
Before we delve into advanced error handling techniques, let’s start with the basics of error handling in Go. In Go, errors are represented by the error interface, which is a built-in interface with the following signature:
go type error interface { Error() string }
Any type that implements this interface becomes an error type, and you can use it to signal and handle errors throughout your code.
1.1. Basic Error Handling with if Statements
In Go, error handling often involves using if statements to check for errors. Here’s a simple example:
go package main import ( "errors" "fmt" ) func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Result:", result) }
In this example, we define a divide function that takes two floating-point numbers and returns their division result along with an error. If the second argument is zero, we return an error with a message. In the main function, we check for errors using an if statement and handle them accordingly.
1.2. Advanced Error Handling with defer and panic
While basic error handling with if statements is essential, Go provides more advanced mechanisms to handle errors, such as defer and panic. These mechanisms are particularly useful when dealing with exceptional situations, like unrecoverable errors.
2. Dealing with Panics in Go
In Go, a panic is a run-time error that typically results from a programmer error, such as dividing by zero or accessing an invalid array index. When a panic occurs, it unwinds the stack, running any deferred functions (functions scheduled to be called later with defer) along the way, and terminates the program. However, Go provides a way to recover from panics using the recover function.
2.1. The panic Function
You can use the panic function to trigger a panic explicitly. Here’s an example:
go package main import "fmt" func main() { fmt.Println("Start of the program") panic("Something went wrong!") fmt.Println("End of the program") }
In this example, the program will panic with the message “Something went wrong!” and terminate without printing “End of the program.” Panics are not meant to be used for normal error handling but are useful for handling exceptional situations where continuing the program’s execution is not possible.
2.2. Recovering from Panics with recover
To recover from a panic and prevent the program from terminating, you can use the recover function in combination with the defer statement. Here’s an example:
go package main import "fmt" func main() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered:", r) } }() fmt.Println("Start of the program") panic("Something went wrong!") fmt.Println("End of the program") }
In this example, we use defer to schedule an anonymous function to run when the surrounding function (main in this case) exits. Inside the anonymous function, we call recover to capture the panic value and print it. This allows the program to continue executing after the panic.
2.3. Best Practices for Using panic and recover
While panic and recover provide a way to handle exceptional situations, they should be used sparingly and only when necessary. Here are some best practices for using them:
- Avoid Using panic for Expected Errors: Reserve panic for truly exceptional situations, such as unrecoverable errors. For expected errors, return error values using the error interface.
- Use defer with recover: When using recover, always pair it with defer to ensure that it captures panics and allows the program to recover gracefully.
- Keep Panic Messages Informative: When calling panic, provide informative error messages that can help diagnose the issue.
- Document Panic Behavior: If your code relies on panics and recoveries for error handling, document this behavior in comments or documentation to make it clear to other developers.
Example: Recovering from a File Read Panic
Let’s look at a practical example of using panic and recover to handle a file read panic:
go package main import ( "fmt" "os" ) func readConfigFile() { file, err := os.Open("config.txt") if err != nil { panic(err) // Panic if unable to open the file } defer file.Close() // Read and process the file here fmt.Println("File opened and processed successfully") } func main() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered:", r) } }() fmt.Println("Start of the program") readConfigFile() fmt.Println("End of the program") }
In this example, the readConfigFile function attempts to open a configuration file. If it encounters an error while opening the file, it triggers a panic. The main function uses recover to capture the panic and continue execution, allowing it to print “End of the program.”
3. Advanced Error Handling Techniques
While panic and recover can handle exceptional situations, they are not a substitute for traditional error handling using the error interface. Here are some advanced error handling techniques to consider:
3.1. Custom Error Types
Go allows you to create custom error types by implementing the error interface. This can help you provide more context-specific information about errors in your code.
go package main import "fmt" type MyError struct { Code int Message string } func (e *MyError) Error() string { return fmt.Sprintf("Error %d: %s", e.Code, e.Message) } func foo() error { return &MyError{Code: 404, Message: "Resource not found"} } func main() { err := foo() if err != nil { fmt.Println("Error:", err) } }
In this example, we define a custom error type MyError that includes both a code and a message. The Error method is implemented to satisfy the error interface.
3.2. Error Wrapping
Error wrapping allows you to add context to an existing error by creating a new error that wraps the original one. This is especially useful when you need to provide more information about where an error occurred.
go package main import ( "fmt" "github.com/pkg/errors" ) func foo() error { return errors.Wrap(fmt.Errorf("something went wrong"), "foo failed") } func main() { err := foo() if err != nil { fmt.Println("Error:", err) // Output: Error: foo failed: something went wrong } }
In this example, the errors.Wrap function is used to wrap an existing error with additional context. This helps in tracing the error back to its source.
3.3. Error Chains
In complex applications, errors can propagate through multiple function calls. To maintain the context of errors and their relationships, you can use error chains. The github.com/pkg/errors package provides convenient functions for handling error chains.
go package main import ( "fmt" "github.com/pkg/errors" ) func foo() error { return errors.New("foo error") } func bar() error { if err := foo(); err != nil { return errors.Wrap(err, "bar error") } return nil } func main() { err := bar() if err != nil { fmt.Printf("Error: %+v\n", err) } }
In this example, the errors.Wrap function is used to create an error chain that reflects the relationship between the errors returned by the foo and bar functions.
Conclusion
Effective error handling is crucial for writing reliable and maintainable Go code. By understanding how to deal with panics and recoveries, creating custom error types, implementing error wrapping, and managing error chains, you can take your error handling skills to the next level.
Remember that while panic and recover have their place in Go, they should be used sparingly and only for exceptional situations. For expected errors, stick to the traditional error handling approach using the error interface.
With these advanced error handling techniques at your disposal, you’ll be better equipped to write robust and resilient Go applications that gracefully handle errors and provide meaningful information for debugging and troubleshooting.
Table of Contents
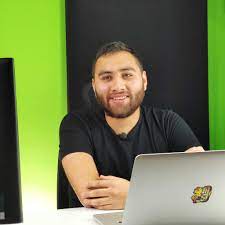
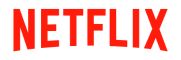