Does Go support anonymous functions (closures)?
Yes, Go supports anonymous functions, also known as closures. An anonymous function is a function defined without a name and can be declared and invoked inline within other function bodies, assignments, or function calls.
Anonymous functions in Go are often used for short-lived or single-use functionality, such as callbacks, event handlers, or deferred execution. They provide a convenient way to encapsulate logic within a specific context without the need to define a separate named function.
Here’s an example of defining and using an anonymous function in Go:
go func main() { add := func(x, y int) int { return x + y } sum := add(3, 5) fmt.Println(sum) // Output: 8 }
In this example, we define an anonymous function add that takes two int parameters and returns their sum. The anonymous function is assigned to a variable add and invoked with arguments 3 and 5 to calculate the sum, which is then printed to the console.
Anonymous functions in Go can capture and access variables from their surrounding lexical scope, making them powerful and flexible constructs for implementing closures and capturing state within a function’s context.
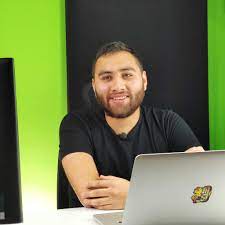
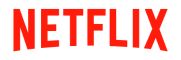