Introduction to Go’s Channels: Synchronization and Communication
Concurrency is a fundamental aspect of modern software development. In a world where multicore processors are the norm, it’s crucial to harness the power of parallelism to build efficient and responsive applications. Go, a statically typed and compiled language developed by Google, provides robust support for concurrent programming through its goroutines and channels.
In this blog post, we’ll delve into one of Go’s most distinctive features: channels. Channels are a mechanism for synchronization and communication between goroutines. They enable safe data sharing and coordination among concurrent processes, making it easier to write efficient, concurrent Go programs. Whether you’re a seasoned Go developer or just getting started with the language, understanding channels is essential for building scalable and responsive applications.
1. Why Concurrency Matters
Before we dive into the specifics of Go’s channels, let’s briefly discuss why concurrency is essential in modern software development.
- Utilizing Multicore Processors: Most modern computers come equipped with multicore processors, which can execute multiple tasks simultaneously. To fully leverage these capabilities, software must be designed to run concurrent operations efficiently.
- Responsiveness: Concurrency allows programs to remain responsive, even when handling heavy workloads. It ensures that tasks like user interface updates or network requests can continue to function smoothly, even if other parts of the program are busy.
- Parallelism: Concurrency provides a foundation for parallelism, where multiple tasks are executed simultaneously. This can lead to significant performance improvements for CPU-bound tasks.
- Efficient Resource Utilization: Concurrent programs can efficiently utilize system resources. They can avoid wasting CPU cycles by waiting for I/O operations to complete, making them more efficient and responsive.
Go’s concurrency model, based on goroutines and channels, simplifies the development of concurrent software while maintaining safety and efficiency.
2. Understanding Goroutines
Before we jump into channels, let’s briefly explore goroutines. Goroutines are lightweight, user-level threads in Go. They allow you to write concurrent code that’s easy to manage and doesn’t require low-level threading operations. Creating a goroutine is as simple as prefixing a function call with the go keyword:
go func main() { go doWork() // Start a new goroutine // ... } func doWork() { // Perform some work concurrently }
Goroutines are inexpensive to create and have minimal overhead, making it practical to use them for many concurrent tasks within a single program.
3. The Need for Communication
Concurrency often involves multiple goroutines working together to accomplish a task. In this collaborative environment, these goroutines need a way to communicate, share data, and coordinate their actions. This is where channels come into play.
3.1. What Are Channels?
Channels are the pipes that connect concurrent goroutines, allowing them to send and receive data. They provide a safe and synchronized means of communication and coordination. In Go, channels are first-class citizens, just like functions and data types, and are integral to building concurrent programs.
3.1.2. Channel Declaration
To declare a channel, you use the make function with the chan keyword, followed by the type of data the channel will carry. For example:
go ch := make(chan int) // Creates an integer channel
Here, we’ve created an int channel named ch. This channel can be used to send and receive integer values between goroutines.
3.2. Sending and Receiving on Channels
Channels support two fundamental operations: sending and receiving. The <- operator is used for both operations. Let’s see how they work:
3.2.1. Sending on a Channel
To send a value on a channel, you use the <- operator with the channel on the left side and the value on the right side. For example:
go ch <- 42 // Send the integer 42 on the channel ch
3.2.2. Receiving from a Channel
To receive a value from a channel, you also use the <- operator, but this time with the channel on the right side and the variable on the left side. For example:
go result := <-ch // Receive an integer from the channel ch and store it in the variable result
It’s important to note that sending and receiving on a channel block until there is another goroutine ready to perform the complementary operation. This blocking behavior enables synchronization and prevents race conditions.
3.3. Unbuffered vs. Buffered Channels
Channels in Go can be categorized into two types: unbuffered and buffered.
- Unbuffered Channels: These channels have no capacity to hold values. When a value is sent on an unbuffered channel, it must be received immediately by another goroutine. If there’s no receiver ready, the sender will block until one is available. This tight coupling ensures safe data exchange and synchronization between goroutines.
- Buffered Channels: Buffered channels, on the other hand, have a specified capacity. They allow multiple values to be sent on the channel before a receiver is ready. Sending on a buffered channel only blocks when the channel is full, and receiving only blocks when the channel is empty. Buffered channels are useful for scenarios where temporary bursts of data need to be exchanged without immediate synchronization.
Here’s an example of creating and using a buffered channel:
go ch := make(chan int, 3) // Create a buffered channel with a capacity of 3 ch <- 1 ch <- 2 ch <- 3 // These sends do not block
In this example, the channel won’t block until the fourth value is sent.
4. Synchronization with Channels
One of the primary use cases for channels is synchronization between goroutines. Channels act as a synchronization barrier, ensuring that certain actions only occur when both the sender and receiver are ready. This synchronization is crucial for coordinating the execution of concurrent code.
4.1. Wait for Goroutines to Finish
A common use case for channels is waiting for a group of goroutines to finish their work before proceeding. You can achieve this by creating a channel and having each goroutine signal its completion by sending a value on the channel. The main function can then wait for these signals by receiving the same number of values from the channel.
Here’s an example that demonstrates waiting for multiple goroutines to finish:
go func main() { numGoroutines := 3 done := make(chan bool, numGoroutines) for i := 0; i < numGoroutines; i++ { go doWork(i, done) } // Wait for all goroutines to finish for i := 0; i < numGoroutines; i++ { <-done } fmt.Println("All goroutines have finished.") } func doWork(id int, done chan bool) { // Simulate some work time.Sleep(time.Second * time.Duration(rand.Intn(3))) fmt.Printf("Goroutine %d has finished.\n", id) done <- true // Signal that this goroutine has finished }
In this example, done is a buffered channel used to signal the completion of each goroutine. The main function waits for all goroutines to finish by receiving a value from the done channel for each goroutine.
4.2. Coordinating Data Flow
Channels not only enable synchronization but also facilitate data flow between goroutines. They ensure that data is sent and received in a coordinated manner, preventing race conditions and data corruption.
Consider a scenario where you have two goroutines: one producing data, and another consuming it. Channels can be used to safely pass data between them, ensuring that the consumer waits for data to be available before proceeding.
Here’s a simple example that demonstrates this data flow:
go func main() { dataChannel := make(chan int) go produceData(dataChannel) go consumeData(dataChannel) // Wait for a few seconds to allow goroutines to finish time.Sleep(time.Second * 3) } func produceData(ch chan int) { for i := 1; i <= 5; i++ { fmt.Printf("Producing data: %d\n", i) ch <- i time.Sleep(time.Second) } close(ch) // Close the channel to signal that no more data will be sent } func consumeData(ch chan int) { for { data, ok := <-ch if !ok { fmt.Println("No more data to consume.") break } fmt.Printf("Consuming data: %d\n", data) } }
In this example, the produceData goroutine sends data to the consumeData goroutine through the dataChannel. The consumer waits for data to be available and safely processes it.
5. Select Statement for Channel Operations
The select statement in Go provides a way to choose between multiple channel operations. It’s a powerful tool for building concurrent programs that need to respond to multiple communication channels. The select statement allows you to wait on multiple channels and perform different actions depending on which channel is ready.
Here’s an example that demonstrates the use of select:
go func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(time.Second) ch1 <- "Hello from channel 1" }() go func() { time.Sleep(time.Second * 2) ch2 <- "Hello from channel 2" }() select { case msg1 := <-ch1: fmt.Println(msg1) case msg2 := <-ch2: fmt.Println(msg2) } }
In this example, we have two goroutines sending messages on different channels. The select statement waits for the first channel that becomes ready and then proceeds to execute the corresponding case.
6. Closing Channels
Closing a channel is a way to signal that no more values will be sent on it. It’s an important concept when working with channels because it allows receivers to know when they should stop waiting for additional data.
To close a channel, you use the close function:
go ch := make(chan int) close(ch) // Close the channel
After closing a channel, any subsequent send operation on the channel will panic, and any receive operation will return a zero value immediately.
Here’s an example that demonstrates closing a channel:
go func main() { ch := make(chan int) go func() { defer close(ch) for i := 1; i <= 5; i++ { ch <- i } }() for data := range ch { fmt.Printf("Received data: %d\n", data) } }
In this example, the producer goroutine closes the channel after sending all the data. The consumer uses a for range loop to receive data until the channel is closed.
Conclusion
Go’s channels are a powerful tool for synchronization and communication in concurrent programs. They provide a safe and efficient means of coordinating goroutines, allowing you to build responsive and scalable software. In this blog post, we’ve covered the basics of channels, including their declaration, sending and receiving operations, and the use of unbuffered and buffered channels. We’ve also explored how channels can be used for synchronization, data flow, and handling multiple communication channels with the select statement.
As you delve deeper into Go’s concurrency model, channels will become an indispensable part of your toolkit. They enable you to harness the full potential of goroutines and build highly concurrent and responsive applications. So, embrace the power of Go’s channels and start building concurrent software with confidence.
Table of Contents
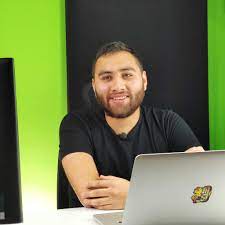
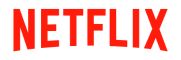