Creating Robust Command-Line Tools: A Comprehensive Guide Using Go
Go, often referred to as Golang, is a powerful language known for its simplicity and performance. Developed by Google engineers, it offers a great standard library that facilitates building robust and efficient software. This makes it an ideal language if you’re looking to hire Golang developers for your project. One area where Go truly excels is in the creation of command-line tools. In this post, we’ll explore how to build a command-line tool using Go, through practical examples and explanations. These insights will also help you understand the skills to look for when you hire Golang developers.
Getting Started with Go
Before we dive into building command-line tools, it’s important to understand Go’s basic principles. Go has a strong focus on simplicity, readability, and efficiency. It’s statically typed and compiled, which allows for strong performance and robustness. These traits make Go a top choice for various applications, and as a result, many companies are choosing to hire Golang developers to leverage these benefits. Understanding these principles will not only help you in building effective command-line tools, but also in making informed decisions when you plan to hire Golang developers.
You will need Go installed on your machine to follow this guide. If you haven’t installed it yet, follow the instructions on the [official Go website] (https://golang.org/doc/install).
The Basics of Building a Command-Line Tool
A command-line tool typically reads input from the user, performs some operation, and returns an output. A simple “Hello, World!” command-line tool can be written in Go as follows:
```go package main import ( "fmt" ) func main() { fmt.Println("Hello, World!") } ```
Save this as `hello.go` and run it using the command `go run hello.go`. You will see “Hello, World!” printed to your terminal.
Parsing Command-Line Arguments
Most command-line tools take arguments that alter their behavior. In Go, these arguments are accessible through the `os.Args` slice. The first element (`os.Args[0]`) is the program name. Any subsequent elements are the arguments passed in. Here’s how we can enhance our “Hello, World!” tool to greet a user-specified name:
```go package main import ( "fmt" "os" ) func main() { if len(os.Args) < 2 { fmt.Println("No name provided.") os.Exit(1) } fmt.Printf("Hello, %s!\n", os.Args[1]) } ```
This program checks whether a name was provided as an argument. If not, it prints an error message and exits with status 1 (indicating an error). If a name was provided, it uses that name in the greeting.
While `os.Args` is sufficient for simple programs, for more complex tools, we might want to use a library like `flag` or `cobra` that offers advanced parsing capabilities.
Building a More Complex Command-Line Tool
Let’s now create a more complex command-line tool – a basic task manager. This tool will allow users to add tasks, list tasks, and mark tasks as completed.
1. Defining the Task Structure
We start by defining a `Task` type to hold our task data:
```go package main type Task struct { ID int Name string Completed bool } ```
Each task has an ID, a Name, and a boolean indicating whether it’s been completed.
2. Setting Up Task Operations
Next, we define operations on tasks. For simplicity, we’ll use a slice to hold tasks and assign each task a unique ID based on its position in the slice:
```go var tasks []Task func addTask(name string) int { id := len(tasks) + 1 task := Task{id, name, false} tasks = append(tasks, task) return id } func listTasks() { for _, task := range tasks { fmt.Printf("ID: %d, Name: %s, Completed: %t\n", task.ID, task.Name, task.Completed) } } func completeTask(id int) { for i, task := range tasks { if task.ID == id { tasks[i].Completed = true return } } fmt.Println("Task not found.") } ```
3. Parsing Command-Line Arguments
We’ll use the `flag` package to handle argument parsing. This allows us to define flags that the user can use to interact with the task manager:
```go func main() { addCommand := flag.NewFlagSet("add", flag.ExitOnError) addName := addCommand.String("name", "", "Name of the task") listCommand := flag.NewFlagSet("list", flag.ExitOnError) completeCommand := flag.NewFlagSet("complete", flag.ExitOnError) completeID := completeCommand.Int("id", 0, "ID of the task to mark as complete") if len(os.Args) < 2 { fmt.Println("expected 'add', 'list', or 'complete' subcommands") os.Exit(1) } switch os.Args[1] { case "add": addCommand.Parse(os.Args[2:]) addTask(*addName) case "list": listCommand.Parse(os.Args[2:]) listTasks() case "complete": completeCommand.Parse(os.Args[2:]) completeTask(*completeID) default: flag.PrintDefaults() os.Exit(1) } } ```
In the main function, we set up flag sets for each of our commands and parse the command-line arguments accordingly.
Conclusion
As we’ve seen, Go’s simplicity and powerful standard library make it an excellent language for building command-line tools. The `os` and `flag` packages provide all the capabilities we need to handle input and control program behavior. With these tools in hand, you could build anything from simple scripts to powerful CLI applications, or even hire Golang developers to extend your project further. This flexibility and power truly highlight the strengths of using Go in your development process. Happy coding!
Table of Contents
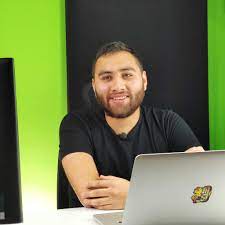
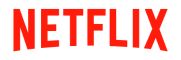