Working with Dates and Times in Go: Handling Timezones and Formatting
Time is a fundamental aspect of programming, and when it comes to building applications, handling dates and times accurately is crucial. In Go, the standard library provides powerful tools for working with dates and times, making it relatively straightforward to manipulate, format, and manage time-related data. In this blog post, we will explore how to work with dates and times in Go, focusing on two essential aspects: handling timezones and formatting.
1. Understanding Time in Go
Before diving into handling timezones and formatting, it’s essential to understand how Go represents dates and times internally. Go uses the time package to work with time-related data, which offers a robust and flexible system for managing time. At its core, the time.Time struct is the cornerstone of time manipulation in Go.
1.1. Creating Time Objects
To create a time.Time object representing the current time, you can use the time.Now() function:
go package main import ( "fmt" "time" ) func main() { currentTime := time.Now() fmt.Println("Current time:", currentTime) }
This will output the current time in your system’s timezone. However, when dealing with dates and times in applications, you often need to work with different timezones and format the output according to specific requirements.
2. Handling Timezones
Dealing with timezones is a common challenge in programming, especially when your application interacts with users or systems in various parts of the world. Fortunately, Go’s time package provides excellent support for handling timezones.
2.1. Setting a Specific Timezone
To work with a specific timezone, you can use the time.LoadLocation function. This function allows you to load a location by its name, such as “America/New_York” or “UTC,” and then create a time.Time object with that location.
go package main import ( "fmt" "time" ) func main() { // Load the "America/New_York" timezone newYork, err := time.LoadLocation("America/New_York") if err != nil { fmt.Println("Error:", err) return } // Create a time object with the specified timezone currentTime := time.Now().In(newYork) fmt.Println("Current time in New York:", currentTime) }
In this example, we load the “America/New_York” timezone and use it to display the current time in New York. The .In() method is used to convert the time to the desired timezone.
2.2. Converting Time to Different Timezones
You can also convert a time.Time object from one timezone to another using the .In() method. This is especially useful when you have timestamps in one timezone and need to display them in another.
go package main import ( "fmt" "time" ) func main() { // Create a time object in UTC currentTimeUTC := time.Now().UTC() // Convert it to the "America/Los_Angeles" timezone losAngeles, err := time.LoadLocation("America/Los_Angeles") if err != nil { fmt.Println("Error:", err) return } currentTimeLosAngeles := currentTimeUTC.In(losAngeles) fmt.Println("Current time in Los Angeles:", currentTimeLosAngeles) }
In this example, we first create a time.Time object representing the current time in UTC. Then, we convert it to the “America/Los_Angeles” timezone using the .In() method.
2.3. Timezone Abbreviations
While working with timezones, it’s essential to note that timezone abbreviations can change due to daylight saving time (DST) transitions. To display the full timezone name, you can use the .String() method on a time.Time object:
go package main import ( "fmt" "time" ) func main() { newYork, _ := time.LoadLocation("America/New_York") currentTime := time.Now().In(newYork) fmt.Println("Full timezone name:", currentTime.String()) }
This will print the full timezone name, including information about DST, if applicable.
3. Formatting Dates and Times
Formatting dates and times in Go is flexible and allows you to generate output in various styles and formats. Go uses a special format string to define the desired output format.
3.1. Formatting a Time Object
To format a time.Time object, you can use the .Format() method and provide a format string that specifies how you want the time to be displayed. Here are some common format options:
- “2006-01-02 15:04:05” for a standard date and time format.
- “Monday, Jan 2, 2006 15:04:05” for a more verbose format including the day and month names.
- “02/01/2006” for a date format commonly used in some regions.
go package main import ( "fmt" "time" ) func main() { currentTime := time.Now() // Format the current time formattedTime := currentTime.Format("Monday, Jan 2, 2006 15:04:05") fmt.Println("Formatted time:", formattedTime) }
This will display the current time in the specified format.
3.2. Parsing Strings into Time Objects
Conversely, if you have a string representing a date or time in a particular format, you can parse it into a time.Time object using the time.Parse() function:
go package main import ( "fmt" "time" ) func main() { // A date and time string in a specific format dateString := "2023-09-13 12:34:56" // Define the expected format format := "2006-01-02 15:04:05" // Parse the string into a time object parsedTime, err := time.Parse(format, dateString) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Parsed time:", parsedTime) }
In this example, we parse a date and time string into a time.Time object by specifying the expected format in the time.Parse() function.
3.3. Predefined Format Constants
Go also provides predefined constants for common date and time formats, making it easier to work with standard representations. For example, you can use time.RFC3339 to work with timestamps in the RFC3339 format:
go package main import ( "fmt" "time" ) func main() { currentTime := time.Now() // Format the current time in RFC3339 format formattedTime := currentTime.Format(time.RFC3339) fmt.Println("RFC3339 formatted time:", formattedTime) }
4. Combining Timezone Handling and Formatting
Now that we’ve explored handling timezones and formatting separately, let’s combine these concepts to work with timezones and display formatted timestamps correctly.
Example: Displaying Times in Different Timezones
Suppose you have a timestamp in UTC and need to display it in multiple timezones for an international audience. You can load different timezones and format the time accordingly:
go package main import ( "fmt" "time" ) func main() { // Create a timestamp in UTC currentTimeUTC := time.Now().UTC() // Load different timezones newYork, _ := time.LoadLocation("America/New_York") london, _ := time.LoadLocation("Europe/London") sydney, _ := time.LoadLocation("Australia/Sydney") // Format and display the time in different timezones fmt.Println("Current time in New York:", currentTimeUTC.In(newYork).Format("Monday, Jan 2, 2006 15:04:05 MST")) fmt.Println("Current time in London:", currentTimeUTC.In(london).Format("Monday, Jan 2, 2006 15:04:05 MST")) fmt.Println("Current time in Sydney:", currentTimeUTC.In(sydney).Format("Monday, Jan 2, 2006 15:04:05 MST")) }
In this example, we create a timestamp in UTC and then use the .In() method to convert and format it in different timezones. The MST format specifier represents the timezone abbreviation.
Conclusion
Working with dates and times in Go is made more accessible by the powerful time package. You can handle timezones, format timestamps, and perform various operations with precision. By understanding the core concepts of time representation, timezone handling, and formatting, you can build robust applications that accurately manage and display time-related data. Remember to consider the specific requirements of your project and choose the appropriate time-related functions and formats to ensure correctness and user-friendliness in your applications. Happy coding!
Table of Contents
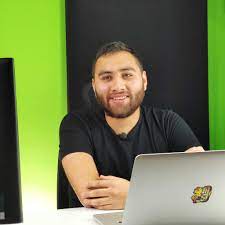
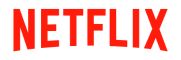