How do you handle file I/O in Go?
In Go, file I/O (Input/Output) operations are performed using the os and io/ioutil packages, which provide functions and utilities for reading from and writing to files on the filesystem.
Here’s an overview of how file I/O is typically handled in Go:
Opening Files: To open a file for reading or writing, you use the os.Open or os.Create functions, respectively. These functions return a file descriptor (*os.File) that represents the opened file.
go file, err := os.Open("example.txt") if err != nil { log.Fatal(err) } defer file.Close()
Reading from Files: To read data from a file, you can use various methods provided by the os.File type, such as Read, ReadAt, and ReadAll, or the io/ioutil package’s ReadFile function.
go data, err := ioutil.ReadFile("example.txt") if err != nil { log.Fatal(err) } fmt.Println(string(data))
Writing to Files: To write data to a file, you use methods provided by the os.File type, such as Write, WriteString, and WriteAt, or the io/ioutil package’s WriteFile function.
go data := []byte("Hello, world!") err := ioutil.WriteFile("output.txt", data, 0644) if err != nil { log.Fatal(err) }
Closing Files: It’s important to close files after you’ve finished using them to free up system resources. You can use the Close method provided by the os.File type to close an open file.
go file.Close()
In addition to basic file I/O operations, Go also provides support for file manipulation, directory operations, file permissions, and symbolic links through functions and utilities available in the os package.
Go’s file I/O facilities offer a simple and efficient way to interact with files and directories, making it easy to perform common file-related tasks in Go programs.
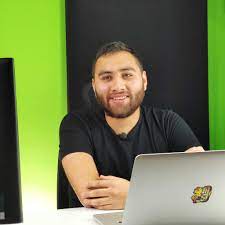
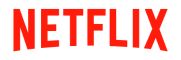