How do you format strings in Go?
In Go, string formatting is typically accomplished using the fmt package, which provides functions for formatting and printing strings, numbers, and other data types. The fmt package follows a syntax similar to the C language’s printf function for formatting strings with placeholders.
The most commonly used function for string formatting in Go is fmt.Sprintf, which formats a string according to a format specifier and returns the resulting string. The format specifier consists of % followed by a verb that represents the type of value to be formatted. For example, %s is used to format strings, %d for integers, %f for floating-point numbers, and so on.
Here’s a basic example of string formatting using fmt.Sprintf:
go package main import ( "fmt" ) func main() { name := "John" age := 30 height := 6.2 formattedString := fmt.Sprintf("Name: %s, Age: %d, Height: %.2f feet", name, age, height) fmt.Println(formattedString) }
Output:
yaml Name: John, Age: 30, Height: 6.20 feet
In addition to fmt.Sprintf, the fmt package also provides other functions like fmt.Printf for printing formatted strings to the standard output, fmt.Fprintf for printing to a specified file or io.Writer, and fmt.Sprint for formatting without printing.
The fmt package supports a wide range of formatting options and modifiers, allowing developers to customize the appearance of formatted strings according to their requirements. By leveraging the capabilities of the fmt package, developers can create clear, readable, and well-formatted output for various purposes, including logging, debugging, and user interface display.
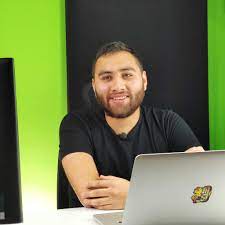
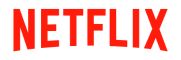