Delving into the World of Goroutines and Channels: Mastering Concurrency in Go
Go, also known as Golang, has become a favored programming language among developers, leading to a surge in businesses looking to hire Golang developers. The language’s simplicity, performance, and robust tooling make it an excellent choice for a wide array of projects. One of its most appealing features, and a primary reason businesses hire Golang developers, is its native support for concurrency through goroutines and channels. In this article, we’ll deep dive into these core elements of Go’s concurrency model. By exploring practical examples, we will illustrate their use and provide a glimpse into the skills you’d tap into when you hire Golang developers.
Understanding Concurrency
Concurrency is the ability to run multiple tasks simultaneously, improving the efficiency of your application. It’s essential for modern web applications handling several requests simultaneously or computations that can be broken into independent tasks. Although concurrency is often associated with parallelism (multiple tasks running simultaneously on different cores), it doesn’t always mean the same thing. Concurrency focuses on dealing with a lot of things at once, while parallelism emphasizes doing a lot of things at once.
What are Goroutines?
Goroutines are functions or methods that run concurrently with others. They are lightweight threads managed by the Go runtime. Creating a goroutine is simple; prepend the function call with the `go` keyword:
```go func helloWorld() { fmt.Println("Hello, World!") } func main() { go helloWorld() time.Sleep(1 * time.Second) // pause the main function to allow the goroutine to complete } ```
In this example, `helloWorld()` runs concurrently with `main()`. The `time.Sleep()` function ensures the `main()` function doesn’t exit before the goroutine completes, as the program would end otherwise.
Channels: Communicating Between Goroutines
While goroutines enable concurrent operations, communication between these goroutines is crucial for synchronizing tasks or exchanging data. This communication is facilitated through channels.
You can think of channels as conduits or pipes that connect concurrent goroutines, allowing them to send or receive values. Here’s a basic example:
```go func greet(c chan string) { fmt.Println("Hello " + <-c + "!") } func main() { msgChannel := make(chan string) go greet(msgChannel) msgChannel <- "World" time.Sleep(1 * time.Second) } ```
Here, we make a channel `msgChannel` that passes strings. The `greet()` goroutine reads from the channel using `<-c`, while the main goroutine writes to the channel using `msgChannel <- “World”`.
Buffered Channels and Select Statements
Channels are unbuffered by default, meaning they will only hold a value when there is a receiver ready to accept it. However, Go supports buffered channels, which can hold multiple values without requiring immediate pickup:
```go func main() { channel := make(chan string, 2) channel <- "Hello" channel <- "World" fmt.Println(<-channel) fmt.Println(<-channel) } ```
In this example, the `channel` has a buffer size of 2, so it can hold two values simultaneously.
Additionally, Go provides the `select` keyword to handle multiple channel operations, allowing you to choose the first operation that’s ready:
```go func main() { chan1 := make(chan string) chan2 := make(chan string) go func() { time.Sleep(1 * time.Second) chan1 <- "one" }() go func() { time.Sleep(2 * time.Second) chan2 <- "two" }() for i := 0; i < 2; i++ { select { case msg1 := <-chan1: fmt.Println("received", msg1) case msg2 := <-chan2: fmt.Println("received", msg2) } } } ```
This code creates two goroutines that send values to `chan1` and `chan2` after sleeping for 1 and 2 seconds, respectively. The `select` statement waits for either channel to be ready and then executes the corresponding case.
Conclusion
Concurrency in Go, facilitated by goroutines and channels, is a powerful feature that enables efficient multitasking in your applications. Its simplicity of implementation – just prefixing your function with `go` and establishing communication using channels – is part of what makes Go a preferred choice for many developers.
However, it’s also important to be mindful of potential pitfalls, like data races and deadlocks. This is especially crucial when you hire Golang developers, as their understanding of these potential issues can significantly influence your application’s performance and reliability. Always ensure that goroutines have completed their tasks before the main program exits and avoid scenarios where goroutines are indefinitely waiting to receive or send data.
As with any language or feature, practice and understanding are key. When you hire Golang developers, look for those who continually incorporate these principles into their work, demonstrating a solid grasp of goroutines and channels. By doing so, they can harness the power of concurrency in Go and optimize your applications to their fullest potential.
Table of Contents
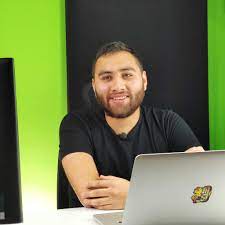
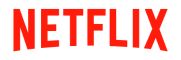