Conquering Golang: A Detailed Guide to Mastering the Go Programming Language
In the ever-evolving world of programming languages, Go, often referred to as Golang, has emerged as a sought-after skills for hiring Golang developers. Golang is an efficient, statically-typed compiled language renowned for its high performance. Introduced by Google in 2007, Go’s simplicity, efficiency, and powerful built-in concurrency have propelled its popularity among developers and increased the demand to hire Golang developers.
This guide will walk you through the key concepts of the Go language, providing examples to help not only those looking to master the language themselves, but also those looking to understand the skills required to hire effective Golang developers.
.
Understanding the Basics of Go
Variables and Data Types
In Go, variables are declared using the `var` keyword, followed by the variable name and the type. If an initial value is given, Go can determine the type automatically. Go supports several data types such as integers, floating-point numbers, boolean, and string.
```go var name string = "Gopher" var age int = 25 // Short declaration city := "San Francisco" ```
Functions
A function in Go is declared using the `func` keyword, followed by the function name. The parameters and their types go inside parentheses, followed by the return type. If there are no parameters or return values, the parentheses are still required.
```go func add(x int, y int) int { return x + y } result := add(10, 20) // result is 30 ```
In Go, functions can return multiple values, a powerful feature that enables handling errors effectively.
```go func divide(x, y float64) (float64, error) { if y == 0.0 { return 0.0, errors.New("division by zero is not allowed") } return x / y, nil } result, err := divide(20.0, 0.0) if err != nil { log.Fatal(err) } ```
Arrays and Slices
Arrays in Go are fixed-length, ordered collections of elements of the same type. The length forms part of the array’s type. For instance, `[4]int` and `[5]int` are distinct types.
```go var numbers [5]int numbers = [5]int{1, 2, 3, 4, 5} ``` Slices, on the other hand, are more flexible. They're like arrays but without a fixed size. ```go var names []string names = []string{"Alice", "Bob", "Charlie"} ```
Unleashing Go’s Concurrency Model: Goroutines and Channels
One of the defining characteristics of Go is its native support for concurrency. In Go, concurrency is achieved using Goroutines and Channels.
Goroutines
A Goroutine is a lightweight thread of execution managed by the Go runtime. It allows functions to run concurrently with others. To launch a Goroutine, use the `go` keyword followed by a function invocation:
```go func printNumbers() { for i := 1; i <= 10; i++ { fmt.Println(i) } } func printLetters() { for i := 'a'; i <= 'j'; i++ { fmt.Println(string(i)) } } func main() { go printNumbers() go printLetters() time.Sleep(time.Second * 2) // Gives enough time for goroutines to finish } ```
Channels
Channels provide a way for two Goroutines to communicate with each other and synchronize their execution. Here is a simple example of using channels
```go func produce(c chan int) { for i := 0; i < 10; i++ { c <- i } close(c) } func consume (c chan int) { for i := range c { fmt.Println(i) } } func main() { c := make(chan int) go produce(c) consume(c) }
In this example, the `produce` Goroutine produces values that the `consume` Goroutine consumes. The channel `c` serves as a conduit between the two Goroutines.
Structs and Interfaces: Building Blocks of Go’s Type System
Structs
Structs are custom types that allow you to group/combine items of possibly different types into a single type.
```go type Employee struct { Name string Age int Salary float64 } // Creating a new Employee john := Employee{ Name: "John Doe", Age: 32, Salary: 50000.0, } ```
Interfaces
Interfaces provide a way to specify the behavior of an object. If a type provides certain methods, it implements the interface.
```go type Shape interface { Area() float64 } type Circle struct { Radius float64 } type Rectangle struct { Width, Height float64 } // Implementing the Shape interface for Circle func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius } // Implementing the Shape interface for Rectangle func (r Rectangle) Area() float64 { return r.Width * r.Height } func printArea(s Shape) { fmt.Println(s.Area()) } func main() { c := Circle{Radius: 5.0} r := Rectangle{Width: 5.0, Height: 7.0} printArea(c) printArea(r) } ```
Error Handling in Go
Go stands out among many languages as it does not support exceptions. Instead, it opts for a unique approach where errors are returned as normal return values. This paradigm is one of the aspects that those looking to hire Golang developers should be aware of. If a function can cause an error, it usually returns the last return value as an error, a distinctive characteristic that Golang developers should master.
```go func openFile(path string) (*os.File, error) { f, err := os.Open(path) if err != nil { return nil, err } return f, nil } func main() { f, err := openFile("invalid/path") if err != nil { log.Fatal(err) } // Work with file f } ```
Conclusion
Go’s simplicity, readability, and powerful concurrency model make it a compelling choice for many types of software development, increasing the demand to hire Golang developers who have mastered these qualities. This guide has touched on some fundamental concepts of the Go programming language, including variables, functions, arrays, slices, goroutines, channels, structs, interfaces, and error handling. By understanding these, you’ve taken a significant step towards mastering Go, whether for your personal development or to evaluate skills when you’re looking to hire Golang developers.
Remember, the journey to master any language, especially as a Golang developer, involves continuous learning and practice. To continue advancing your Go skills, consider building small projects, contributing to Go-based open source projects, or solving coding challenges in Go. This can also serve as a practical guide for those who wish to hire Golang developers, as it gives insights into the essential skills to look for. Happy coding!
Table of Contents
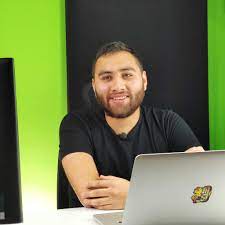
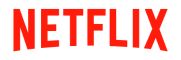