Implementing Caching in Go: Boosting Performance with Memory Caches
In the world of software development, performance is paramount. Users expect applications to respond swiftly and seamlessly, regardless of the complexity of the underlying processes. One way to achieve this is by implementing caching mechanisms, and in this article, we’ll delve into the world of memory caching in Go. Memory caching can significantly enhance the speed and responsiveness of your applications, making them more efficient and user-friendly.
1. Why Caching Matters?
Before diving into the technical details, let’s understand why caching is crucial for application performance. In a nutshell, caching involves storing frequently accessed data in a faster storage system, such as memory, to reduce the need for repeated computations or time-consuming data retrieval from slower sources like databases. This optimization technique can lead to substantial performance improvements, especially when dealing with data that doesn’t change frequently.
Imagine a scenario where a web application retrieves a set of configuration settings from a database every time a user makes a request. Without caching, this could lead to database bottlenecks, slowing down the application’s response time. By caching the configuration settings in memory, the application can quickly serve subsequent requests without hitting the database repeatedly.
2. Introducing Memory Caches
Memory caching involves storing frequently used data in the system’s main memory (RAM) rather than fetching it from a disk-based storage system like a hard drive or a database. RAM access is much faster than disk access, resulting in significantly reduced data retrieval times. There are various memory cache libraries available for Go, each offering different features and capabilities. In this article, we’ll explore a simple example using the popular go-cache library.
3. Getting Started with go-cache
1. To begin, let’s install the go-cache library:
bash go get github.com/patrickmn/go-cache
2. Next, we’ll import the package into our Go code:
go import ( "github.com/patrickmn/go-cache" )
With the library imported, we can now create a cache instance and start caching data.
4. Creating a Cache Instance
Here’s how you can create a cache instance using go-cache:
go func main() { // Create a new cache instance with a default expiration time of 5 minutes c := cache.New(5*time.Minute, 10*time.Minute) // Store data in the cache c.Set("key", "value", cache.DefaultExpiration) // Retrieve data from the cache if data, found := c.Get("key"); found { fmt.Println("Data from cache:", data) } }
In this example, we’ve created a cache instance with a default item expiration time of 5 minutes and a cleanup interval of 10 minutes. The Set function is used to store data in the cache, and the Get function retrieves data from the cache.
5. Customizing Cache Behavior
The go-cache library provides various options to customize the behavior of the cache. You can set a specific expiration time for each item, remove items from the cache, and even run a cleanup routine to remove expired items automatically. Here’s an example of how you can use these features:
go func main() { c := cache.New(5*time.Minute, 10*time.Minute) // Store data with a custom expiration time of 2 minutes c.Set("key1", "value1", 2*time.Minute) // Store data with a custom expiration time and callback on expiration c.SetWithExpirationCallback("key2", "value2", 3*time.Minute, func(key string, value interface{}) { fmt.Println("Item expired:", key, value) }) // Retrieve and print data from the cache if data, found := c.Get("key1"); found { fmt.Println("Data from cache (key1):", data) } if data, found := c.Get("key2"); found { fmt.Println("Data from cache (key2):", data) } // Remove an item from the cache c.Delete("key1") // Clean up the cache, removing expired items c.DeleteExpired() }
In this example, we’ve demonstrated how to set custom expiration times for items using the Set and SetWithExpirationCallback functions. Additionally, we’ve shown how to remove items from the cache using the Delete method and how to perform a cleanup to remove expired items using the DeleteExpired method.
6. Caching Strategies and Considerations
While memory caching can significantly boost performance, it’s essential to choose the right caching strategy for your application. Here are a few considerations to keep in mind:
6.1. Cache Invalidation
Data in the cache might become outdated if the source data changes. Implement cache invalidation mechanisms to ensure that the cached data remains accurate. This might involve updating the cache when the source data changes or setting an appropriate expiration time.
6.2. Cache Size
Caches have limited memory, so it’s crucial to manage the cache size effectively. Evict less frequently used items or implement a strategy like Least Recently Used (LRU) to make room for new items.
6.3. Hot and Cold Data
Identify which data is “hot” (frequently accessed) and which is “cold” (infrequently accessed). Consider implementing a multi-tiered caching approach, where frequently accessed data resides in a faster but smaller cache, while less frequently accessed data is stored in a larger but slower cache.
6.4. Cache-Aside vs. Read-Through
Choose between cache-aside and read-through caching strategies. Cache-aside involves the application code being responsible for managing the cache, while read-through caching delegates cache management to the caching library.
Conclusion
In this article, we’ve explored the world of memory caching in Go, focusing on the go-cache library as an example. We’ve learned why caching is crucial for application performance and how memory caching can significantly boost responsiveness by reducing data retrieval times. By implementing caching strategies and considering cache invalidation, size management, and data access patterns, you can create highly performant applications that provide a seamless user experience.
Remember, caching is a powerful tool, but it’s not a one-size-fits-all solution. Carefully analyze your application’s requirements and data access patterns to determine the most suitable caching strategy. With the right approach, you can harness the benefits of memory caching and deliver applications that excel in both speed and efficiency.
Table of Contents
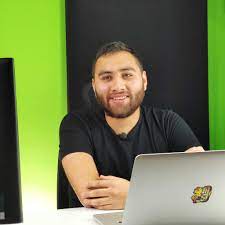
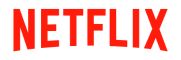