Interview Guide: Hire Go Developers
Go, also known as Golang, has gained popularity as a powerful and efficient programming language for building scalable and concurrent applications. Hiring skilled Go developers is crucial to ensure the success of your projects.
Table of Contents
This comprehensive interview guide will help you identify top Go talent and build a proficient development team.
1. Key Skills of Go Developers to Look Out For:
Before diving into the interview process, take note of the crucial skills to look for in Go developers:
- Proficiency in Go Language: Strong knowledge of Go language fundamentals, including goroutines, channels, and concurrency.
- Web Development with Go: Experience in building web applications using Go and popular frameworks like Gin or Echo.
- RESTful APIs and Microservices: Familiarity with building and consuming RESTful APIs and developing microservices architecture.
- Database Interaction: Knowledge of interacting with databases, both SQL and NoSQL, using Go.
- Testing and Debugging: Experience in writing unit tests and debugging Go applications for reliability.
- DevOps Skills: Understanding of Docker, Kubernetes, and continuous integration/continuous deployment (CI/CD) pipelines.
2. An Overview of the Go Developer Hiring Process:
- Defining Job Requirements & Skills: Clearly outline the technical skills and experience you are seeking in a Go developer, including Go language proficiency, web development expertise, and experience with microservices.
- Creating an Effective Job Description: Craft a comprehensive job description with a clear title, specific requirements, and details about the projects the developer will work on.
- Preparing Interview Questions: Develop a list of technical questions to assess candidates’ Go knowledge, problem-solving abilities, and experience in building scalable applications.
- Evaluating Candidates: Use a combination of technical assessments, coding challenges, and face-to-face interviews to evaluate potential candidates and determine the best fit for your organization.
3. Technical Interview Questions and Sample Answers:
Q1. Explain the concept of goroutines and how they differ from threads in traditional programming languages.
Sample Answer:
Goroutines are lightweight concurrent functions in Go that can be executed concurrently with other goroutines. They are managed by the Go runtime and allow developers to write concurrent code with ease. Goroutines differ from threads in traditional languages as they are more efficient in terms of memory usage, and Go manages them with a single operating system thread, making it easier to create thousands of goroutines without running out of resources.
Q2. How do you handle errors in Go? Explain the use of the error
type and panic
function.
Sample Answer:
In Go, errors are represented using the error
type, which is an interface with a single method, Error() string
. We handle errors using the if err != nil
pattern. Additionally, we can use the panic
function to terminate the program immediately and display an error message. However, panic
should be used judiciously, mainly for unrecoverable errors.
Q3. Explain how you would build a simple web server using the standard net/http
package in Go.
Sample Answer:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In this example, we define a handler function that writes “Hello, World!” to the HTTP response. We then use http.HandleFunc
to map the handler to the root path (“/”) and http.ListenAndServe
to start the web server on port 8080.
Q4. How do you perform database operations with MySQL in Go? Provide an example of querying data from a MySQL database.
Sample Answer:
package main import ( "database/sql" _ "github.com/go-sql-driver/mysql" "log" ) func main() { db, err := sql.Open("mysql", "username:password@tcp(127.0.0.1:3306)/database_name") if err != nil { log.Fatal(err) } defer db.Close() rows, err := db.Query("SELECT id, name FROM users") if err != nil { log.Fatal(err) } defer rows.Close() for rows.Next() { var id int var name string if err := rows.Scan(&id, &name); err != nil { log.Fatal(err) } fmt.Println(id, name) } }
In this example, we use the database/sql
package along with the MySQL driver (github.com/go-sql-driver/mysql
) to connect to a MySQL database and query data from the “users” table.
Q5. Explain the concept of middleware in Go web frameworks. How do you implement middleware using Gorilla Mux?
Sample Answer:
Middleware in Go web frameworks is a way to intercept and process HTTP requests and responses before they reach the actual handler. It allows developers to perform tasks such as authentication, logging, and error handling. In Gorilla Mux, we can implement middleware by creating a function that takes an http.Handler
as input and returns a new http.Handler
. The middleware function can then perform pre-processing before calling the next handler in the chain.
package main import ( "net/http" "github.com/gorilla/mux" ) func LoggerMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Perform logging or pre-processing here next.ServeHTTP(w, r) }) } func main() { router := mux.NewRouter() router.Use(LoggerMiddleware) router.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, Middleware!")) }) http.Handle("/", router) http.ListenAndServe(":8080", nil) }
In this example, we create a LoggerMiddleware
function that logs requests before calling the next handler in the chain. We use router.Use
to apply the middleware globally to all routes.
Q6. Explain the concept of channels in Go. How do you use channels for communication between goroutines?
Sample Answer:
Channels in Go are a built-in feature used for communication and synchronization between goroutines. They allow safe data transfer between concurrent goroutines. We use the make
function to create channels, and the <-
operator to send and receive data through channels.
package main import "fmt" func worker(id int, jobs <-chan int, results chan<- int) { for job := range jobs { fmt.Printf("Worker %d processing job %d\n", id, job) results <- job * 2 } } func main() { numJobs := 5 jobs := make(chan int, numJobs) results := make(chan int, numJobs) // Start multiple workers for w := 1; w <= 3; w++ { go worker(w, jobs, results) } // Send jobs to workers for j := 1; j <= numJobs; j++ { jobs <- j } close(jobs) // Collect results from workers for r := 1; r <= numJobs; r++ { <-results } }
In this example, we create two channels, jobs
and results
. We start three worker goroutines, and then send five jobs to the jobs
channel. The worker goroutines process the jobs and send the results to the results
channel, which we later collect.
Q7. How do you handle concurrent access to shared resources in Go? Explain the use of mutexes for synchronization.
Sample Answer:
In Go, to prevent concurrent access to shared resources, we use mutexes for synchronization. A mutex is a mutual exclusion lock that allows only one goroutine to access the shared resource at a time. We use the sync.Mutex
type to create mutexes and the Lock
and Unlock
methods to protect critical sections of code.
package main import ( "fmt" "sync" ) type Counter struct { count int mu sync.Mutex } func (c *Counter) Increment() { c.mu.Lock() defer c.mu.Unlock() c.count++ } func (c *Counter) GetCount() int { c.mu.Lock() defer c.mu.Unlock() return c.count } func main() { var counter Counter for i := 0; i < 1000; i++ { go counter.Increment() } // Wait for goroutines to finish time.Sleep(time.Second) fmt.Println(counter.GetCount()) }
In this example, we create a Counter
type with a mutex. The Increment
and GetCount
methods use the mutex to ensure that only one goroutine can modify the count
variable at a time.
Q8. How do you handle errors in concurrent Go code? Explain the use of the errgroup
package for managing goroutines and their errors.
Sample Answer:
In concurrent Go code, managing errors can be challenging, especially when dealing with multiple goroutines. The errgroup
package from the golang.org/x/sync/errgroup
module helps manage goroutines and their errors. It allows us to run multiple goroutines concurrently and wait for all of them to complete or for any error to occur.
package main import ( "context" "fmt" "golang.org/x/sync/errgroup" ) func worker(ctx context.Context, id int) error { // Simulate work return nil } func main() { var g errgroup.Group for i := 1; i <= 5; i++ { id := i g.Go(func() error { return worker(context.Background(), id) }) } if err := g.Wait(); err != nil { fmt.Println("Error:", err) } else { fmt.Println("All goroutines completed successfully.") } }
In this example, we use the errgroup.Group
to manage multiple worker goroutines. Each goroutine calls the worker
function, and we use g.Wait()
to wait for all goroutines to complete or for any error to occur.
Q9. How do you work with JSON data in Go? Explain the use of the encoding/json
package for JSON serialization and deserialization.
Sample Answer:
In Go, we use the encoding/json
package for JSON serialization and deserialization. We can convert Go data structures to JSON format using json.Marshal
, and vice versa using json.Unmarshal
.
package main import ( "encoding/json" "fmt" ) type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` } func main() { // JSON serialization p1 := Person{Name: "John Doe", Age: 30, Email: "john@example.com"} data, err := json.Marshal(p1) if err != nil { fmt.Println("Error:", err) return } fmt.Println(string(data)) // JSON deserialization var p2 Person jsonStr := `{"name":"Jane Smith","age":25,"email":"jane@example.com"}` err = json.Unmarshal([]byte(jsonStr), &p2) if err != nil { fmt.Println("Error:", err) return } fmt.Printf("Name: %s, Age: %d, Email: %s\n", p2.Name, p2.Age, p2.Email) }
In this example, we define a Person
struct and use json.Marshal
to serialize a Person
object to JSON format. We then use json.Unmarshal
to deserialize a JSON string back into a Person
object.
Q10. Explain the concept of interfaces in Go. How do you use interfaces to achieve polymorphism?
Sample Answer:
In Go, interfaces are a collection of method signatures. They define the behavior of an object, but the object’s underlying type determines the implementation of these methods. Interfaces enable polymorphism by allowing different types to satisfy the same interface and be used interchangeably in code that relies on the interface.
package main import "fmt" type Shape interface { Area() float64 } type Circle struct { Radius float64 } type Rectangle struct { Width float64 Height float64 } func (c Circle) Area() float64 { return 3.14 * c.Radius * c.Radius } func (r Rectangle) Area() float64 { return r.Width * r.Height } func PrintArea(s Shape) { fmt.Printf("Area: %.2f\n", s.Area()) } func main() { c := Circle{Radius: 5} r := Rectangle{Width: 4, Height: 3} PrintArea(c) PrintArea(r) }
In this example, we define a Shape
interface with an Area()
method. The Circle
and Rectangle
types both implement this interface, allowing us to use them interchangeably in the PrintArea
function.
With these technical questions and sample answers, you can confidently interview and evaluate potential Go developers for your projects. Tailor the questions to your specific needs and requirements to ensure you hire the best-fit Go developers for your organization. Good luck with your hiring process!
4. The Importance of Hiring the Right Go Developers
Hiring the right Go developers is a critical step in building high-quality, performant, and scalable applications. Skilled Go developers bring several advantages to your organization:
- Expertise in Efficient Concurrency: Go’s concurrency features, such as goroutines and channels, allow developers to build concurrent programs easily. Experienced Go developers understand how to effectively utilize these features to create applications that can handle thousands of concurrent requests without sacrificing performance.
- Highly Performant Applications: Go is renowned for its speed and efficiency. Seasoned Go developers know how to write optimized code, reducing resource consumption and improving the overall performance of your applications.
- Microservices and Cloud-Native Development: Go is well-suited for building microservices and cloud-native applications. Skilled Go developers can design and implement microservices architecture, enabling your organization to scale and deploy applications efficiently in cloud environments.
- Robust Web Development: With Go’s web frameworks like Gin and Echo, Go developers can build robust and scalable web applications. They have the expertise to create RESTful APIs, handle HTTP requests efficiently, and build responsive user interfaces.
- Collaborative Team Players: Seasoned Go developers are team players who can collaborate effectively with other developers, designers, and stakeholders. Their ability to communicate ideas and work cohesively in a team environment ensures smooth project development.
- Error Handling and Testing: Go developers pay special attention to error handling and testing. They write unit tests to ensure the reliability and correctness of their code, minimizing potential bugs and vulnerabilities.
5. How CloudDevs Can Help You Hire Go Developers
Finding top Go developers can be challenging and time-consuming. CloudDevs simplifies the hiring process and connects you with the best-fit Go talent for your projects. Here’s how CloudDevs can assist you:
- Pre-Screened Senior Developers: CloudDevs maintains a pool of pre-screened senior Go developers with proven expertise and experience. This eliminates the need for you to go through an extensive screening process, saving you time and effort.
- Dedicated Consultants: CloudDevs provides dedicated consultants who will discuss your project requirements and help you define the skills and experience you need in a Go developer.
- Curated Shortlist: Within 24 hours, CloudDevs presents you with a curated shortlist of top Go developers that match your project’s requirements. This saves you the hassle of sifting through numerous applications.
- Technical Assessments and Interviews: CloudDevs helps you conduct technical assessments and interviews to evaluate candidates’ Go skills and proficiency. This ensures that you find developers who meet your technical standards.
- Risk-Free Trial: CloudDevs offers a risk-free trial period for your chosen Go developer. This allows you to assess their compatibility with your team and project before making a long-term commitment.
- Smooth Onboarding: CloudDevs assists in the onboarding process, making the transition seamless for the hired Go developer to start contributing to your projects effectively.
With CloudDevs’ expertise in talent acquisition and Go development, you can build a high-performing development team with ease. Trust CloudDevs to find the right Go developers who will drive the success of your projects and bring your vision to life.
Table of Contents
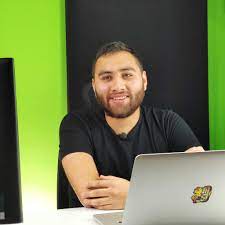
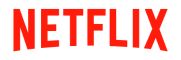