Working with PostgreSQL in Go: Database Connectivity and ORM
In the realm of backend development, the ability to seamlessly interact with databases is indispensable. Go, also known as Golang, is a programming language designed for efficiency and simplicity, making it an excellent choice for building high-performance applications. PostgreSQL, on the other hand, is a powerful open-source relational database management system (RDBMS) known for its reliability and extensibility. When these two technologies come together, they form a potent combination for building data-driven applications.
In this comprehensive guide, we’ll delve into the intricacies of working with PostgreSQL in Go, exploring how to establish a robust database connection and harness the power of Object-Relational Mapping (ORM) for efficient data manipulation. Whether you’re a seasoned developer or just starting with Go and databases, this tutorial will equip you with the knowledge and skills needed to handle database operations effectively.
1. Setting Up PostgreSQL
1.1. Installing PostgreSQL
Before we can start working with PostgreSQL, we need to install it on our system. If you haven’t already, follow these steps to install PostgreSQL:
bash # On Linux (Ubuntu) sudo apt update sudo apt install postgresql postgresql-contrib # On macOS (using Homebrew) brew install postgresql # On Windows (using Chocolatey) choco install postgresql
1.2. Creating a Database
Once PostgreSQL is installed, we can create a database for our Go application. This can be done using the createdb command:
bash createdb myappdb
1.3. Configuring Connection Parameters
To connect to the PostgreSQL database from your Go application, you’ll need to specify connection parameters such as the database name, username, and password. These parameters can be configured in your Go code or provided as environment variables for added security.
In your Go code, you can use a connection string like this:
go const ( host = "localhost" port = 5432 user = "your_username" password = "your_password" dbname = "myappdb" ) func main() { connStr := fmt.Sprintf("host=%s port=%d user=%s password=%s dbname=%s sslmode=disable", host, port, user, password, dbname) // Use connStr to establish a connection }
Now that we’ve set up PostgreSQL and configured the connection parameters, it’s time to establish a connection to the database from our Go application.
2. Database Connectivity in Go
2.1. Importing the Required Packages
In Go, the database/sql package provides a general interface around SQL (or SQL-like) databases. To work with PostgreSQL, you’ll also need a PostgreSQL driver. The most commonly used driver is pq. You can install it using:
bash go get github.com/lib/pq
Next, import the necessary packages in your Go code:
go import ( "database/sql" "fmt" _ "github.com/lib/pq" )
The _ before the import is used to ensure that the pq package’s init() function is executed, registering it as a driver for database/sql.
2.2. Establishing a Database Connection
Now, let’s create a function to establish a database connection using the previously configured connection string:
go func connectDB() (*sql.DB, error) { connStr := fmt.Sprintf("host=%s port=%d user=%s password=%s dbname=%s sslmode=disable", host, port, user, password, dbname) db, err := sql.Open("postgres", connStr) if err != nil { return nil, err } err = db.Ping() if err != nil { return nil, err } return db, nil }
The sql.Open function creates a new database handle and the Ping method is used to check if the connection to the database is successful. If any errors occur during these steps, they will be returned.
2.3. Executing SQL Queries
With a database connection in place, you can execute SQL queries. Here’s an example of inserting data into a table:
go func insertData(db *sql.DB, name string, age int) error { insertSQL := "INSERT INTO users (name, age) VALUES ($1, $2)" _, err := db.Exec(insertSQL, name, age) return err }
The $1 and $2 placeholders are used to safely insert data and prevent SQL injection.
2.4. Handling Errors
It’s crucial to handle errors properly when working with databases. Always check for errors after executing queries or opening connections, and log or handle them accordingly to ensure your application’s robustness.
Now that we’ve covered the basics of database connectivity in Go, let’s move on to the world of Object-Relational Mapping (ORM).
3. Introduction to ORM (Object-Relational Mapping)
3.1. What Is ORM?
Object-Relational Mapping (ORM) is a programming technique that allows you to interact with a relational database using an object-oriented paradigm. Instead of writing raw SQL queries, you work with objects and classes that represent database tables and their relationships. ORM libraries handle the translation between these objects and the underlying SQL queries.
3.2. Why Use ORM?
ORM offers several advantages, including:
- Abstraction of database-specific details: You can write database-agnostic code.
- Improved code readability: ORM code is often more concise and easier to understand.
- Faster development: You can focus on business logic rather than writing SQL queries.
3.3. Choosing an ORM Library for Go
Go has several ORM libraries available, each with its own features and trade-offs. One popular choice is GORM, a full-featured ORM library that provides a wide range of capabilities for working with databases. Let’s explore how to use GORM to work with PostgreSQL in Go.
4. Using GORM for ORM in Go
4.1. Installing GORM
To get started with GORM, you’ll need to install it using Go modules:
bash go get -u github.com/go-gorm/gorm
Next, import GORM in your code:
go import ( "gorm.io/driver/postgres" "gorm.io/gorm" )
4.2. Defining Models
In GORM, you define database models as Go structs. Each struct represents a table in your database. For example, if you have a users table, you can define a corresponding Go struct:
go type User struct { gorm.Model Name string Age int }
The gorm.Model embedded struct provides fields like ID, CreatedAt, and UpdatedAt, which are commonly used in database models.
4.3. CRUD Operations with GORM
GORM simplifies CRUD (Create, Read, Update, Delete) operations. Here are some examples:
4.3.1. Create
go func createUser(db *gorm.DB, name string, age int) error { user := User{Name: name, Age: age} result := db.Create(&user) if result.Error != nil { return result.Error } return nil }
4.3.2. Read
go func getUserByID(db *gorm.DB, id uint) (User, error) { var user User result := db.First(&user, id) if result.Error != nil { return User{}, result.Error } return user, nil }
4.3.3. Update
go func updateUserAge(db *gorm.DB, id uint, newAge int) error { result := db.Model(&User{}).Where("id = ?", id).Update("age", newAge) if result.Error != nil { return result.Error } return nil }
4.3.4. Delete
go func deleteUser(db *gorm.DB, id uint) error { result := db.Delete(&User{}, id) if result.Error != nil { return result.Error } return nil }
GORM takes care of generating the necessary SQL queries and executing them, making your code more concise and readable.
4.4. Migrations
Migrations are an essential part of managing database schema changes. GORM provides a built-in migration feature to create and update database tables based on your Go models. Here’s how you can perform a migration:
go func runMigrations(db *gorm.DB) error { err := db.AutoMigrate(&User{}) if err != nil { return err } return nil }
With GORM, you can automate the process of keeping your database schema in sync with your Go code.
Conclusion
Working with PostgreSQL in Go can be a highly productive experience, thanks to the combination of Go’s performance and simplicity with PostgreSQL’s robustness and extensibility. By following the steps outlined in this guide, you can set up PostgreSQL, establish database connections, and leverage the power of GORM for seamless ORM in your Go applications.
Whether you’re building a web application, a microservice, or any data-driven software, mastering these database connectivity and ORM techniques will enable you to handle data effectively and efficiently. As you continue your journey with Go and databases, remember to explore additional features and best practices to optimize your development process further.
Now, armed with the knowledge of PostgreSQL and GORM in Go, you’re ready to embark on your database-driven coding adventures. Happy coding!
Table of Contents
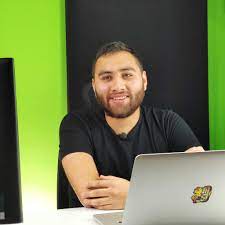
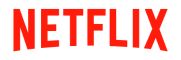