Introduction to Go’s Template Engines: Generating Dynamic Content
When building web applications, one of the most critical tasks is rendering dynamic content to display to users. Go, also known as Golang, is a versatile programming language renowned for its performance and efficiency, and it offers robust tools for web development. One of these tools is its template engine system, which enables developers to generate dynamic HTML, XML, or text content effortlessly. In this blog post, we’ll explore Go’s template engines, understand their fundamental concepts, and provide practical examples to demonstrate how they can be used effectively for dynamic content generation.
1. What Are Template Engines?
Template engines, often referred to simply as templates, are a mechanism for separating the structure and content of a web page or document. They allow developers to define the layout and structure of a page in a template file while inserting dynamic content using placeholders or variables. When a request is made to a web application, the template engine processes the template, replacing the placeholders with actual data, and generates the final output, typically in HTML format. This dynamic content generation approach simplifies web development by keeping the code organized and making it easier to maintain.
2. Go’s Templating Package: html/template
In Go, the standard library provides a powerful templating package called html/template. This package offers a flexible and secure way to generate HTML, XML, and other text-based formats. The html/template package ensures that the generated output is properly escaped, preventing common web vulnerabilities such as Cross-Site Scripting (XSS) attacks. It also enforces strict separation between the template logic and the data, which promotes cleaner code and security.
2.1. Getting Started with html/template
Before diving into the details, let’s see how easy it is to get started with Go’s html/template package. First, you need to import the package:
go import "html/template"
Now, you can create a simple template by defining a string that represents the HTML structure with placeholders enclosed in double curly braces:
go const templateString = "<html><body><h1>Hello, {{.Name}}!</h1></body></html>"
In this example, {{.Name}} is a placeholder that will be replaced with dynamic data. The .Name part is a reference to a field in the data that we will provide later.
Next, you can parse the template string to create a template:
go t, err := template.New("greeting").Parse(templateString) if err != nil { panic(err) }
Now, you have a template ready to use. To render the template with data, you can create a data structure and execute the template:
go data := struct{ Name string }{"John"} err = t.Execute(os.Stdout, data) if err != nil { panic(err) }
When you execute this code, it will replace {{.Name}} with “John” and output the following HTML:
html <html><body><h1>Hello, John!</h1></body></html>
This simple example demonstrates the basic process of creating and rendering templates using the html/template package. However, Go’s template engine is capable of handling much more complex scenarios, making it a valuable tool for web developers.
3. Template Actions and Control Structures
In addition to simple placeholders like {{.Name}}, Go’s html/template package provides a set of template actions and control structures that enable you to create dynamic content efficiently. These actions are enclosed in double curly braces, just like placeholders, but they begin with a dollar sign ($).
3.1. Output Actions
Output actions are used to insert dynamic values into the template. Here are some common output actions:
- {{.Field}}: Inserts the value of the specified field from the data.
- {{.Field.Method}}: Calls a method on the specified field and inserts the result.
- {{.Field.Field2}}: Chains multiple fields together.
go const templateString = "<p>Name: {{.Name}}</p><p>Age: {{.Age}}</p>"
In this example, we use output actions to insert the Name and Age fields from the data into the HTML template.
3.2. Control Structures
Control structures allow you to add logic and conditional statements to your templates. Here are some common control structures:
- {{if .Condition}} … {{end}}: Conditionally includes content based on a boolean condition.
- {{range .Slice}} … {{end}}: Iterates over a slice and executes the inner template for each element.
- {{with .Field}} … {{end}}: Sets a temporary context and executes the inner template with that context.
go const templateString = ` {{if .IsAdmin}} <p>Welcome, Administrator!</p> {{else}} <p>Welcome, User!</p> {{end}}
In this example, the template displays a different message based on the value of the IsAdmin field in the data.
3.3. Functions
Go’s html/template package also allows you to define and use custom functions within your templates. You can register functions using the Funcs method of the template object and then call them within the template.
go func add(a, b int) int { return a + b } t := template.New("math").Funcs(template.FuncMap{ "add": add, }) const templateString = "{{add .Value1 .Value2}}"
In this example, we define a custom function add that adds two integers. We then register this function with the template and use it to calculate the sum of Value1 and Value2.
4. Data Injection and Context
In Go’s html/template package, data is provided to templates as a structured Go value. This data can be a simple struct, a map, or any other Go type. To inject data into a template, you pass the data as an argument when executing the template.
4.1. Structs as Data
Using a struct as your data container is a common and recommended practice in Go’s templating system. It allows you to define the structure of the data explicitly, which makes the code more readable and maintainable.
go type Person struct { Name string Age int } data := Person{"Alice", 30}
In this example, we define a Person struct to represent the data we want to inject into the template. We then create an instance of the Person struct and populate it with values.
4.2. Maps as Data
Alternatively, you can use maps to provide data to templates. While maps offer flexibility, they don’t provide the same level of type safety as structs.
go data := map[string]interface{}{ "Name": "Bob", "Age": 25, }
In this example, we create a map with string keys and interface{} values to hold our data. The keys in the map correspond to the placeholders and actions in the template.
4.3. Context and Nested Data
Templates in Go have access to the fields of the data structure directly, but sometimes it’s useful to work with nested data or temporary contexts. The with action allows you to set a temporary context and execute a template with that context.
go const templateString = ` {{with .Author}} <p>Name: {{.Name}}</p> <p>Age: {{.Age}}</p> {{end}} `
In this example, the with action sets the context to the Author field within the data and executes the inner template with that context. This can be helpful when dealing with complex data structures.
5. Escaping and Security
Security is a paramount concern in web development. Go’s html/template package was designed with security in mind and provides automatic HTML escaping by default. This means that any data inserted into a template is automatically escaped to prevent Cross-Site Scripting (XSS) attacks.
For example, if you have the following data:
go data := struct{ Name string }{"<script>alert('XSS')</script>"}
And you insert this data into your template:
go const templateString = "<p>{{.Name}}</p>"
The generated HTML will look like this:
html <p><script>alert('XSS')</script></p>
The < and > characters, as well as the single quotes, have been escaped to their HTML entities, ensuring that any potential JavaScript code is treated as plain text.
6. Creating Reusable Templates
In real-world web applications, you’ll often need to reuse templates across multiple pages or sections of your site. Go’s html/template package supports template composition, allowing you to create reusable components and assemble them into larger templates.
6.1. Template Inclusion
You can include one template within another using the {{template}} action. This action inserts the content of the specified template into the current template at the specified location.
go const headerTemplate = "<header><h1>My Website</h1></header>" const contentTemplate = "<div>{{template \"header\"}}</div>"
In this example, we have a headerTemplate that defines the website header and a contentTemplate that includes the header using the {{template}} action. This allows you to maintain a consistent header across multiple pages.
6.2. Template Blocks
Another way to create reusable components is by defining template blocks. Blocks are sections of a template that can be replaced with custom content when the template is executed.
go const baseTemplate = ` <html> <head> <title>{{block "title"}}Default Title{{end}}</title> </head> <body> {{block "content"}}Default Content{{end}} </body> </html> `
In this example, the baseTemplate defines two blocks: “title” and “content”. When you execute this template, you can provide custom content for these blocks using the {{define}} action.
go const pageTemplate = ` {{template "base" .}} {{define "title"}}Page Title{{end}} {{define "content"}}Page Content{{end}}
Here, the pageTemplate includes the baseTemplate using the {{template}} action and defines custom content for the “title” and “content” blocks using the {{define}} action.
When you execute the pageTemplate, it will replace the blocks in the baseTemplate with the custom content you’ve defined, resulting in a complete HTML page.
7. Advanced Topics and Best Practices
While we’ve covered the basics of Go’s html/template package, there are many advanced features and best practices to explore. Here are some topics to delve into as you become more proficient with template-based web development in Go:
- Template Composition: Learn how to create modular templates and compose them to build complex web pages efficiently.
- Custom Functions: Explore the creation of custom functions to extend the template engine’s capabilities.
- Template Caching: Understand how to cache and reuse templates to improve performance.
- Security Considerations: Study best practices for handling user-generated content and preventing security vulnerabilities.
- Error Handling: Implement robust error handling strategies when working with templates, including error reporting and debugging.
- Benchmarking: Use benchmarking to measure the performance of your templates and identify optimization opportunities.
Conclusion
Go’s template engines, particularly the html/template package, provide a powerful and secure way to generate dynamic content for web applications. By separating the template logic from the data, you can create maintainable and secure web applications that are less prone to common web vulnerabilities. With control structures, custom functions, and template composition, Go’s templating system offers the flexibility and expressiveness needed for building modern web applications.
As you continue to explore and master Go’s templating capabilities, you’ll find that it’s a valuable tool in your web development toolkit, enabling you to create dynamic and engaging user interfaces with ease. Whether you’re building a simple website or a complex web application, Go’s template engines have you covered, delivering performance and security in equal measure. Happy templating!
Table of Contents
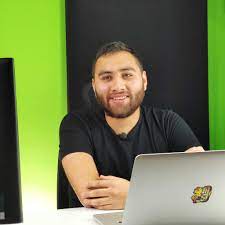
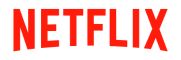