How do you work with time and dates in Go?
Working with time and dates in Go is straightforward and powerful, thanks to the built-in time package. The time package provides functionalities for representing and manipulating time, durations, and dates in Go programs.
At the core of the time package are two fundamental types: Time and Duration. The Time type represents an instant in time, while Duration represents a length of time. You can create Time objects using various constructors provided by the package, such as time.Now() to get the current time or time.Date() to specify a specific date and time.
Once you have a Time object, you can perform various operations like formatting, comparison, addition, subtraction, and extraction of individual components such as year, month, day, hour, minute, second, and nanosecond.
go package main import ( "fmt" "time" ) func main() { // Get the current time now := time.Now() fmt.Println("Current time:", now) // Create a specific date and time date := time.Date(2023, time.March, 8, 12, 30, 0, 0, time.UTC) fmt.Println("Specific date:", date) // Format time as string fmt.Println("Formatted time:", now.Format("2006-01-02 15:04:05")) // Add 2 hours to the current time futureTime := now.Add(2 * time.Hour) fmt.Println("Future time:", futureTime) // Calculate the duration between two times duration := futureTime.Sub(now) fmt.Println("Duration:", duration) }
Go’s time package also offers support for parsing time strings, performing timezone conversions, and calculating differences between time values accurately.
Furthermore, the time package provides a rich set of functions and methods for working with timezones, formatting and parsing time strings, and performing arithmetic operations on time values. With these capabilities, Go makes it easy to handle a wide range of time-related tasks in a reliable and efficient manner.
The time package in Go provides a comprehensive set of tools for working with time and dates, enabling developers to build robust and reliable applications that handle time-sensitive operations effectively.
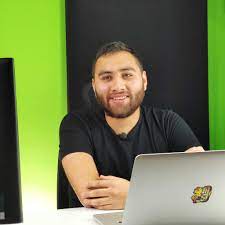
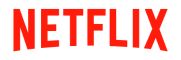