How do you write and execute unit tests in Go?
Writing and executing unit tests in Go is a straightforward process thanks to the built-in testing framework provided by the testing package. To write unit tests in Go, follow these steps:
Create a Test File: Create a new Go source file with a name ending in _test.go. This convention signals to the Go compiler that the file contains test code.
Write Test Functions: Define one or more test functions in the test file. Test functions must have names starting with Test followed by the name of the function or method being tested and accept a single parameter of type *testing.T, which is used to report test failures and errors.
Use Test Helpers: Utilize helper functions provided by the testing package, such as t.Errorf and t.Fatalf, to report test failures and errors.
Run the Tests: To execute the tests, use the go test command in the terminal. This command automatically discovers and runs all test functions in files ending with _test.go in the current directory and its subdirectories.
Here’s an example illustrating the process:
go // math_test.go package math import "testing" func Add(a, b int) int { return a + b } func TestAdd(t *testing.T) { result := Add(2, 3) expected := 5 if result != expected { t.Errorf("Add(2, 3) returned %d, expected %d", result, expected) } }
In this example, we define a simple function Add for adding two integers and a corresponding test function TestAdd to verify its correctness. We use the testing.T type provided by the testing package to report any test failures or errors.
To execute the tests, navigate to the directory containing the test file (math_test.go) in the terminal and run the following command:
bash go test
The go test command compiles the test code, executes the tests, and reports the results. It indicates the number of tests executed, the number of failures, and any error messages encountered during the testing process.
By following these steps, you can effectively write and execute unit tests for your Go code, ensuring its correctness, reliability, and maintainability throughout the development process.
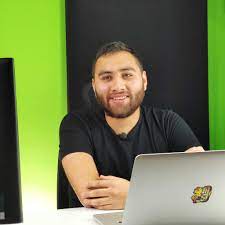
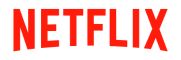