Exploring iOS App Security: Best Practices and Vulnerabilities
In today’s digital age, mobile applications play a pivotal role in our lives, from banking and shopping to social interactions and entertainment. As the demand for feature-rich and user-friendly iOS apps continues to grow, ensuring the security of these applications has become a paramount concern. With sensitive user data and confidential information being exchanged within these apps, it’s crucial for developers to understand the best practices and potential vulnerabilities that can compromise their users’ security. In this article, we’ll delve into the realm of iOS app security, exploring both the recommended practices to follow and the common vulnerabilities to watch out for.
1. Understanding iOS App Security
Securing an iOS app involves a multi-layered approach, ranging from the coding phase to the deployment and ongoing maintenance. Let’s break down the key areas to focus on:
1.1. Secure Code Development
Writing secure code is the foundation of building a robust iOS app. Utilize the following best practices during the development process:
1.1.1 Input Validation
Always validate user inputs to prevent injection attacks like SQL injection and cross-site scripting. Use frameworks like NSRegularExpression to define strict input patterns and avoid processing potentially malicious data.
swift if let range = input.range(of: "^[A-Za-z]+$", options: .regularExpression) { // Valid input } else { // Invalid input }
1.1.2 Avoid Hardcoding Secrets
Never hardcode sensitive information such as API keys, passwords, or tokens directly into the source code. Instead, store them securely in the Keychain or use environment variables.
swift import Foundation guard let apiKey = ProcessInfo.processInfo.environment["API_KEY"] else { fatalError("API key not found") }
1.2. Secure Data Storage
Protecting user data is of utmost importance. When storing data locally, follow these practices:
1.2.1 Use the Keychain
Store sensitive data such as passwords and authentication tokens in the Keychain, which provides a secure and encrypted storage mechanism.
swift import Security let keychainQuery: [CFString: Any] = [ kSecClass: kSecClassGenericPassword, kSecAttrService: "MyApp", kSecAttrAccount: "User123", kSecValueData: passwordData ] let status = SecItemAdd(keychainQuery as CFDictionary, nil)
1.2.2 Data Encryption
When saving sensitive data in files, encrypt the data using algorithms like AES before storing it on the device.
swift import CryptoKit let dataToEncrypt = Data("SensitiveData".utf8) let encryptionKey = SymmetricKey(size: .bits256) let encryptedData = try! AES.GCM.seal(dataToEncrypt, using: encryptionKey).combined
1.3. Network Communication
Secure communication between your app and servers is crucial to prevent eavesdropping and man-in-the-middle attacks.
1.3.1 Use HTTPS
Always communicate with servers over HTTPS to encrypt data transmitted between the app and the server.
swift import Foundation let url = URL(string: "https://api.example.com/data")! let task = URLSession.shared.dataTask(with: url) { data, response, error in // Handle response } task.resume()
1.3.2 Certificate Pinning
Implement certificate pinning to ensure that your app only communicates with servers having valid and trusted SSL certificates.
swift import Foundation class URLSessionDelegate: NSObject, URLSessionDelegate { func urlSession(_ session: URLSession, didReceive challenge: URLAuthenticationChallenge, completionHandler: @escaping (URLSession.AuthChallengeDisposition, URLCredential?) -> Void) { if challenge.protectionSpace.authenticationMethod == NSURLAuthenticationMethodServerTrust { // Implement certificate pinning logic } } } let sessionDelegate = URLSessionDelegate() let session = URLSession(configuration: .default, delegate: sessionDelegate, delegateQueue: nil)
1.4. Code Obfuscation
Make reverse engineering difficult by using code obfuscation techniques. This prevents attackers from easily understanding your app’s logic and vulnerabilities.
1.4.1 ProGuard for Swift
Use tools like ProGuard for Swift to rename symbols, remove unused code, and optimize the binary.
1.4.2 String Encryption
Encrypt strings in your code to make it harder for attackers to extract sensitive information.
swift extension String { func decrypted() -> String { // Implement decryption logic } }
2. Common iOS App Vulnerabilities
Despite taking preventive measures, iOS apps are still susceptible to various vulnerabilities. Let’s explore some of the common ones:
2.1. Insecure Data Storage
Storing sensitive data in plain text or using weak encryption methods can lead to data leaks if an attacker gains access to the device.
2.2. Inadequate Jailbreak Detection
Jailbroken devices can expose your app to increased security risks. Failing to detect and respond to a jailbroken environment can compromise your app’s security.
2.3. Improper Session Management
Weak session management can result in unauthorized access to user accounts. Always use secure session tokens, implement proper logout mechanisms, and handle token expiration gracefully.
2.4. Lack of Input Validation
Insufficient input validation can open doors to various attacks, including injection attacks and cross-site scripting. Always validate and sanitize user inputs.
2.5. Insecure APIs
Exposed or poorly protected APIs can be exploited to gain unauthorized access or perform actions on behalf of users. Implement strong authentication and authorization mechanisms.
2.6. Insecure Third-party Libraries
Using outdated or vulnerable third-party libraries can introduce security flaws into your app. Regularly update and audit the libraries you depend on.
Conclusion
Creating a secure iOS app involves a combination of following best practices during development and staying vigilant against potential vulnerabilities. By understanding the security landscape and incorporating measures to protect user data, you can build apps that users can trust and rely on. Remember, security is an ongoing process that requires continuous monitoring, updates, and adaptation to the evolving threat landscape. Prioritize security in every phase of your iOS app development lifecycle to provide a safe and secure experience for your users.
Table of Contents
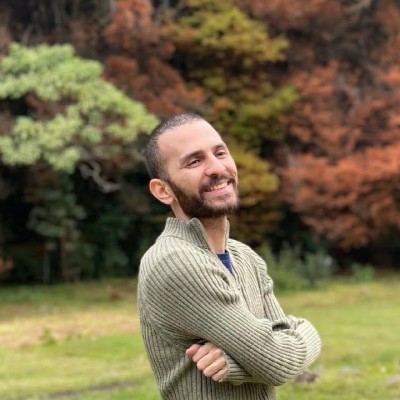
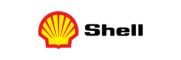