iOS Q & A
How do I perform asynchronous tasks in Swift?
Asynchronous programming is essential in Swift for performing tasks that may take time to complete, such as network requests, file I/O, or computation-intensive operations, without blocking the main thread and causing the app to become unresponsive. Here’s how you can perform asynchronous tasks in Swift:
- Completion Handlers: Use completion handlers to execute code asynchronously when a task completes. Define a closure parameter in your function that accepts the result of the asynchronous task and invoke the closure when the task finishes.
swift func fetchData(completion: @escaping (Result<Data, Error>) -> Void) { // Perform asynchronous task DispatchQueue.global().async { // Simulate fetching data if let data = fetchDataFromServer() { completion(.success(data)) } else { completion(.failure(NetworkError())) } } }
- Dispatch Queues: Use Grand Central Dispatch (GCD) to perform tasks asynchronously on background threads. Dispatch work items or closures to a background dispatch queue using DispatchQueue.global().async.
swift DispatchQueue.global().async { // Perform asynchronous task fetchDataFromServer() }
- Async/Await: Use async/await syntax introduced in Swift 5.5 and later to perform asynchronous tasks in a more concise and readable manner. Mark asynchronous functions with the async keyword and use await to suspend execution until the asynchronous operation completes.
swift func fetchData() async throws -> Data { // Perform asynchronous task let data = try await fetchDataFromServer() return data }
- Combine Framework: Use the Combine framework to work with asynchronous data streams and handle asynchronous operations using publishers, subscribers, and operators. Combine provides a declarative and functional approach to asynchronous programming in Swift.
swift import Combine func fetchData() -> AnyPublisher<Data, Error> { // Perform asynchronous task return URLSession.shared.dataTaskPublisher(for: URL(string: "https://example.com/data")!) .map(\.data) .eraseToAnyPublisher() }
By using these techniques, you can perform asynchronous tasks in Swift effectively, ensuring that your app remains responsive and performs efficiently even when executing time-consuming operations.
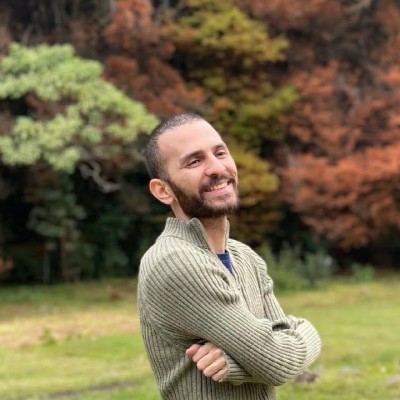
Previously at
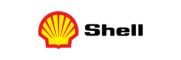
Skilled iOS Engineer with extensive experience developing cutting-edge mobile solutions. Over 7 years in iOS development.