Building Data Visualizations in iOS: Charts and Graphs
In the world of iOS app development, data visualization is a crucial component for conveying information effectively and engaging users. Whether you’re building a fitness app tracking workout progress, a finance app displaying stock market trends, or a weather app showcasing temperature fluctuations, charts and graphs are indispensable tools. In this blog, we’ll explore how to create stunning data visualizations in iOS using popular libraries like Charts and Core Plot. You’ll find code samples, step-by-step guides, and best practices to help you build informative and engaging visuals for your app.
Table of Contents
1. Why Data Visualizations Matter
Before diving into the technical aspects of building data visualizations, let’s briefly discuss why they are essential in iOS app development.
1.1. Enhanced User Engagement
Users are drawn to visuals. Data visualizations make your app more engaging and user-friendly, helping users quickly grasp complex information.
1.2. Improved Data Understanding
Charts and graphs simplify data interpretation, allowing users to make informed decisions based on your app’s data.
1.3. Increased Retention
Apps that effectively communicate data tend to retain users for longer periods, contributing to your app’s success.
2. Choosing the Right Library
To create data visualizations in iOS, you have several libraries to choose from. Two of the most popular options are “Charts” and “Core Plot.” Let’s explore the features and benefits of each to help you make an informed decision.
2.1. Charts Library
Charts is a widely-used iOS library for creating interactive charts and graphs. It offers a variety of chart types, including line charts, bar charts, pie charts, and more. Here are some key advantages of using Charts:
2.2. Easy to Use
Charts provides a straightforward API, making it accessible to both beginners and experienced developers.
2.3. Rich Customization
You can customize the appearance of your charts extensively, including colors, fonts, and animations.
2.4. Interactive
Charts supports interactive gestures like tapping and zooming, enhancing the user experience.
2.5. Data Integration
It seamlessly integrates with Core Data, making it a powerful choice for data-driven apps.
2.6. Community Support
The Charts library has an active community, ensuring you can find help and resources easily.
2.7. Core Plot Library
Core Plot is another powerful library for creating data visualizations in iOS. While it offers more flexibility, it may require a steeper learning curve. Here are some advantages of using Core Plot:
2.8. Highly Customizable
Core Plot allows for fine-grained customization, making it suitable for complex charting requirements.
2.9. Versatile
You can create a wide range of chart types, from basic line and bar charts to complex financial charts.
2.10. No External Dependencies
Core Plot does not rely on external libraries, reducing potential conflicts with other dependencies.
2.11. Open Source
Being open-source, Core Plot is continually improved and maintained by the community.
3. Setting Up Your Development Environment
Now that you’ve chosen your library, let’s set up your development environment to get started with building data visualizations. We’ll use the Charts library as an example.
3.1. Create a New Xcode Project
Start by creating a new Xcode project or opening an existing one where you want to integrate data visualizations.
3.2. Install Charts via CocoaPods
To integrate the Charts library into your project, you can use CocoaPods, a popular dependency manager for iOS. If you haven’t installed CocoaPods yet, run the following command in your terminal:
gem install cocoapods
Then, navigate to your project directory and create a Podfile if you don’t have one already:
csharp pod init
Edit your Podfile to include Charts:
ruby target 'YourApp' do use_frameworks! pod 'Charts' end
Save the file and install the dependencies by running:
pod install
Finally, open the .xcworkspace file generated by CocoaPods.
3.3. Import Charts in Your View Controller
In your view controller where you want to display the chart, import the Charts library:
swift import Charts
3.4. Create a ChartView
Add a UIView to your storyboard or create it programmatically in your view controller’s view. Change the class of this view to BarChartView or the type of chart you want to use (e.g., LineChartView, PieChartView).
swift @IBOutlet weak var chartView: BarChartView!
Connect this IBOutlet to your view controller’s storyboard.
3.5. Configure Your Chart
In your view controller’s viewDidLoad method, configure your chart view:
swift override func viewDidLoad() { super.viewDidLoad() configureChart() } func configureChart() { // Configure chart properties here }
4. Creating Your First Chart
With the development environment set up, it’s time to create your first chart. We’ll start with a simple bar chart as an example.
4.1. Providing Data
To populate your chart with data, create an array of BarChartDataEntry objects. Each entry represents a data point on the chart. For instance, if you’re creating a bar chart displaying monthly revenue, each entry would represent revenue for a specific month.
swift let dataEntries: [BarChartDataEntry] = [ BarChartDataEntry(x: 1.0, y: 2000.0), BarChartDataEntry(x: 2.0, y: 2500.0), BarChartDataEntry(x: 3.0, y: 1800.0), // Add more entries here ]
4.2. Creating a DataSet
Next, create a BarChartDataSet by providing your data entries and a label for the dataset. You can customize the appearance of the bars, such as colors and bar width, through the dataset.
swift let dataSet = BarChartDataSet(entries: dataEntries, label: "Monthly Revenue") dataSet.colors = ChartColorTemplates.joyful() dataSet.drawValuesEnabled = false // Disable value labels on bars
4.3. Creating a Data Object
Combine your dataset into a BarChartData object:
swift let data = BarChartData(dataSet: dataSet)
4.4. Setting the Chart Data
Finally, assign the data object to your chart view:
swift chartView.data = data
Your bar chart is now ready to be displayed in your app.
5. Customizing Your Chart
One of the key advantages of using the Charts library is its flexibility in customization. You can tailor your chart’s appearance to match your app’s design and style.
5.1. Chart Appearance
Customize the appearance of your chart by modifying properties like background color, border color, and chart description:
swift chartView.backgroundColor = .white chartView.borderColor = .gray chartView.borderLineWidth = 1.0 chartView.chartDescription?.text = "Monthly Revenue Chart"
5.2. Axis Configuration
You can configure the x-axis and y-axis according to your data:
swift chartView.xAxis.labelPosition = .bottom chartView.xAxis.labelFont = UIFont.systemFont(ofSize: 12.0) chartView.xAxis.valueFormatter = IndexAxisValueFormatter(values: ["Jan", "Feb", "Mar", "Apr"]) // Customize labels chartView.leftAxis.labelFont = UIFont.systemFont(ofSize: 12.0) chartView.rightAxis.enabled = false // Disable the right y-axis
5.3. Legend
Customize the legend, which provides information about the dataset:
swift chartView.legend.enabled = true chartView.legend.font = UIFont.systemFont(ofSize: 12.0)
5.4. Animation
Add animations to your chart to make it more engaging:
swift chartView.animate(xAxisDuration: 1.0, yAxisDuration: 1.0, easingOption: .linear)
6. Handling User Interactions
Interactive charts enhance user engagement and understanding. You can implement various gestures and interactions with the Charts library.
6.1. Tapping on Data Points
To respond to user taps on data points, conform your view controller to the ChartViewDelegate protocol and implement the chartValueSelected(_ chartView: ChartViewBase, entry: ChartDataEntry, highlight: Highlight) method:
swift func chartValueSelected(_ chartView: ChartViewBase, entry: ChartDataEntry, highlight: Highlight) { // Handle the selection of a data point here }
6.2. Zooming
Enable zooming by setting the scaleXEnabled and scaleYEnabled properties to true for your chart view:
swift chartView.scaleXEnabled = true chartView.scaleYEnabled = true
6.3. Highlighting Values
You can highlight specific values programmatically by using the highlightValue(x: y: dataSetIndex: dataIndex:) method:
swift chartView.highlightValue(x: 2.0, dataSetIndex: 0, dataIndex: 1)
7. Real-World Example: Financial Chart
Let’s dive into a more complex example by creating a financial chart using Core Plot. This example will demonstrate how to use Core Plot’s flexibility for custom charting needs.
7.1. Install Core Plot via CocoaPods
Just like with Charts, you can use CocoaPods to integrate Core Plot into your project. Add the following line to your Podfile:
ruby pod 'CorePlot'
Then, run pod install and open the .xcworkspace file.
7.2. Import Core Plot in Your View Controller
Import Core Plot in the view controller where you want to display the financial chart:
swift import CorePlot
7.3. Create a Financial ChartView
Create a Core Plot CPTGraphHostingView and add it to your view controller’s view:
swift let hostingView = CPTGraphHostingView() hostingView.frame = CGRect(x: 0, y: 0, width: view.frame.width, height: view.frame.height) view.addSubview(hostingView)
7.4. Configure the Chart
Configure your financial chart with axes, plot spaces, and data sources:
swift let graph = CPTXYGraph(frame: hostingView.bounds) hostingView.hostedGraph = graph let plotSpace = graph.defaultPlotSpace as? CPTXYPlotSpace // Configure plot space and axes here // …
7.5. Provide Data
Implement the data source methods to provide data for your financial chart:
swift func numberOfRecords(for plot: CPTPlot) -> UInt { // Return the number of data points } func number(for plot: CPTPlot, field: UInt, recordIndex: UInt) -> Any? { // Return data points for each field (x or y) }
7.6. Customize Appearance
Customize the appearance of your financial chart by setting properties like line styles, colors, and labels:
swift let lineStyle = CPTMutableLineStyle() lineStyle.lineColor = CPTColor.blue() lineStyle.lineWidth = 2.0 // Apply line styles and labels to your plot // ...
7.7. Add Interactivity
Implement interaction features, such as zooming and panning, to enhance user experience:
swift graph.plotAreaFrame?.masksToBorder = false graph.plotAreaFrame?.paddingBottom = 30.0 graph.plotAreaFrame?.paddingLeft = 30.0 graph.plotAreaFrame?.paddingRight = 10.0 graph.plotAreaFrame?.paddingTop = 20.0 // Enable user interactions like scrolling, zooming, and panning // ...
Conclusion
Data visualizations are indispensable in modern iOS app development. They not only enhance user engagement but also help users better understand and interpret data. In this blog, we’ve explored two powerful libraries, Charts and Core Plot, for creating stunning data visualizations in your iOS apps. You’ve learned how to set up your development environment, create various types of charts, customize their appearance, and implement user interactions.
Now it’s time to dive into your own app project and start building informative and engaging data visualizations that will set your iOS app apart from the competition. Whether you choose Charts for its simplicity or Core Plot for its flexibility, you have the tools you need to create impressive data visualizations that will captivate your users. Happy coding!
Table of Contents
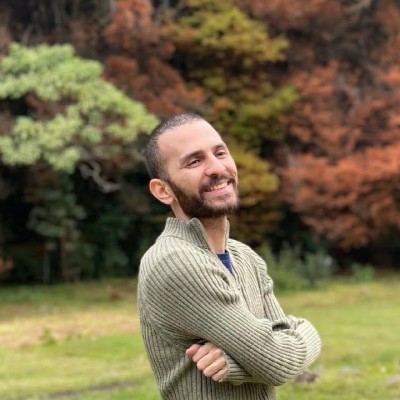
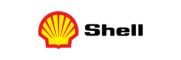