Making the Most of Core Location in iOS: Building Location-Aware Apps
In the ever-evolving landscape of mobile applications, building location-aware apps has become an essential aspect of enhancing user experiences. Whether it’s finding nearby restaurants, tracking fitness activities, or providing turn-by-turn directions, leveraging accurate and reliable location data is crucial. This is where Core Location, a powerful framework provided by Apple, comes into play. In this blog post, we’ll delve into the world of Core Location and explore how to create exceptional location-aware iOS apps. We’ll cover essential concepts, best practices, and provide you with hands-on code samples to kickstart your journey.
1. Understanding Core Location: The Heart of Location Services
1.1 What is Core Location?
At its core, Core Location is a framework that allows iOS apps to gather location-based information from various sources, such as GPS, Wi-Fi, and cellular networks. This framework empowers developers to access precise geographic coordinates, altitude, course, and speed of a device.
1.2 Location Services Permissions: Respecting User Privacy
Before diving into the technical details, it’s important to highlight user privacy. Apple emphasizes user control over their data, and location services are no exception. To access location data, apps must request the appropriate permissions from the user. Always ensure you explain why your app needs location data and provide the user with the option to grant or deny access.
2. Building Blocks of Location Services:
2.1. CLLocationManager: Managing Location Updates
The CLLocationManager class is your gateway to location services. It facilitates the interaction between your app and the system’s location hardware. Through CLLocationManager, you can request various levels of location accuracy and manage updates at different intervals. Let’s see how you can set up and use CLLocationManager:
swift import CoreLocation class LocationManager: NSObject, CLLocationManagerDelegate { private let locationManager = CLLocationManager() override init() { super.init() locationManager.delegate = self locationManager.requestWhenInUseAuthorization() // Request permission } func startUpdatingLocation() { locationManager.startUpdatingLocation() } // Implement CLLocationManagerDelegate methods here }
2.2. Location Accuracy: Precision Matters
Location accuracy is a key factor in providing a seamless user experience. Core Location offers various accuracy levels, such as “Best for Navigation,” “High Accuracy,” and “Reduced Accuracy.” Depending on your app’s requirements, you can select the appropriate accuracy level to balance battery consumption and precision.
2.3. Geocoding and Reverse Geocoding
Converting between geographic coordinates and user-readable addresses is made possible by geocoding and reverse geocoding. The former translates addresses to coordinates, while the latter transforms coordinates into human-readable addresses. Here’s a glimpse of how to perform geocoding using CLGeocoder:
swift let geocoder = CLGeocoder() geocoder.geocodeAddressString("Apple Infinite Loop") { placemarks, error in if let placemark = placemarks?.first { print(placemark.location?.coordinate) } }
3. Best Practices for Exceptional Location-Aware Apps:
3.1. Battery Optimization: Efficiency is Key
Frequent location updates can drain a device’s battery quickly. To optimize battery usage, utilize the desiredAccuracy property of CLLocationManager to request location updates only when the required accuracy is achieved. Additionally, use stopUpdatingLocation() when updates are no longer needed.
3.2. Handling Location Authorization Changes
Users can change location permissions at any time. Handle authorization changes gracefully by implementing the CLLocationManagerDelegate methods like locationManager(_:didChangeAuthorization:). Update your app’s behavior accordingly to ensure a seamless user experience.
3.3. Background Location Updates
Some apps need to track location even when they’re in the background. While this can be a powerful feature, it should be used judiciously due to battery concerns. To enable background location updates, set the allowsBackgroundLocationUpdates property of CLLocationManager to true.
4. Putting It All Together: Building a Location-Aware Weather App
Step 1: Requesting Location Permissions
swift locationManager.requestWhenInUseAuthorization()
Step 2: Handling Location Updates
swift func startUpdatingLocation() { locationManager.startUpdatingLocation() } func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) { if let location = locations.last { // Update UI with new location data } }
Step 3: Displaying Current Weather
swift func fetchWeatherForLocation(_ location: CLLocation) { // Use the location's coordinates to fetch weather data from an API }
Step 4: Geocoding for User-Friendly Display
swift func updateLocationLabel(using placemark: CLPlacemark) { let address = "\(placemark.locality ?? ""), \(placemark.country ?? "")" locationLabel.text = address }
Conclusion
Core Location is a treasure trove for developers seeking to create location-aware iOS apps that deliver value and convenience to users. By mastering the capabilities of the CLLocationManager class, understanding location accuracy, and implementing best practices, you can build apps that seamlessly integrate with users’ lives. Whether you’re guiding them through unknown streets or helping them discover hidden gems in their vicinity, the power of Core Location is at your fingertips. So, dive in, explore its features, and craft remarkable location experiences that elevate your app’s functionality to new heights. Happy coding!
Table of Contents
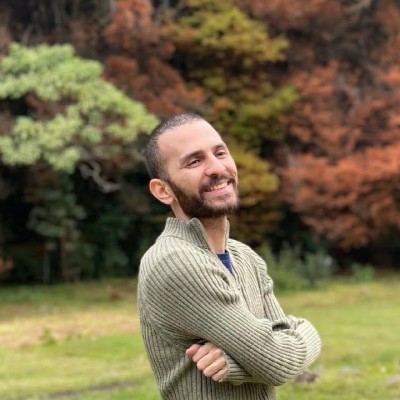
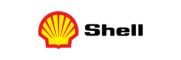