How do I create custom delegates in Swift?
Creating custom delegates in Swift involves several steps to define a protocol, establish the delegate property, and implement the delegate methods. Here’s a step-by-step guide on how to create custom delegates in Swift:
- Define a Protocol: Begin by defining a protocol that declares the methods or properties that the delegate may implement. Protocols define the interface that the delegate must conform to. For example:
swift protocol CustomDelegate: AnyObject { func didReceiveData(_ data: String) }
- Declare a Delegate Property: In the class or structure that requires a delegate, declare a weak or unowned property to hold a reference to the delegate object. It’s common to make the delegate property optional to handle scenarios where no delegate is set.
swift class MyClass { weak var delegate: CustomDelegate? }
- Invoke Delegate Methods: Within the class or structure, invoke the delegate methods as needed. Typically, these methods are called to notify the delegate of specific events or pass data back to the delegate.
swift class MyClass { weak var delegate: CustomDelegate? func fetchData() { // After fetching data, notify the delegate delegate?.didReceiveData("Example data") } }
- Implement the Delegate: Conform to the protocol in a separate class or structure and implement the required delegate methods. This class or structure becomes the delegate and is responsible for responding to the delegate callbacks.
swift class MyDelegate: CustomDelegate { func didReceiveData(_ data: String) { print("Received data: \(data)") } }
- Assign the Delegate: Finally, assign an instance of the delegate class or structure to the delegate property of the delegating object.
swift let myObject = MyClass() let myDelegate = MyDelegate() myObject.delegate = myDelegate
Now, when fetchData() is called on myObject, the didReceiveData(_:) method implemented in MyDelegate will be invoked, allowing it to handle the received data.
Custom delegates provide a flexible and powerful mechanism for communication between objects in Swift. They enable loose coupling between components, allowing for better separation of concerns and modular design. By following these steps, you can create and use custom delegates in your Swift projects to facilitate communication between objects in a type-safe and protocol-oriented manner.
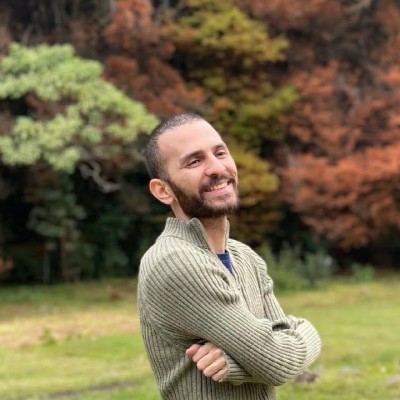
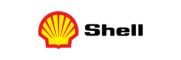