Debugging and Testing in iOS: Essential Tools and Techniques
Creating robust and reliable iOS applications requires a comprehensive approach to debugging and testing. The process of identifying and rectifying bugs and issues within your codebase is an essential aspect of software development. In this blog post, we will delve into the crucial tools and techniques that can aid you in ensuring the quality and stability of your iOS apps.
1. The Importance of Debugging and Testing
Debugging and testing form the backbone of the software development lifecycle. They are pivotal in identifying issues early in the development process, saving time and effort in the long run. Effective debugging and testing lead to improved app performance, enhanced user experience, and higher user satisfaction.
2. Common Bugs in iOS Development
Before diving into the debugging and testing tools and techniques, let’s take a look at some common types of bugs that often arise during iOS development:
- Crashes: These are abrupt terminations of an application. They can occur due to memory issues, null pointer dereferences, or unhandled exceptions.
- Memory Leaks: Memory leaks happen when an application fails to release memory that is no longer in use. Over time, this can lead to decreased app performance and even crashes.
- UI Glitches: User Interface glitches include misaligned elements, unresponsive controls, or layout problems across different devices.
- Networking Errors: Issues with network requests can result in incomplete data loading, slow app performance, or even app crashes.
- Compatibility Problems: Apps may work well on one iOS version but exhibit unexpected behavior on another due to compatibility issues.
3. Essential Debugging Tools
3.1. Xcode Debugger
Xcode, the primary Integrated Development Environment (IDE) for iOS development, comes equipped with a powerful debugger. It allows you to set breakpoints, inspect variables, and step through your code line by line.
swift func calculateTotalPrice() -> Double { let basePrice = 20.0 let taxRate = 0.1 let totalPrice = basePrice / (1 - taxRate) return totalPrice }
In the above code snippet, if we suspect an issue with the totalPrice calculation, we can set a breakpoint at the return statement and use Xcode’s debugger to examine the values of basePrice, taxRate, and totalPrice.
3.2. print() Statements
Strategically placed print() statements can provide valuable insights into the flow of your application and the values of variables at different points. This is especially useful when debugging code that doesn’t involve a graphical interface, like backend logic.
swift func fetchData() { print("Fetching data...") // Code to fetch data print("Data fetched successfully!") }
Print statements help track the progress of your code and identify any unexpected behaviors.
3. Effective Testing Techniques
3.1. Unit Testing
Unit testing involves testing individual units or components of your application in isolation. Xcode provides a built-in unit testing framework that enables you to write test cases for your methods and functions.
swift func divide(_ a: Int, _ b: Int) -> Int { return a / b } func testDivide() { XCTAssertEqual(divide(10, 2), 5) XCTAssertEqual(divide(8, 0), 0) // This test will fail, highlighting an issue }
Unit tests help catch bugs early by ensuring that individual components of your app function as expected.
3.2. UI Testing
UI testing involves automating interactions with the app’s user interface to ensure that UI elements and flows work correctly. Xcode’s UI testing framework lets you write scripts to simulate user actions.
swift func testLogin() { let app = XCUIApplication() app.launch() let usernameField = app.textFields["username"] let passwordField = app.secureTextFields["password"] let loginButton = app.buttons["loginButton"] usernameField.tap() usernameField.typeText("user@example.com") passwordField.tap() passwordField.typeText("pass123") loginButton.tap() XCTAssertTrue(app.otherElements["homeScreen"].exists) }
UI testing ensures that your app’s interface behaves as intended, preventing regressions in user experience.
4. Debugging Best Practices
- Isolate the Issue: Narrow down the problem’s scope by identifying the exact conditions under which it occurs. This can significantly speed up the debugging process.
- Use Version Control: Regularly commit your code and use version control tools like Git. This allows you to revert to a working version if debugging attempts lead to more issues.
- Check Documentation: Always consult official documentation for libraries and frameworks you’re using. The issue you’re facing might be a known one with a documented solution.
5. Testing Best Practices
- Test Early and Often: Start testing your code as soon as possible in the development process. The earlier you catch a bug, the easier and cheaper it is to fix.
- Automate Tests: Automating tests, especially unit and UI tests, saves time and ensures consistency. Continuous Integration (CI) tools can automatically run tests with each code change.
- Edge Cases: Ensure that your tests cover a variety of scenarios, including edge cases and unexpected inputs.
Conclusion
Debugging and testing are indispensable aspects of iOS app development that contribute to the creation of high-quality applications. Utilizing tools like Xcode’s debugger, writing unit and UI tests, and following best practices will aid in identifying and resolving issues efficiently. By integrating these techniques into your development workflow, you can ensure a smooth user experience and the success of your iOS applications. Remember, a well-tested app is a reliable app.
Remember that diligent debugging and testing are not just tasks to check off but ongoing processes that enhance your skills as a developer and lead to the delivery of exceptional apps to your users. So, embrace these practices, refine your skills, and craft iOS apps that stand out in quality and performance. Happy coding!
Table of Contents
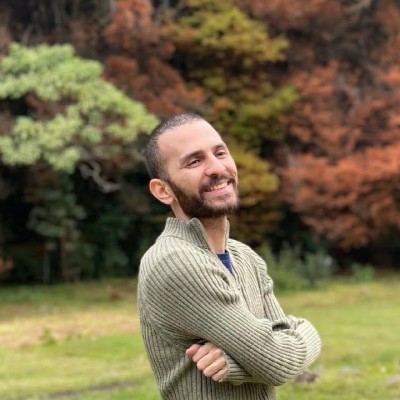
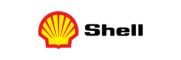