Building Real-Time Chat Apps in iOS: Exploring Firebase Messaging
In the era of instant communication, real-time chat applications have become an integral part of our daily lives. Whether it’s keeping in touch with friends, collaborating with colleagues, or connecting with customers, the demand for seamless and responsive chat apps is higher than ever. If you’re an iOS developer looking to create such apps, Firebase Messaging is a powerful tool that can streamline the development process and provide you with real-time capabilities. In this article, we’ll dive into the world of building real-time chat apps in iOS using Firebase Messaging.
Table of Contents
1. Why Firebase Messaging?
Firebase Messaging, part of the larger Firebase platform by Google, offers a comprehensive set of tools for building real-time features in applications. It provides a cloud-based messaging solution that enables developers to deliver messages reliably and instantly to devices. Whether you’re sending notifications, updates, or facilitating real-time conversations, Firebase Messaging simplifies the complex backend infrastructure required for such functionalities.
2. Key Features and Benefits
- Real-time Delivery: Firebase Messaging enables instant message delivery to devices, ensuring a smooth and real-time chat experience for users.
- Cross-Platform Support: Firebase supports multiple platforms, making it easy to build chat apps that work seamlessly across iOS, Android, and web platforms.
- Notification Handling: Alongside real-time messaging, Firebase also handles push notifications, allowing you to engage users even when the app is not open.
- Scalability: Firebase is built on a cloud infrastructure that automatically scales to accommodate growing user bases, making it suitable for apps of all sizes.
- Offline Support: Firebase provides offline support, ensuring that messages are queued and delivered as soon as the device regains connectivity.
Now that we understand why Firebase Messaging is a compelling choice, let’s explore the step-by-step process of integrating it into your iOS chat application.
Step 1: Setting Up Firebase
Before diving into the development process, you need to set up Firebase for your project. Follow these steps:
1. Create a Firebase Project:
Go to the Firebase Console and create a new project. This project will be the hub for managing your app’s backend.
2. Add iOS App to Project:
In the Firebase Console, add your iOS app by providing the bundle identifier. This step generates a configuration file (GoogleService-Info.plist) that you’ll need to add to your Xcode project.
3. Install Firebase SDK:
Using CocoaPods, add the Firebase SDK to your project. Open your terminal, navigate to your project directory, and run:
bash pod init
Then, add the following line to your Podfile:
ruby pod 'Firebase/Core' pod 'Firebase/Messaging' Run pod install to install the SDK.
4. Configure App Delegate:
In your AppDelegate.swift file, import Firebase and configure it in the application(_:didFinishLaunchingWithOptions:) method:
swift import Firebase func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { FirebaseApp.configure() // Additional setup return true }
Step 2: Handling User Notifications
Firebase Messaging works hand in hand with user notifications to provide a holistic messaging experience. To handle user notifications, follow these steps:
1. Request User Permission:
Request permission to display notifications using the UNUserNotificationCenter:
swift import UserNotifications UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .sound, .badge]) { (granted, error) in if granted { // Handle permission granted } else { // Handle permission denied } }
2. Register for Remote Notifications:
Register for remote notifications in the application(_:didFinishLaunchingWithOptions:) method:
swift application.registerForRemoteNotifications()
3. Handle Token Refresh:
Firebase Messaging uses a device-specific token to send messages. Handle token refresh to ensure your app always has a valid token:
swift func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) { Messaging.messaging().apnsToken = deviceToken } func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) { print("Failed to register: \(error)") }
Step 3: Sending and Receiving Messages
With Firebase Messaging set up, you can now start sending and receiving real-time messages within your app.
1. Sending a Message:
To send a message, you’ll use the send(_:completion:) method from the Messaging class:
swift let message = ["message": "Hello, world!", "sender": "user123"] Messaging.messaging().send(message) { error in if let error = error { print("Error sending message: \(error.localizedDescription)") } else { print("Message sent successfully") } }
2. Receiving Messages:
Firebase Messaging uses a delegate to handle incoming messages. Conform to the MessagingDelegate protocol and implement the messaging(_:didReceive:contentHandler:) method:
swift class MyMessagingDelegate: NSObject, MessagingDelegate { func messaging(_ messaging: Messaging, didReceive remoteMessage: MessagingRemoteMessage) { // Handle the received message } } // In AppDelegate.swift let messagingDelegate = MyMessagingDelegate() func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { Messaging.messaging().delegate = messagingDelegate // Additional setup return true }
Conclusion
Firebase Messaging empowers iOS developers to build robust and responsive real-time chat applications without getting bogged down by complex backend infrastructure. By following the steps outlined in this article, you can seamlessly integrate Firebase Messaging into your iOS app, providing users with a smooth and engaging messaging experience. Whether you’re building a personal chat app or a business collaboration tool, Firebase Messaging has the features and support you need to succeed in the world of real-time communication.
In this article, we’ve explored the key features and benefits of Firebase Messaging, walked through the process of setting up Firebase for your iOS project, handling user notifications, and sending/receiving messages in real-time. Armed with this knowledge and the provided code samples, you’re well on your way to creating your own real-time chat app for iOS.
Remember, the world of real-time communication is constantly evolving, and Firebase Messaging is just one of the tools at your disposal. As you continue to refine your app and explore more advanced features, you’ll be contributing to the exciting landscape of real-time interactions in the digital age.
Table of Contents
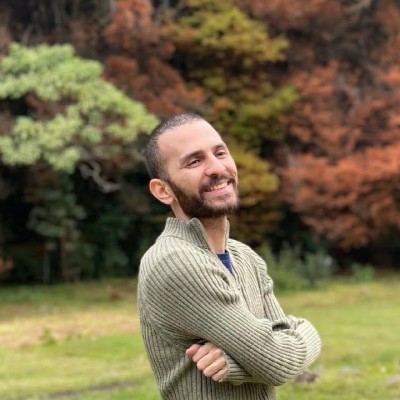
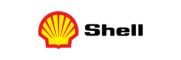