iOS Q & A
How do I handle errors in Swift?
Handling errors in Swift involves identifying, reporting, and recovering from errors that occur during the execution of a program. Swift provides robust error handling mechanisms using the try, catch, and throw keywords, along with error types, to handle and propagate errors effectively.
To handle errors in Swift:
- Throwing Functions and Methods: Define functions and methods that can throw errors using the throws keyword in their signature. Throwing functions indicate that they can fail and may propagate errors to their callers by throwing an error using the throw keyword.
- Error Types: Define custom error types by creating enums that conform to the Error protocol. Error types encapsulate information about specific error conditions and enable type-safe error handling in Swift. Each case of the error enum represents a distinct error condition that can occur during program execution.
- Throwing Errors: Throw errors using the throw keyword followed by an error value or an instance of an error type. Throwing functions and methods use the throw keyword to signal that an error has occurred and terminate execution by propagating the error to the caller.
- Handling Errors: Handle errors using the do-catch statement to catch and handle errors that occur within a block of code. Wrap code that can potentially throw errors inside a do block, and use one or more catch blocks to handle specific error conditions or patterns. Catch blocks specify the types of errors to catch and provide error handling logic to respond to errors appropriately.
- Multiple Catch Blocks: Use multiple catch blocks to handle different types of errors or error conditions separately. Swift matches errors to catch blocks based on their types, allowing developers to implement fine-grained error handling logic for specific error cases.
- Error Propagation: Propagate errors up the call stack by rethrowing errors using the try and catch keywords. Throwing functions can call other throwing functions using the try keyword, and errors are automatically propagated up the call stack until they are caught and handled by a catch block.
- Defer Statements: Use defer statements to execute cleanup code or deferred actions regardless of whether an error occurs or not. defer statements allow developers to ensure that cleanup tasks are performed before exiting a function or scope, regardless of the control flow or error conditions.
By following these steps, you can effectively handle errors in Swift and build robust, reliable, and resilient applications that gracefully handle error conditions and recover from failures. Swift’s expressive error handling syntax and powerful error handling mechanisms enable developers to write safer and more maintainable code, reducing the risk of runtime errors and improving the overall quality of Swift applications.
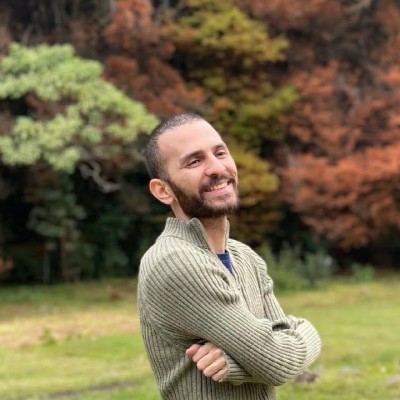
Previously at
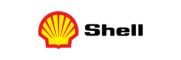
Skilled iOS Engineer with extensive experience developing cutting-edge mobile solutions. Over 7 years in iOS development.