Working with HealthKit in iOS: Building Fitness and Health Apps
In today’s fast-paced world, where health and fitness are becoming increasingly integral to our lifestyles, mobile applications have emerged as powerful tools to help individuals monitor and improve their well-being. Among the many technologies that facilitate this trend, HealthKit stands out as a remarkable framework for building fitness and health apps on the iOS platform. With its seamless integration into the Apple ecosystem, HealthKit empowers developers to access and leverage health-related data, allowing them to create sophisticated applications that cater to users’ diverse health needs.
Table of Contents
1. Understanding HealthKit: A Brief Overview
HealthKit, introduced by Apple in iOS 8, is a framework that enables developers to interact with health and fitness data on iOS devices. It acts as a centralized repository, collecting data from various sources such as the iPhone’s built-in sensors, third-party devices, and user input. This wealth of information includes but is not limited to steps taken, heart rate, sleep patterns, nutrition, and more. By providing a standardized interface for collecting, storing, and retrieving health data, HealthKit simplifies the development process and ensures consistency across different applications.
2. Integrating HealthKit: Getting Started
To start building fitness and health apps with HealthKit, follow these steps:
2.1. Enable HealthKit Capabilities
Before diving into the code, ensure that your Xcode project is configured to work with HealthKit. In your project settings, navigate to the “Signing & Capabilities” tab and add the HealthKit capability. This will enable your app to interact with health data and prompt the user for necessary permissions.
2.2. Requesting User Authorization
User privacy is of paramount importance when dealing with health-related data. HealthKit requires explicit user consent before accessing their health information. To do this, request authorization using the HKHealthStore class. Here’s a code snippet illustrating how to request authorization for step count data:
swift import HealthKit let healthStore = HKHealthStore() let typesToShare: Set<HKSampleType> = [] let typesToRead: Set<HKObjectType> = [HKObjectType.quantityType(forIdentifier: .stepCount)!] healthStore.requestAuthorization(toShare: typesToShare, read: typesToRead) { (success, error) in if success { // User granted authorization } else { // Authorization denied or error occurred } }
2.3. Querying Health Data
Once authorization is granted, you can start querying health data using HKSampleQuery and related classes. The following code demonstrates how to retrieve the user’s daily step count:
swift let stepCountType = HKQuantityType.quantityType(forIdentifier: .stepCount)! let query = HKSampleQuery(sampleType: stepCountType, predicate: nil, limit: HKObjectQueryNoLimit, sortDescriptors: nil) { (query, results, error) in if let stepCountSamples = results as? [HKQuantitySample] { // Process step count data for sample in stepCountSamples { let count = sample.quantity.doubleValue(for: HKUnit.count()) print("Steps: \(count)") } } } healthStore.execute(query)
3. Building a Fitness Dashboard: Putting HealthKit to Work
Let’s dive into a practical example of building a fitness dashboard that displays the user’s daily step count, heart rate, and sleep analysis. This dashboard will showcase how to leverage HealthKit to create a user-friendly and informative interface.
3.1. Designing the User Interface
Begin by designing the interface using Interface Builder. Create a view that includes labels to display step count, heart rate, and sleep analysis. Additionally, add buttons to initiate data retrieval and a user-friendly message for cases where data is unavailable.
3.2. Retrieving and Displaying Data
In the view controller, implement functions to retrieve and display health data. Here’s how you can retrieve and display step count and heart rate data:
swift func fetchStepCount() { // Create and execute a query to fetch step count data // Update the UI with the fetched data } func fetchHeartRate() { // Create and execute a query to fetch heart rate data // Update the UI with the fetched data } @IBAction func retrieveDataButtonTapped(_ sender: UIButton) { fetchStepCount() fetchHeartRate() }
3.3. Enhancing the User Experience
To enhance the user experience, handle scenarios where data is unavailable or the user denies access to health data. Provide informative messages and gracefully guide users to grant necessary permissions.
4. Beyond the Basics: Advanced HealthKit Integration
While retrieving basic health data is valuable, HealthKit offers even more advanced capabilities that can take your fitness and health apps to the next level.
4.1. Writing Data to HealthKit
HealthKit not only allows you to read health data but also enables you to write data back. This is useful for apps that track user-generated information such as dietary intake or workouts. To write data, request authorization and use the appropriate HKObjectType to create and save samples.
4.2. Using Workouts
HealthKit provides a dedicated class, HKWorkout, for tracking workout sessions. You can use this class to create comprehensive workout tracking experiences, complete with start and end times, calorie burn estimates, and associated metadata.
4.3. Data Correlation
HealthKit excels at data correlation, which involves combining different types of health data to derive meaningful insights. For instance, you can correlate heart rate data with sleep patterns to understand how sleep quality affects heart rate.
Conclusion
Incorporating HealthKit into your iOS fitness and health app projects can elevate your applications to new heights. By providing a seamless and secure way to access health-related data, HealthKit empowers developers to build sophisticated apps that cater to users’ well-being. From requesting user authorization to querying and displaying health data, this framework offers a comprehensive toolkit to create compelling fitness experiences. As the world becomes more health-conscious, mastering HealthKit’s capabilities will undoubtedly be a valuable skill for developers aiming to make a positive impact on users’ lives.
Table of Contents
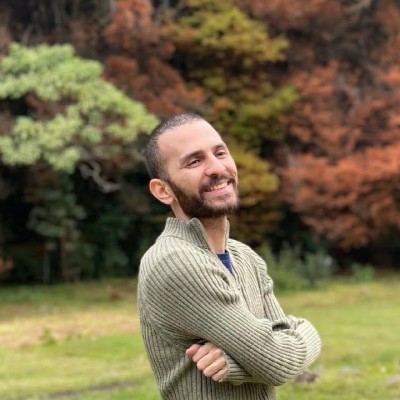
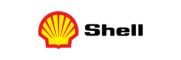