iOS Q & A
How do I implement animations in iOS apps?
Implementing animations in iOS apps can enhance the user experience and make your app more engaging and visually appealing. Here’s how you can implement animations in iOS apps using UIKit:
- UIView Animations:
-
- Use the UIView class methods like animate(withDuration:animations:) to animate changes to UIView properties.
- Define the animation block where you specify the desired changes to the view’s properties, such as its position, size, alpha, or transform.
- UIKit handles the interpolation of property values over the specified duration, creating smooth animations.
swift UIView.animate(withDuration: 0.5) { // Update view properties inside the animation block view.alpha = 0.0 }
- Spring Animations:
-
- Create spring animations using the animate(withDuration:delay:usingSpringWithDamping:initialSpringVelocity:options:animations:completion:) method to add a bounce or elasticity effect to your animations.
- Adjust the damping and initial velocity parameters to control the springiness of the animation.
swift UIView.animate(withDuration: 0.5, delay: 0.0, usingSpringWithDamping: 0.7, initialSpringVelocity: 0.5, options: [], animations: { // Update view properties inside the spring animation block view.transform = CGAffineTransform(scaleX: 1.2, y: 1.2) }, completion: nil)
- Keyframe Animations:
-
- Use keyframe animations to create complex animations with multiple intermediate steps.
- Specify keyframe values and durations to define the animation’s trajectory and timing.
swift UIView.animateKeyframes(withDuration: 1.0, delay: 0.0, options: [], animations: { UIView.addKeyframe(withRelativeStartTime: 0.0, relativeDuration: 0.5) { // Update view properties for the first keyframe view.transform = CGAffineTransform(rotationAngle: .pi) } UIView.addKeyframe(withRelativeStartTime: 0.5, relativeDuration: 0.5) { // Update view properties for the second keyframe view.transform = CGAffineTransform(rotationAngle: 0) } }, completion: nil)
- UIViewPropertyAnimator:
-
- Use UIViewPropertyAnimator for interactive animations and to control animation timing, reversibility, and completion.
- You can pause, resume, or reverse animations based on user interactions.
swift let animator = UIViewPropertyAnimator(duration: 1.0, curve: .easeInOut) { // Update view properties inside the animation closure view.transform = CGAffineTransform(translationX: 100, y: 0) } animator.startAnimation()
- CAAnimation and Core Animation:
-
- For more advanced animations and fine-grained control, you can use Core Animation and its CAAnimation subclasses.
- Core Animation allows you to create animations for individual layers, apply 3D transformations, and use advanced timing functions.
By leveraging these techniques and APIs provided by UIKit and Core Animation, you can implement a wide range of animations in your iOS app to create dynamic and visually appealing user interfaces. Experiment with different animation types, durations, and easing functions to achieve the desired effects and enhance the user experience.
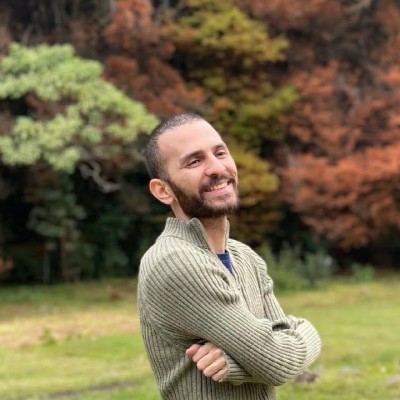
Previously at
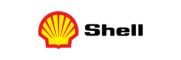
Skilled iOS Engineer with extensive experience developing cutting-edge mobile solutions. Over 7 years in iOS development.