Implementing In-App Purchases in iOS: Monetizing Your Apps
In the ever-evolving landscape of mobile app development, finding the right monetization strategy is crucial to sustaining your efforts and generating revenue. One powerful way to monetize your iOS apps is through in-app purchases (IAPs). In this guide, we’ll delve into the world of in-app purchases and explore how you can effectively implement them to monetize your iOS applications.
Table of Contents
1. Understanding In-App Purchases (IAPs)
1.1. What are In-App Purchases?
In-app purchases (IAPs) refer to the ability for users to buy a variety of content, features, or digital goods within your app. This strategy allows you to provide your app for free on the App Store while offering additional, premium content for purchase. This approach has become a staple for app developers as it grants users a taste of what the app offers before they commit to spending money.
1.2. Types of In-App Purchases
- Consumable: These are items that users can buy, use, and then buy again. Examples include in-game currency, virtual goods, or extra lives.
- Non-Consumable: These are items that users buy once and keep forever. For instance, unlocking a premium version of the app with additional features.
- Subscriptions: Subscriptions grant users access to content or features for a limited time, often on a monthly or yearly basis. This model is great for apps that provide regularly updated content.
- Auto-Renewable Subscriptions: Similar to subscriptions, but they renew automatically unless the user cancels. Common in news or media apps.
- Non-Renewing Subscriptions: These subscriptions don’t renew automatically and require users to manually renew when they expire. Useful for apps with irregular content updates.
2. Getting Started with In-App Purchases
Step 1: Enroll in the Apple Developer Program
Before implementing in-app purchases, you’ll need to be enrolled in the Apple Developer Program. This allows you to access the tools and resources required for integrating IAPs into your app.
Step 2: Set Up In-App Purchase Products
- Open your app in Xcode.
- Navigate to the “Capabilities” tab and enable “In-App Purchase.”
- In App Store Connect, create the products you want to sell.
- Associate your Xcode project with your in-app purchases.
Step 3: Implement StoreKit Framework
The StoreKit framework provides the essential classes and methods for integrating in-app purchases. Here’s a simplified example of how you might fetch and display available products:
swift import StoreKit class StoreManager: NSObject, SKProductsRequestDelegate { var productsRequest: SKProductsRequest? func fetchProducts(productIDs: Set<String>) { productsRequest = SKProductsRequest(productIdentifiers: productIDs) productsRequest?.delegate = self productsRequest?.start() } func productsRequest(_ request: SKProductsRequest, didReceive response: SKProductsResponse) { let products = response.products for product in products { print("Product: \(product.localizedTitle), Price: \(product.price)") } } }
Step 4: Handle Transactions
Once the user initiates a purchase, you’ll need to handle the transactions and provide the purchased content. Here’s a simplified version of how transaction handling might look:
swift class PaymentManager: NSObject, SKPaymentTransactionObserver { func paymentQueue(_ queue: SKPaymentQueue, updatedTransactions transactions: [SKPaymentTransaction]) { for transaction in transactions { switch transaction.transactionState { case .purchased: // Unlock content or provide purchased goods. queue.finishTransaction(transaction) case .failed: // Handle failed transaction. queue.finishTransaction(transaction) case .restored: // Handle restored transaction (for non-consumables and subscriptions). queue.finishTransaction(transaction) case .deferred, .purchasing: // Transaction is in progress, no action needed. break @unknown default: break } } } }
3. Best Practices for Implementing In-App Purchases
- Transparency: Clearly communicate the value of the in-app purchases to users. Ensure they understand what they’re getting and why it’s worth the price.
- Testing: Thoroughly test your in-app purchases in the sandbox environment to ensure a smooth user experience.
- Localized Pricing: Adjust your prices to be regionally appropriate, taking into account currency exchange rates and local purchasing power.
- User Experience: Make the purchasing process seamless and easy to understand. Complicated or confusing purchase flows can deter users.
- Regular Updates: Continuously provide new and enticing content for users who have made purchases. This encourages them to remain engaged and potentially make more purchases.
- Offer Variety: Provide a range of purchase options to cater to different user preferences. This might include different subscription tiers or bundles.
Conclusion
In-app purchases offer a powerful way to monetize your iOS apps while providing users with a choice to access premium content. By understanding the different types of in-app purchases, following the steps to integrate them, and adhering to best practices, you can create a revenue stream that supports the growth and sustainability of your app development efforts. Remember, the key lies not just in monetization, but in delivering value that keeps users coming back for more.
Monetizing your iOS app can be a rewarding endeavor, and in-app purchases are a tool that, when used thoughtfully, can benefit both you and your users. So go ahead, implement in-app purchases with care, and take your app development journey to new heights of success.
Table of Contents
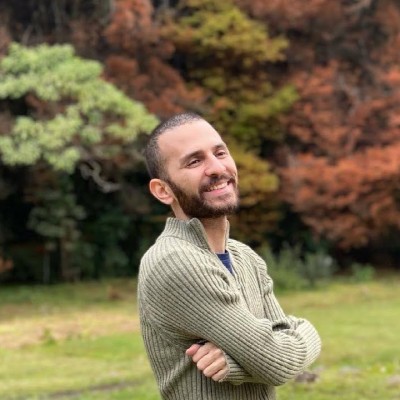
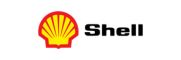