Introduction to SpriteKit: Building 2D Games for iOS
In the ever-evolving world of mobile gaming, creating visually appealing and engaging games is a top priority for developers. With the advent of powerful frameworks like SpriteKit, building immersive 2D games for iOS has become more accessible than ever. Whether you’re a seasoned developer or a newcomer to the world of game development, SpriteKit offers a versatile platform to bring your creative ideas to life. In this blog post, we will delve into the exciting realm of SpriteKit and explore how you can use it to craft captivating 2D games for iOS devices.
1. Understanding SpriteKit: A Brief Overview
SpriteKit, developed by Apple, is a 2D game framework that provides developers with a robust set of tools for creating captivating games for iOS, macOS, watchOS, and tvOS platforms. With SpriteKit, you can effortlessly integrate animations, physics, sound effects, and more into your games, resulting in a seamless and engaging user experience.
2. Key Features of SpriteKit
Node-Based Hierarchy: SpriteKit employs a hierarchical node-based structure, making it easy to organize and manipulate elements in your game scene. Each node can represent a character, object, or background element, allowing for intuitive control over various aspects of your game.
swift let playerNode = SKSpriteNode(imageNamed: "player") playerNode.position = CGPoint(x: 100, y: 200) self.addChild(playerNode) // Adding the player node to the scene
Physics Engine: SpriteKit’s built-in physics engine enables realistic interactions between game elements. Define physical properties like gravity, collisions, and forces to create dynamic and lifelike gameplay.
swift playerNode.physicsBody = SKPhysicsBody(rectangleOf: playerNode.size) playerNode.physicsBody?.affectedByGravity = true playerNode.physicsBody?.categoryBitMask = playerCategory
Animation and Actions: Animating elements is a breeze with SpriteKit’s animation system. You can create smooth transitions, rotations, and more using simple actions.
swift let moveAction = SKAction.moveBy(x: 100, y: 0, duration: 2.0) playerNode.run(SKAction.repeatForever(moveAction))
Particle Systems: Enhance your game’s visual effects with particle systems. From dazzling explosions to swirling vortexes, particle systems can add an extra layer of excitement to your gameplay.
swift if let particleEmitter = SKEmitterNode(fileNamed: "ExplosionParticle.sks") { playerNode.addChild(particleEmitter) }
3. Setting Up Your SpriteKit Project
Before diving into the intricacies of SpriteKit, you need to set up your Xcode project for game development. Follow these steps to get started:
Step 1: Create a New Project
- Launch Xcode and select “Create a new Xcode project.”
- Choose the “Game” template under iOS application.
- Select “SpriteKit” as the game technology.
Step 2: Design Your Game Scene
- Locate the GameScene.sks file in your project.
- Use the SpriteKit Scene Editor to design your game’s initial scene layout.
- Drag and drop nodes onto the scene to represent characters, objects, and background elements.
4. Building Your First SpriteKit Game
Let’s create a simple example of a SpriteKit game—a classic “Dodge the Enemy” scenario. In this game, the player will control a character and avoid incoming enemy obstacles.
Step 1: Adding Player and Enemy Nodes
In your GameScene.swift file, you can add player and enemy nodes within the didMove(to:) function:
swift class GameScene: SKScene { let playerNode = SKSpriteNode(imageNamed: "player") let enemyNode = SKSpriteNode(imageNamed: "enemy") override func didMove(to view: SKView) { playerNode.position = CGPoint(x: size.width * 0.5, y: 100) enemyNode.position = CGPoint(x: size.width * 0.5, y: size.height - 100) addChild(playerNode) addChild(enemyNode) } }
Step 2: Responding to Player Input
To make the player character move in response to touch input, override the touchesBegan(_:with:) function:
swift override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) { if let touch = touches.first { let touchLocation = touch.location(in: self) let moveAction = SKAction.move(to: touchLocation, duration: 0.5) playerNode.run(moveAction) } }
Step 3: Adding Collision Detection
Implement collision detection to check if the player collides with the enemy:
swift enum PhysicsCategory { static let player: UInt32 = 0b01 static let enemy: UInt32 = 0b10 } // Inside didMove(to:) playerNode.physicsBody = SKPhysicsBody(rectangleOf: playerNode.size) playerNode.physicsBody?.categoryBitMask = PhysicsCategory.player playerNode.physicsBody?.contactTestBitMask = PhysicsCategory.enemy playerNode.physicsBody?.collisionBitMask = 0 enemyNode.physicsBody = SKPhysicsBody(rectangleOf: enemyNode.size) enemyNode.physicsBody?.categoryBitMask = PhysicsCategory.enemy enemyNode.physicsBody?.collisionBitMask = 0 enemyNode.physicsBody?.contactTestBitMask = PhysicsCategory.player
Step 4: Handling Collisions
Handle collisions by conforming to the SKPhysicsContactDelegate protocol and implementing the didBegin(_:) function:
swift class GameScene: SKScene, SKPhysicsContactDelegate { override func didMove(to view: SKView) { physicsWorld.contactDelegate = self } func didBegin(_ contact: SKPhysicsContact) { if contact.bodyA.categoryBitMask == PhysicsCategory.player || contact.bodyB.categoryBitMask == PhysicsCategory.player { // Handle player-enemy collision } } }
Conclusion
SpriteKit opens up a world of possibilities for creating captivating 2D games for iOS devices. With its intuitive node-based structure, built-in physics engine, animation capabilities, and more, SpriteKit provides a comprehensive toolkit for game development. This introductory guide has provided a glimpse into the power and flexibility of SpriteKit. Armed with this knowledge, you’re ready to embark on your journey of crafting exciting and visually appealing games that will captivate players on the iOS platform. So, gather your ideas, fire up Xcode, and let your creativity flow as you dive into the fascinating realm of SpriteKit.
Table of Contents
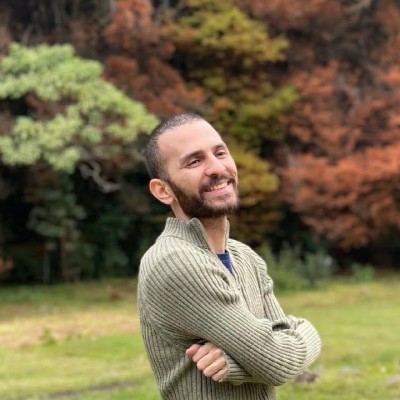
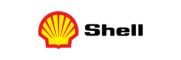