How do I parse JSON in iOS apps?
Parsing JSON (JavaScript Object Notation) is a common task in iOS app development, as many APIs and web services return data in JSON format. JSON is a lightweight and human-readable data interchange format used for transmitting structured data between a server and a client. iOS provides built-in support for parsing JSON data using the Foundation framework, allowing developers to convert JSON data into native Swift objects or structures. Here’s how you can parse JSON in iOS apps:
- Decode JSON with Codable: Codable is a protocol provided by Swift for encoding and decoding data between JSON and native Swift types. Codable enables you to define Swift structs or classes that conform to the Codable protocol, specifying how JSON data should be mapped to Swift properties. You can use the JSONDecoder class provided by the Foundation framework to decode JSON data into Codable objects, or use the JSONEncoder class to encode Codable objects into JSON data.
- Create Codable Data Models: Before parsing JSON data, define Codable data models representing the structure of the JSON data. Codable data models consist of Swift structs or classes that conform to the Codable protocol and define properties corresponding to the JSON keys and values. Codable data models specify the data structure and types of the JSON data, allowing you to map JSON data to Swift objects or structures.
- Decode JSON with JSONDecoder: Once you have defined Codable data models, use the JSONDecoder class provided by the Foundation framework to decode JSON data into Codable objects. JSONDecoder decodes JSON data from an input source, such as a Data object or a file, into Codable objects by mapping JSON keys to Swift properties based on the Codable data model’s structure. You can customize the decoding process by providing decoding options and handling errors or exceptions.
- Handle Decoding Errors: When decoding JSON data into Codable objects, handle decoding errors and exceptions that may occur during the decoding process. JSONDecoder throws an error if it encounters invalid JSON data, missing keys, or type mismatches while decoding. Handle decoding errors using error handling mechanisms such as do-catch statements or try? and try! expressions to gracefully handle errors and recover from failures.
- Access Decoded Data: After decoding JSON data into Codable objects, access the decoded data by accessing properties and values of the Codable objects. Use dot syntax to access properties of Codable objects and retrieve values parsed from JSON data. You can access nested properties, arrays, dictionaries, and optional values of Codable objects to extract and use the parsed data in your app’s logic and user interface.
By following these steps, you can effectively parse JSON data in iOS apps using Codable and the Foundation framework’s JSONDecoder class. Codable simplifies the process of mapping JSON data to Swift objects or structures, providing a type-safe and efficient way to work with JSON data in iOS development.
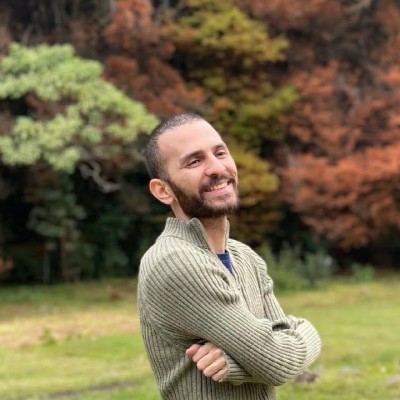
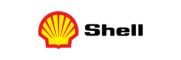