Exploring Core Spotlight in iOS: Search and Index Content in Your Apps
Mobile applications have become an integral part of our lives, catering to a wide range of needs. One of the key features that users expect in any app is a robust and efficient search functionality. To meet this demand, iOS provides a powerful framework called Core Spotlight, which allows developers to easily index and search content within their apps. In this comprehensive guide, we will delve into the world of Core Spotlight, exploring its capabilities, and learning how to implement it in your iOS applications.
Table of Contents
1. Understanding Core Spotlight
1.1. What is Core Spotlight?
Core Spotlight is a framework introduced by Apple that enables developers to index and search content within their iOS applications. This framework integrates seamlessly with iOS’s built-in search functionality, making it easier for users to discover content within your app.
1.2. Key Features of Core Spotlight:
1.2.1. Indexing Content
Core Spotlight allows you to index various types of content within your app, including text, images, videos, and documents. This indexing process makes it possible for the system to search and retrieve specific items quickly.
1.2.2. User-Friendly Search
The indexed content becomes accessible through the iOS Spotlight search, making it convenient for users to find relevant information directly from their device’s home screen.
1.2.3. Deep Linking
Core Spotlight provides deep linking capabilities, allowing users to launch your app and navigate to the exact location of the indexed content.
Now that we have a basic understanding of what Core Spotlight is, let’s dive into the practical implementation of this framework in your iOS app.
2. Implementing Core Spotlight in Your iOS App
2.1. Prerequisites
Before you start implementing Core Spotlight, ensure you have the following prerequisites in place:
- Xcode (latest version).
- A Mac running macOS (to develop iOS apps).
- Basic knowledge of Swift or Objective-C programming languages.
- An existing iOS app project or a new one you’d like to integrate Core Spotlight into.
2.2. Indexing Content
Step 1: Import Core Spotlight Framework
To begin using Core Spotlight in your iOS app, you need to import the Core Spotlight framework. Open your Xcode project, go to the project settings, and under “Linked Frameworks and Libraries,” add “CoreSpotlight.framework.”
swift import CoreSpotlight
Step 2: Define Your Data Model
Before you can index content, you should have a clear data model representing the content you want to make searchable. For example, if you’re building a note-taking app, your data model might include a Note class with properties like title, content, and date.
swift struct Note { let title: String let content: String let date: Date }
Step 3: Create Searchable Items
To make your content searchable, you’ll need to create CSSearchableItem objects and add them to the Core Spotlight index. Here’s an example of how you can create a searchable item for a note in your app:
swift func indexNote(note: Note) { let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = note.title attributeSet.contentDescription = note.content attributeSet.creationDate = note.date let searchableItem = CSSearchableItem(uniqueIdentifier: note.title, domainIdentifier: "com.example.myapp.notes", attributeSet: attributeSet) CSSearchableIndex.default().indexSearchableItems([searchableItem]) { error in if let error = error { print("Error indexing note: \(error.localizedDescription)") } else { print("Note indexed successfully!") } } }
In this code snippet, we first create an CSSearchableItemAttributeSet to define the attributes of our searchable item, such as the title, content description, and creation date. Then, we create a CSSearchableItem object with a unique identifier and domain identifier.
Finally, we use the indexSearchableItems method of CSSearchableIndex.default() to add the item to the Core Spotlight index. Don’t forget to handle errors appropriately.
Step 4: Index Your Content
Now that you have a function to index a single note, you can loop through your app’s data and index all relevant content. This might involve iterating through an array of notes and calling the indexNote function for each one.
swift let notes: [Note] = // Your array of notes for note in notes { indexNote(note: note) }
Congratulations! You’ve successfully indexed your app’s content using Core Spotlight. Users can now search for your notes using iOS’s Spotlight search.
2.3. Searching Content
Step 1: Implementing Search
To allow users to search your app’s content using iOS Spotlight, you don’t need to do much. iOS handles the search interface for you. However, you should implement a delegate method to handle the selection of search results.
swift func application(_ application: UIApplication, continue userActivity: NSUserActivity, restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool { if userActivity.activityType == CSSearchableItemActionType { if let noteTitle = userActivity.userInfo?[CSSearchableItemActivityIdentifier] as? String { // Handle the selection of the searchable item here // You can open your app and display the selected note // using the noteTitle identifier return true } } return false }
In this delegate method, you check if the user activity’s activity type is CSSearchableItemActionType, indicating that a searchable item was selected. You can then extract the unique identifier of the selected item and use it to navigate to the corresponding content within your app.
Step 2: User Experience Enhancement
To enhance the user experience, you can also provide relevant search suggestions by adopting the UISearchControllerDelegate protocol and implementing the didPresentSearchController method:
swift func didPresentSearchController(_ searchController: UISearchController) { // Provide search suggestions here based on user input or app history searchController.searchSuggestions = // Your search suggestions }
This method allows you to present search suggestions as the user interacts with the search interface, making your app even more user-friendly.
3. Advanced Usage of Core Spotlight
3.1. Customizing Searchable Item Attributes
You can customize the attributes of your searchable items to provide more context to the users’ search results. For example, you can add an image to the attribute set to display a thumbnail alongside the search result.
swift attributeSet.thumbnailData = // Your image data
By adding relevant attributes, you can make your search results more informative and visually appealing.
3.2. Handling Updates and Deletions
As your app’s content evolves, you may need to update or delete searchable items. Core Spotlight provides methods to handle these scenarios. To update an item, simply index it again with the same unique identifier. To delete an item, use the deleteSearchableItems(withIdentifiers:) method.
swift let updatedNote: Note = // The updated note indexNote(note: updatedNote) // Update an existing item let noteToDelete: Note = // The note to delete CSSearchableIndex.default().deleteSearchableItems(withIdentifiers: [noteToDelete.title]) { error in if let error = error { print("Error deleting note: \(error.localizedDescription)") } else { print("Note deleted successfully!") } }
3.3. Core Spotlight and Security
It’s essential to be mindful of user privacy and data security when using Core Spotlight. Ensure that you only index content that the user has permission to access and that you handle user data responsibly.
Conclusion
Core Spotlight is a powerful framework for enhancing the search functionality of your iOS applications. With the ability to index, search, and deep link to your app’s content, it provides a seamless user experience. By following the steps outlined in this guide, you can easily integrate Core Spotlight into your iOS app, making it more discoverable and user-friendly.
Remember to keep user privacy and data security in mind while using Core Spotlight, and regularly update and optimize your indexed content to provide the best possible search experience for your app’s users.
Incorporating Core Spotlight into your iOS app can lead to increased user engagement and satisfaction, as users can quickly find the content they need. So why wait? Start exploring Core Spotlight today and take your iOS app’s search functionality to the next level. Happy coding!
Table of Contents
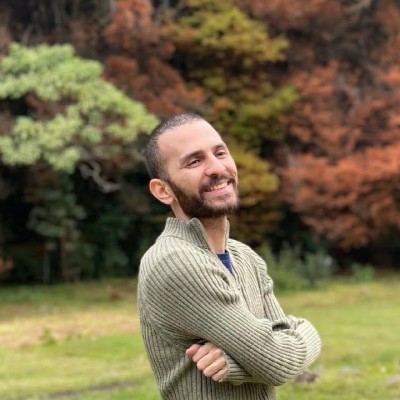
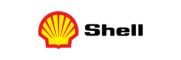