iOS Q & A
How do I implement the Singleton pattern in Swift?
The Singleton pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. In Swift, you can implement the Singleton pattern using a private initializer and a static property to hold the single instance. Here’s how you can implement the Singleton pattern in Swift:
swift class Singleton { // Static property to hold the single instance static let shared = Singleton() // Private initializer to prevent external instantiation private init() { // Initialization code } // Additional methods and properties }
In this implementation:
- The class Singleton has a private initializer to prevent external instantiation of the class.
- It declares a static property named shared of type Singleton, which holds the single instance of the class. This property is accessed using the class name (Singleton.shared).
- The single instance is lazily initialized the first time it’s accessed, thanks to Swift’s lazy initialization mechanism.
- Clients access the singleton instance using the static shared property, ensuring that only one instance of the class exists throughout the app’s lifecycle.
You can then access the singleton instance from anywhere in your code using Singleton.shared.
swift let singletonInstance = Singleton.shared
The Singleton pattern is commonly used for managing global resources, shared configurations, or centralized services in iOS apps. However, it’s essential to use singletons judiciously, as they can introduce tight coupling and make testing and debugging more challenging.
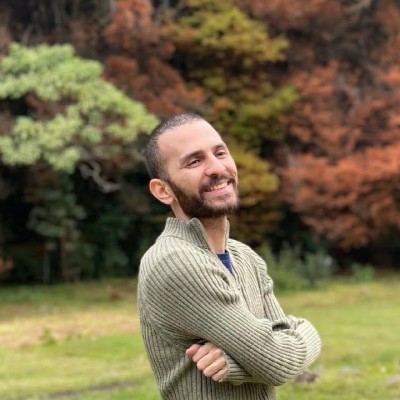
Previously at
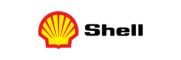
Skilled iOS Engineer with extensive experience developing cutting-edge mobile solutions. Over 7 years in iOS development.