Mastering Modern App Development with SwiftUI Tutorial
If you are interested in creating your own iOS apps, there’s no better way to start than by learning SwiftUI, Apple’s intuitive and comprehensive framework for creating slick, interactive user interfaces for all Apple devices. With SwiftUI, you can bring your app to life with less code and a live preview, giving you real-time feedback on your design.
This step-by-step tutorial will guide you through building a basic iOS app using SwiftUI. However, if you find that you want to expand your project or need help along the way, don’t hesitate to hire iOS developers who are well-versed in SwiftUI. Their expertise can elevate your app and make the development process smoother and more efficient.
Whether you’re diving into iOS development solo or planning to hire iOS developers for your projects, SwiftUI is an excellent starting point. Enjoy the journey!
Step 1: Setting up Your Environment
Before you begin, ensure that you have the latest version of Xcode installed. Xcode is Apple’s Integrated Development Environment (IDE) where you’ll be writing your Swift and SwiftUI code. As of my knowledge cutoff in September 2021, the latest version is Xcode 13.
Once you have Xcode installed, launch it, and let’s create a new SwiftUI project. Go to File > New > Project. In the ensuing dialog box, select “App” under the “iOS” header, then click “Next.”
Give your app a name; let’s call ours “InteractiveTodoList”. Make sure the Interface is set to SwiftUI, and the Language is set to Swift. Select a location to save your project, and click “Create.”
Step 2: Understanding the SwiftUI Project Structure
With your project created, Xcode will display several files in the project navigator. Here’s what they mean:
– `ContentView.swift`: This is where you’ll build your app’s UI.
– `InteractiveTodoListApp.swift`: This is the entry point of your application, where the app launches your interface.
– `Assets.xcassets`: This is where you can store your images and icons.
– `Info.plist`: This file contains configuration settings for your app.
Step 3: Creating the User Interface
Let’s start by creating the interface for our to-do list app. Open the `ContentView.swift` file. Here’s what it looks like to start:
```swift import SwiftUI struct ContentView: View { var body: some View { Text("Hello, world!") .padding() } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } } ```
We will create a simple list view to display our tasks and a text field to add new tasks. Replace your `ContentView.swift` with the following:
```swift import SwiftUI struct ContentView: View { @State private var newTodo: String = "" @State private var allTodos: [String] = ["Sample Task"] var body: some View { NavigationView { VStack { HStack { TextField("New task", text: $newTodo) .textFieldStyle(RoundedBorderTextFieldStyle()) Button(action: { allTodos.append(newTodo) newTodo = "" }) { Image(systemName: "plus") } }.padding() List { ForEach(allTodos, id: \.self) { todo in Text(todo) } } } .navigationBarTitle("Tasks") } } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } } ```
We added a `NavigationView` for a navigation bar, an `HStack` for horizontal alignment of items, a `TextField` for user input, and a `Button` for adding tasks. Notice the use of `@State` for stateful variables; it allows SwiftUI to refresh the view when these variables change.
Step 4: Making the To-Do List Interactive
Next, let’s make our to-do list interactive. We want users to be able to mark tasks as complete by tapping them. We’ll make completed tasks display with a strikethrough.
Modify the `ForEach` loop in `ContentView.swift` as follows:
```swift ForEach(allTodos.indices, id: \.self) { index in Button(action: { allTodos[index] = allTodos[index] + (allTodos[index].contains("?") ? "" : " ?") }) { Text(allTodos[index]) .strikethrough(allTodos[index].contains("?"), color: .black) } } ```
We’ve turned each task into a `Button` that, when tapped, appends a “?” symbol to the task. The `.strikethrough` modifier adds a line through the task if it has been marked as completed.
Step 5: Adding Task Deletion Functionality
Our to-do list app is already quite useful, but there’s one thing missing: the ability to delete tasks. Let’s add that now.
Add the `.onDelete` modifier to the `List` as follows:
```swift List { ForEach(allTodos.indices, id: \.self) { index in Button(action: { allTodos[index] = allTodos[index] + (allTodos[index].contains("?") ? "" : " ?") }) { Text(allTodos[index]) .strikethrough(allTodos[index].contains("?"), color: .black) } } .onDelete(perform: removeTodos) } ```
Also, add the `removeTodos` method:
```swift func removeTodos(at offsets: IndexSet) { allTodos.remove(atOffsets: offsets) } ```
By swiping a task to the left, you will now see a delete option appear, which you can use to remove the task from the list.
Conclusion
And that’s it! We have just created an interactive iOS app with SwiftUI. We learned how to create a user interface, add interactivity with stateful variables, and implement task deletion functionality.
This is just the beginning of what you can create with SwiftUI. It’s a powerful tool that can be used to create complex, beautiful, and interactive applications for all Apple platforms. If you are looking to create more advanced apps or projects, consider the opportunity to hire iOS developers who have deep experience with SwiftUI.
Start building your own apps today, and see what you can create! Whether you’re working independently or collaborating with hired iOS developers, the possibilities are vast.
Remember that practice is crucial when learning any new technology. Don’t be afraid to experiment with different views and modifiers to create unique and interesting user experiences. Happy coding!
Table of Contents
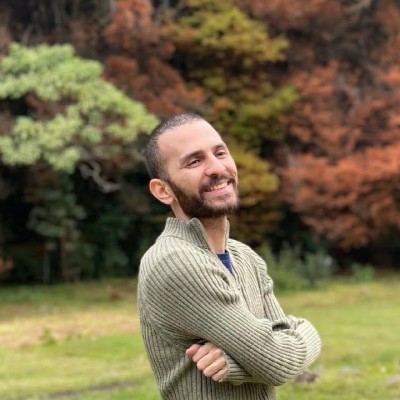
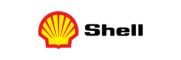