Java for Beginners: An Introduction to the Basics and Syntax of Java Programming
Welcome to Java Programming 101! Today, we dive into the world of Java, an object-oriented programming language that’s widely used for developing web applications, mobile apps, game development, and much more. Its ease of use and versatility make Java a preferred choice for many companies looking to hire Java developers. The simplicity of Java also makes it a great stepping stone for beginners starting their coding journey.
Let’s start with the basics, shall we?
1. Introduction to Java
Developed by Sun Microsystems (now Oracle Corporation) in 1995, Java is a high-level, class-based, object-oriented language. Its design was influenced by the principle of “Write Once, Run Anywhere” (WORA), meaning compiled Java code can run on any platform that supports Java without the need for recompilation. This compatibility feature has largely contributed to its immense popularity and is one of the reasons why many companies choose to hire Java developers.
One of the main features that distinguish Java from other languages is its robust security. It achieves this by making use of a bytecode verification process and a sandbox model that limits the access of Java code to system resources. This high level of security is another driving factor for organizations looking to hire Java developers, as it ensures their applications are secure from potential threats.
2. Setting Up Your Java Environment
Before you start programming, you’ll need to install the Java Development Kit (JDK), which is a software development environment used for developing Java applications. After installation, ensure your JDK is correctly set up by opening a command prompt or terminal and typing the following:
```java java -version ```
If your JDK is correctly set up, you should see an output similar to this:
```java java version "1.8.0_231" Java(TM) SE Runtime Environment (build 1.8.0_231-b11) Java HotSpot(TM) 64-Bit Server VM (build 25.231-b11, mixed mode) ```
3. Your First Java Program
After setting up your Java environment, it’s time to write your first program. In Java, every application begins with a class name, and that class must match the filename.
Let’s create a program named “HelloWorld.java“. Open your text editor and type the following lines of code:
```java public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } } ```
This program consists of three sections:
- The first line defines a class named `HelloWorld`. Java is an object-oriented language, and everything in Java revolves around classes and objects.
- The second line is the main method. This is the entry point of any Java code. The Java Virtual Machine (JVM) calls this method when the program is executed.
- The third line is a simple print statement. It uses the `System.out.println()` function to print “Hello, World!” to the console.
To run your program, save your file as “HelloWorld.java”. Then, in your terminal, navigate to where your file is saved, and compile it using the Java compiler `javac`:
```java javac HelloWorld.java ```
Then, use the `java` command to run your compiled code:
```java java HelloWorld ```
If everything is working correctly, you’ll see `Hello, World!` printed to your terminal.
4. Understanding Java Syntax
Now that we’ve covered the basics, let’s delve a little deeper into the syntax.
4.1 Variables and Data Types
Variables are used to store data, which can be of various types. In Java, there are several types of variables, including:
– Integer (`int`): This can hold a whole number without decimals, such as 123 or -456.
– Float (`float`): This can hold a floating point number, like 3.14 or -38.4.
– String (`String`): This is used to store text.
Here is an example of declaring variables in Java:
```java public class Main { public static void main(String[] args) { int myNum = 5; float myFloatNum = 5.99f; String myText = "Hello"; System.out.println(myNum); System.out.println(myFloatNum); System.out.println(myText); } } ```
4.2 Operators
Operators are used to perform operations on variables and values. The most commonly used operators in Java are:
– Arithmetic Operators: `+`, `-`, `*`, `/`, `%`, `++`, `–`.
– Assignment Operators: `=`, `+=`, `-=`, `*=`, `/=`, `%=`, `&=`, `|=`, `^=`, `>>=`, `<<=`.
– Comparison Operators: `==`, `!=`, `>`, `<`, `>=`, `<=`.
– Logical Operators: `&&`, `||`, `!`.
4.3 Control Flow Statements
Java uses control flow statements to make decisions and loop over sections of code. There are several types of control flow statements, including `if`, `else`, `for`, `while`, and `switch`.
Here is an example of a control flow statement:
```java public class Main { public static void main(String[] args) { int temp = 25; if (temp > 30) { System.out.println("It's hot outside!"); } else { System.out.println("It's not hot outside."); } } } ```
Conclusion
The basics covered in this post provide a foundation for Java programming. However, Java is a rich language with numerous features not covered here, including classes, objects, exceptions, threads, and more. As you continue your Java journey, you’ll encounter these topics and delve into more complex applications of the language. This expertise is highly sought after by businesses seeking to hire Java developers.
Remember, becoming a competent programmer doesn’t happen overnight. It’s a journey filled with many challenges and successes. The journey from learning the basics to becoming proficient is exactly what companies look for when they hire Java developers. So, keep learning and practicing, and you’ll be amazed at how far you can go with Java!
Happy Coding!
Table of Contents
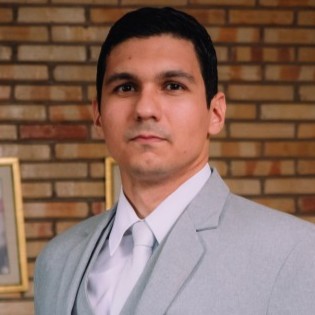
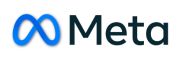