Java Cryptography: Securing Data and Communications
In today’s interconnected digital world, securing sensitive data and communications is paramount. With cyber threats on the rise, businesses and individuals alike must employ robust encryption techniques to safeguard their information from prying eyes. Java, with its powerful cryptography libraries, offers a comprehensive toolkit for implementing secure communication protocols and protecting data integrity. In this article, we’ll delve into the world of Java cryptography, exploring its capabilities and providing practical examples of how it can be used to enhance security.
Understanding Java Cryptography
Java Cryptography Architecture (JCA) is a part of the Java Development Kit (JDK) that provides a framework and implementation for encryption, key generation, digital signatures, and secure communication protocols. It includes a set of APIs and cryptographic algorithms that enable developers to build secure applications without having to implement cryptographic functions from scratch.
Key Components of Java Cryptography
- Java Cryptography Extension (JCE): This extension to the JCA provides additional cryptographic services, algorithms, and key generation facilities. It enhances the capabilities of the standard JCA by offering a wider range of cryptographic algorithms and stronger security features.
- Java Secure Socket Extension (JSSE): JSSE enables secure communication over the network by implementing SSL/TLS protocols. It allows Java applications to establish encrypted connections with remote servers, ensuring confidentiality and integrity of data exchanged over the network.
Examples of Java Cryptography in Action
1. Data Encryption and Decryption
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; public class DataEncryptionExample { public static void main(String[] args) throws Exception { // Generate a secret key KeyGenerator keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(128); // AES key size SecretKey secretKey = keyGenerator.generateKey(); // Initialize the cipher for encryption Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, secretKey); // Encrypt the data byte[] encryptedData = cipher.doFinal("Sensitive information".getBytes()); // Initialize the cipher for decryption cipher.init(Cipher.DECRYPT_MODE, secretKey); // Decrypt the data byte[] decryptedData = cipher.doFinal(encryptedData); // Print the decrypted data System.out.println(new String(decryptedData)); } }
This example demonstrates how to encrypt and decrypt data using the AES algorithm, a widely used symmetric encryption algorithm supported by Java’s cryptography libraries.
2. Digital Signatures
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; import java.security.Signature; public class DigitalSignatureExample { public static void main(String[] args) throws Exception { // Generate a key pair KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA"); keyPairGenerator.initialize(2048); // RSA key size KeyPair keyPair = keyPairGenerator.generateKeyPair(); // Create a signature object Signature signature = Signature.getInstance("SHA256withRSA"); // Initialize the signature with the private key signature.initSign(keyPair.getPrivate()); // Generate a digital signature for the data byte[] data = "Data to be signed".getBytes(); signature.update(data); byte[] digitalSignature = signature.sign(); // Initialize the signature with the public key for verification signature.initVerify(keyPair.getPublic()); // Verify the digital signature signature.update(data); boolean verified = signature.verify(digitalSignature); // Print the verification result System.out.println("Signature verified: " + verified); } }
This example illustrates how to generate and verify digital signatures using the RSA algorithm, enabling data integrity and authenticity verification.
3. Secure Communication with HTTPS
import java.net.URL; import javax.net.ssl.HttpsURLConnection; public class HttpsConnectionExample { public static void main(String[] args) throws Exception { // Create an HTTPS connection URL url = new URL("https://www.example.com"); HttpsURLConnection connection = (HttpsURLConnection) url.openConnection(); // Set up SSL context (optional) // SSLContext sslContext = SSLContext.getInstance("TLS"); // sslContext.init(null, null, new SecureRandom()); // connection.setSSLSocketFactory(sslContext.getSocketFactory()); // Send an HTTPS request connection.setRequestMethod("GET"); connection.connect(); // Read the response int responseCode = connection.getResponseCode(); System.out.println("Response Code: " + responseCode); } }
This example demonstrates how to establish a secure HTTPS connection using Java’s HttpsURLConnection class, ensuring encrypted communication with remote servers.
Conclusion
Java cryptography provides a robust framework for securing data and communications in Java applications. By leveraging its powerful APIs and algorithms, developers can implement encryption, digital signatures, and secure communication protocols to protect sensitive information from unauthorized access and tampering. As cyber threats continue to evolve, adopting strong cryptographic practices is essential for safeguarding digital assets and maintaining trust in the digital ecosystem.
External Resources
- Oracle Java Cryptography Architecture
- Java Cryptography Extension (JCE) Documentation
- Java Secure Socket Extension (JSSE) Guide
By mastering Java cryptography, you can encrypt, sign, and secure your applications to create ironclad data protection. Happy coding!
Table of Contents
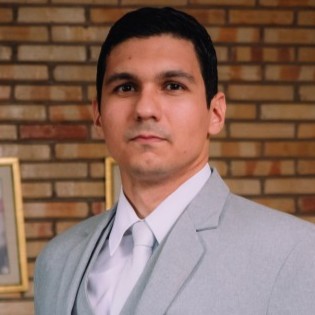
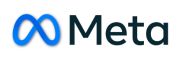