JavaFX Custom Controls: Building Reusable UI Components
JavaFX is a powerful framework for building rich and interactive user interfaces in Java. One of the key features of JavaFX is the ability to create custom controls that can be reused across different parts of your application. This article explores how to build custom UI components in JavaFX, providing practical examples to help you enhance your application’s user interface.
Understanding JavaFX Custom Controls
Custom controls in JavaFX allow you to create unique UI components that are tailored to your application’s needs. These controls can be styled, extended, and reused, making them a vital part of any well-structured JavaFX application.
Creating a Simple Custom Control
The first step in building custom controls is to create a basic component. JavaFX provides a flexible architecture that allows you to extend existing controls or build entirely new ones from scratch.
Example: Creating a Custom Button
Let’s start with a simple example of creating a custom button with enhanced functionality.
```java import javafx.scene.control.Button; public class CustomButton extends Button { public CustomButton(String text) { super(text); this.setStyle("-fx-background-color: #007bff; -fx-text-fill: white;"); this.setOnMouseEntered(e -> this.setStyle("-fx-background-color: #0056b3;")); this.setOnMouseExited(e -> this.setStyle("-fx-background-color: #007bff;")); } } ```
In this example, we extend the `Button` class to create a `CustomButton` with specific styling and hover effects. This custom button can be reused across different scenes in your application.
Adding Custom Properties
Custom controls can also include properties that allow you to customize their behavior and appearance dynamically.
Example: Adding a Custom Property
Here’s how you can add a custom property to your control.
```java import javafx.beans.property.SimpleStringProperty; import javafx.beans.property.StringProperty; import javafx.scene.control.Label; public class CustomLabel extends Label { private final StringProperty customText = new SimpleStringProperty(); public CustomLabel() { textProperty().bind(customText); } public String getCustomText() { return customText.get(); } public void setCustomText(String customText) { this.customText.set(customText); } public StringProperty customTextProperty() { return customText; } } ```
In this example, the `CustomLabel` class includes a `StringProperty` named `customText` that can be bound to other properties or modified programmatically.
Composing Complex Controls
Custom controls can also be composed of multiple existing controls to create more complex UI components.
Example: Creating a Custom Form Control
Let’s create a custom form control that combines a `TextField` and a `Label` for better user input handling.
```java import javafx.geometry.Insets; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.layout.HBox; public class LabeledTextField extends HBox { private final Label label; private final TextField textField; public LabeledTextField(String labelText) { this.label = new Label(labelText); this.textField = new TextField(); this.getChildren().addAll(label, textField); this.setSpacing(10); this.setPadding(new Insets(5)); } public String getText() { return textField.getText(); } public void setText(String text) { textField.setText(text); } } ```
This `LabeledTextField` custom control combines a `Label` and a `TextField` into a single reusable component that can be used for form inputs.
Styling Custom Controls with CSS
JavaFX supports CSS for styling, allowing you to customize the appearance of your controls in a flexible and maintainable way.
Example: Styling a Custom Control with CSS
You can define a CSS file and link it to your custom controls for consistent styling across your application.
```css .custom-button { -fx-background-color: #007bff; -fx-text-fill: white; } .custom-button:hover { -fx-background-color: #0056b3; } ``` And apply this style in your custom button class: ```java public class CustomButton extends Button { public CustomButton(String text) { super(text); this.getStyleClass().add("custom-button"); } } ```
Conclusion
JavaFX’s support for custom controls provides a powerful way to create reusable and dynamic UI components tailored to your application’s specific needs. By understanding the basics of creating, composing, and styling custom controls, you can significantly enhance the user experience and maintainability of your JavaFX applications.
Further Reading:
Table of Contents
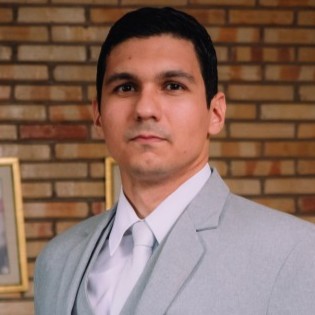
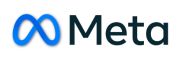