Java Database Connectivity (JDBC): Integrating with Databases
In the world of software development, databases play a pivotal role in storing and managing data. To interact with databases effectively, Java Database Connectivity (JDBC) is an indispensable technology. JDBC enables Java applications to communicate with various relational databases, making data retrieval, manipulation, and management a seamless process. In this article, we will delve into the intricacies of JDBC, exploring its features, benefits, and providing hands-on code samples to illustrate its usage.
Table of Contents
1. Understanding JDBC
1.1. What is JDBC?
Java Database Connectivity (JDBC) is a Java-based API that facilitates the interaction between Java applications and relational databases. It acts as a bridge, allowing developers to write Java code that can execute SQL queries against a database. JDBC offers a consistent way to connect to various database systems, regardless of their underlying differences.
1.2. Why is JDBC important?
JDBC plays a crucial role in modern application development by enabling the seamless integration of databases. It allows developers to build applications that can store, retrieve, and manipulate data, thereby providing a dynamic and interactive user experience. Moreover, JDBC’s database-agnostic nature ensures that an application can work with different databases without major code modifications.
2. JDBC Architecture
2.1. JDBC Drivers
JDBC drivers are essential components that enable communication between Java applications and databases. There are four types of JDBC drivers:
Type 1 (JDBC-ODBC Bridge Driver): This driver translates JDBC calls into ODBC (Open Database Connectivity) calls. It’s suitable for applications that need to connect to legacy ODBC-based databases.
java // Example of Type 1 JDBC driver Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); Connection connection = DriverManager.getConnection("jdbc:odbc:DataSourceName");
Type 2 (Native-API Driver): This driver converts JDBC calls into calls for the database’s native API. It provides better performance than the Type 1 driver.
java // Example of Type 2 JDBC driver Class.forName("com.mysql.jdbc.Driver"); Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "username", "password");
Type 3 (Network Protocol Driver): Also known as the middleware driver, it converts JDBC calls into a middleware-specific protocol, which is then translated into database-specific calls.
java // Example of Type 3 JDBC driver Class.forName("com.ibm.db2.jdbc.app.DB2Driver"); Connection connection = DriverManager.getConnection("jdbc:db2://localhost:50000/mydb", "username", "password");
Type 4 (Thin Driver): This driver is purely Java-based and communicates directly with the database through the network protocol.
java // Example of Type 4 JDBC driver Class.forName("oracle.jdbc.driver.OracleDriver"); Connection connection = DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe", "username", "password");
2.2. JDBC API
The JDBC API consists of classes and interfaces that allow developers to interact with databases. Some key interfaces include Connection, Statement, PreparedStatement, CallableStatement, and ResultSet. These interfaces define methods for establishing connections, executing queries, handling results, and managing transactions.
2.3. Steps in JDBC Workflow
- Loading the Driver: The appropriate JDBC driver is loaded into memory using Class.forName() or by relying on Java’s automatic service provider mechanism.
- Establishing a Connection: A connection to the database is established using the Connection interface, obtained through the DriverManager.getConnection() method.
- Creating Statements: SQL queries are executed using either Statement for simple queries or PreparedStatement for parameterized queries.
- Executing Queries: The executeQuery() method is used to execute SELECT queries, returning a ResultSet containing the query results.
- Retrieving and Modifying Data: Data from the ResultSet can be retrieved and manipulated using various methods provided by the interface.
- Handling Exceptions: JDBC methods can throw SQLException instances, which must be handled to ensure graceful error management.
- Closing Resources: It’s essential to close Connection, Statement, and ResultSet objects to release database resources.
3. Setting up the Environment
3.1. Importing JDBC Libraries
To start using JDBC, you need to include the appropriate JDBC driver library in your project. For instance, if you’re using MySQL, you would download the MySQL Connector/J library and add it to your project’s classpath.
3.2. Database Setup
Before you can connect to a database, ensure that you have a database server running and accessible. For this example, let’s consider a MySQL database named “mydb.”
4. Establishing a Connection
4.1. Loading the Driver
Loading the JDBC driver into memory is a crucial step. This step ensures that the driver’s code is executed, and it registers itself with the DriverManager. For instance, loading the MySQL JDBC driver:
java try { Class.forName("com.mysql.cj.jdbc.Driver"); } catch (ClassNotFoundException e) { e.printStackTrace(); }
5.2. Creating a Connection
After loading the driver, you can establish a connection to the database using the DriverManager.getConnection() method. This method takes the database URL, username, and password as parameters:
java String url = "jdbc:mysql://localhost:3306/mydb"; String username = "yourUsername"; String password = "yourPassword"; try { Connection connection = DriverManager.getConnection(url, username, password); // Use the connection for database operations } catch (SQLException e) { e.printStackTrace(); }
5. Executing SQL Queries
5.1. Creating Statements
To execute SQL queries, you need to create a statement using the Statement interface:
java try { Statement statement = connection.createStatement(); // Use the statement for query execution } catch (SQLException e) { e.printStackTrace(); }
5.2. Executing Queries
Use the created statement to execute SQL queries. For instance, executing a simple SELECT query:
java try { ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); // Process the ResultSet } catch (SQLException e) { e.printStackTrace(); }
6. Retrieving and Modifying Data
6.1. Retrieving Data from ResultSet
Once you have a ResultSet after executing a SELECT query, you can retrieve data from it:
java try { ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); while (resultSet.next()) { int id = resultSet.getInt("id"); String username = resultSet.getString("username"); String email = resultSet.getString("email"); // Process the retrieved data } } catch (SQLException e) { e.printStackTrace(); }
6.2. Updating Data
For data modification queries (INSERT, UPDATE, DELETE), you can use the executeUpdate() method of the Statement interface:
java try { int rowsAffected = statement.executeUpdate("UPDATE users SET email = 'new@email.com' WHERE id = 1"); // Process the update result } catch (SQLException e) { e.printStackTrace(); }
7. Handling Exceptions
7.1. SQLException
JDBC methods can throw SQLException when something goes wrong during database operations. It’s important to handle these exceptions to provide meaningful feedback to users and ensure proper error management.
7.2. Best Practices for Exception Handling
- Use try-catch blocks to handle exceptions.
- Close resources (connection, statement, result set) in the finally block to ensure they are released.
- Log exceptions for troubleshooting.
8. Closing Resources
8.1. Closing Connections
Always close the database connection once you’re done using it:
java try { connection.close(); } catch (SQLException e) { e.printStackTrace(); }
8.2. Closing Statements and ResultSets
Close statements and result sets in a similar manner:
java try { statement.close(); resultSet.close(); } catch (SQLException e) { e.printStackTrace(); }
9. Advanced JDBC Features
9.1. Prepared Statements
Prepared statements allow you to use parameterized queries, improving efficiency and security:
java String sql = "SELECT * FROM users WHERE username = ?"; try { PreparedStatement preparedStatement = connection.prepareStatement(sql); preparedStatement.setString(1, "john_doe"); ResultSet resultSet = preparedStatement.executeQuery(); // Process the result } catch (SQLException e) { e.printStackTrace(); }
9.2. Batch Processing
Batch processing enables you to execute multiple queries as a batch, reducing the number of round-trips to the database:
java try { connection.setAutoCommit(false); Statement statement = connection.createStatement(); statement.addBatch("INSERT INTO users (username, email) VALUES ('user1', 'user1@email.com')"); statement.addBatch("INSERT INTO users (username, email) VALUES ('user2', 'user2@email.com')"); int[] batchResults = statement.executeBatch(); connection.commit(); } catch (SQLException e) { e.printStackTrace(); }
9.3. Transactions
Transactions ensure data consistency by grouping multiple database operations into a single unit of work:
java try { connection.setAutoCommit(false); // Perform multiple database operations connection.commit(); // Commit the transaction } catch (SQLException e) { connection.rollback(); // Rollback the transaction if an error occurs e.printStackTrace(); }
10. Sample Application: User Management System
10.1. Database Schema
Consider a simple user management system with a “users” table:
sql CREATE TABLE users ( id INT PRIMARY KEY, username VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL );
10.2. Implementing JDBC Operations
Here’s a high-level overview of implementing JDBC operations in the user management system:
- Load the JDBC driver.
- Establish a connection.
- Create statements and execute queries.
- Retrieve and display user data.
- Update user data.
- Close resources.
Conclusion
Java Database Connectivity (JDBC) is a fundamental technology for integrating Java applications with relational databases. Its flexibility, portability, and database-agnostic nature make it a powerful tool for developers to manage and manipulate data seamlessly. By understanding the architecture, workflow, and advanced features of JDBC, developers can build robust and efficient database-driven applications. Through this comprehensive guide, you’ve gained the knowledge and tools to embark on your journey of successful database integration with Java.
Table of Contents
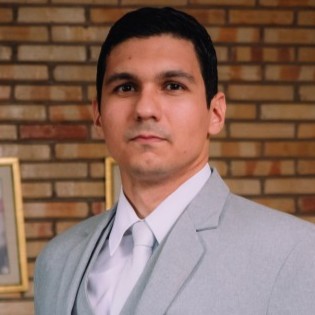
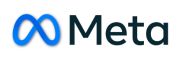