Debugging Java Applications: Tips and Tricks
Debugging is an essential part of the software development process. It’s the detective work that developers undertake to identify and fix issues in their code. When it comes to Java applications, debugging can sometimes feel like navigating a maze, especially when dealing with complex systems. In this blog post, we’ll delve into some valuable tips and tricks that can help you debug Java applications more effectively and efficiently.
Table of Contents
1. Understanding the Debugger
Before we dive into specific techniques, let’s briefly discuss the debugger itself. The debugger is a tool that allows developers to track the execution of their code, set breakpoints, inspect variables, and step through the code line by line. Familiarizing yourself with the debugger’s interface and capabilities is crucial for effective debugging.
1.1. Utilize Breakpoints Strategically
Breakpoints are your allies in debugging Java applications. Placing breakpoints at strategic points in your code allows you to pause the execution and inspect the state of variables, helping you pinpoint the root cause of issues. Use breakpoints to stop execution at critical junctures, such as the beginning of a problematic function or loop.
java public class Example { public static void main(String[] args) { int x = 10; int y = 20; int result = x + y; // Set breakpoint here System.out.println(result); } }
1.2. Leverage Conditional Breakpoints
Conditional breakpoints take breakpoint usage to the next level. They allow you to specify a condition that must be met for the breakpoint to be triggered. This can be incredibly useful when you’re trying to debug a specific scenario or when a certain value is causing issues.
java public class Example { public static void main(String[] args) { for (int i = 0; i < 10; i++) { System.out.println(i); } // Set conditional breakpoint with condition i == 5 } }
1.3. Print Debugging
Sometimes, a simple System.out.println() statement can provide valuable insights into what’s happening in your code. While more sophisticated logging frameworks exist, using print statements for quick and dirty debugging can be effective, especially in scenarios where setting up a logger might be overkill.
java public class Example { public static void main(String[] args) { String message = "Debugging is fun!"; System.out.println("Message: " + message); } }
1.4. Exception Breakpoints
Java applications often encounter exceptions, and tracking down the source of an exception can be challenging. Utilize exception breakpoints to pause execution whenever a specific exception is thrown. This can help you catch exceptions at the exact point where they occur, making diagnosis much easier.
java public class Example { public static void main(String[] args) { try { int result = divide(10, 0); System.out.println(result); } catch (ArithmeticException e) { // Set exception breakpoint here System.out.println("Division by zero!"); } } public static int divide(int a, int b) { return a / b; } }
1.5. Explore Data with Watches
Watches are a powerful feature of debuggers that allow you to monitor the value of variables in real-time. Instead of manually adding print statements, you can add variables to your watch list, and their values will be displayed as you step through the code.
1.6. Take Advantage of Stack Traces
Stack traces provide a wealth of information about the sequence of method calls that led to an issue. When an exception occurs, examining the stack trace can help you trace the path the program took to reach that point. This can be immensely valuable in identifying where the problem originates.
1.7. Remote Debugging
In some cases, issues might only arise in specific environments or scenarios. Remote debugging allows you to attach a debugger to a running Java application, even if it’s on a different machine. This enables you to diagnose problems that only manifest in certain circumstances.
1.8. Profiling Tools for Performance Issues
Debugging isn’t just about fixing bugs; it’s also about optimizing your code’s performance. Profiling tools can help you identify bottlenecks, memory leaks, and other performance-related issues. Tools like Java VisualVM and YourKit Java Profiler can provide deep insights into your application’s runtime behavior.
1.9. Unit Testing for Preventive Debugging
Unit tests aren’t just for verifying that your code works; they can also act as a preventive measure against future bugs. By writing comprehensive unit tests, you can catch issues early in the development cycle and ensure that changes to your codebase don’t introduce regressions.
1.10. Continuous Integration and Debugging
Integrating debugging practices into your continuous integration (CI) pipeline is crucial. Automated tests that include debugging techniques can catch problems early, ensuring that new code changes don’t break existing functionality. This tight feedback loop minimizes the chances of introducing new bugs.
Conclusion
Debugging Java applications can be a challenging yet rewarding endeavor. Armed with the right tools, techniques, and mindset, you can effectively navigate the complexities of your codebase and identify and resolve issues efficiently. Whether it’s through strategic breakpoint placement, leveraging conditional breakpoints, or using print statements for quick insights, debugging remains an integral part of the development process. Embrace these tips and tricks, and you’ll be well-equipped to tackle even the most elusive bugs in your Java applications. Happy debugging!
Remember, debugging is a skill that improves with practice. Each debugging session is a learning opportunity, helping you gain a deeper understanding of your codebase and sharpening your troubleshooting abilities. So, dive into your Java applications with confidence, knowing that you have a powerful set of debugging tools at your disposal.
Table of Contents
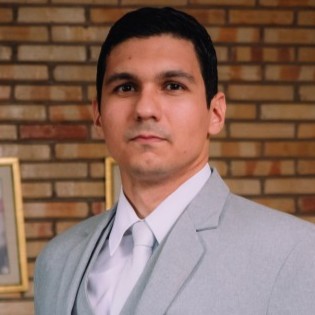
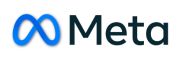