Building Desktop Applications with JavaFX and Scene Builder
In the ever-evolving landscape of software development, desktop applications continue to hold their ground as reliable tools for a wide range of tasks. JavaFX, coupled with Scene Builder, offers developers a robust framework for creating visually appealing and interactive desktop applications. In this guide, we’ll dive into the world of JavaFX and Scene Builder, exploring their capabilities, and providing a comprehensive tutorial for building your own desktop applications.
Table of Contents
1. Introduction to JavaFX and Scene Builder
1.1. What is JavaFX?
JavaFX is a Java library that enables developers to build rich client applications with visually appealing user interfaces. It provides a comprehensive set of UI controls, layout containers, and multimedia integration capabilities. JavaFX allows developers to create cross-platform applications that can run on Windows, macOS, and Linux.
1.2. What is Scene Builder?
Scene Builder is a visual layout tool provided by Oracle to design JavaFX application user interfaces. It allows developers to create UIs by dragging and dropping components onto a canvas, visually setting properties, and arranging elements. Scene Builder generates FXML, a declarative markup language, which describes the UI layout. This separation of design and logic enhances collaboration between designers and developers.
1.3. Why use JavaFX and Scene Builder?
JavaFX offers a plethora of benefits for desktop application development. Its rich set of UI controls enables the creation of intuitive interfaces. JavaFX also supports animations, transitions, and multimedia integration, enhancing the user experience. As for Scene Builder, its visual approach streamlines UI design and promotes cleaner code architecture through the use of FXML.
In the following sections, we’ll delve into the practical aspects of using JavaFX and Scene Builder to build desktop applications.
2. Setting Up Your Development Environment
Before we begin building applications, let’s set up the development environment.
2.1. Installing Java Development Kit (JDK)
JavaFX requires a compatible Java Development Kit (JDK). Download and install the latest version of the JDK from the official Oracle website or use a package manager if you’re on Linux.
2.2. Downloading and Installing JavaFX SDK
Next, download the JavaFX SDK from the official OpenJFX website. Extract the SDK to a location on your machine. We’ll need to configure our project to use the JavaFX libraries from this SDK.
2.3. Installing Scene Builder
Download and install Scene Builder from the official Gluon website. Once installed, Scene Builder will automatically integrate with your JavaFX SDK.
With your development environment ready, we can now proceed to create our first JavaFX application.
3. Creating Your First JavaFX Application
Let’s create a simple “Hello World” application to get started.
3.1. Initializing a New JavaFX Project
In your preferred Integrated Development Environment (IDE), create a new JavaFX project. Configure the project to use the JDK and JavaFX SDK you’ve installed.
3.2. Structuring the Project Directory
A typical JavaFX project has a well-defined structure. You’ll have a source folder for your Java code and a resources folder for your FXML files and other assets. This separation keeps your code organized.
plaintext MyJavaFXApp/ ??? src/ ? ??? main/ ? ? ??? java/ ? ? ? ??? com/ ? ? ? ??? myapp/ ? ? ? ??? Main.java ? ? ??? resources/ ? ? ??? com/ ? ? ??? myapp/ ? ? ??? main.fxml ??? …
3.3. Building the Main UI Components
Open the main.fxml file in Scene Builder. Drag and drop UI components onto the canvas. For our “Hello World” app, let’s add a Label and a Button.
fxml <VBox alignment="CENTER" xmlns="http://javafx.com/javafx/16" xmlns:fx="http://javafx.com/fxml/1"> <Label text="Hello, JavaFX!" /> <Button text="Click Me" /> </VBox>
3.4. Adding Functionality to UI Elements
In the Main.java class, load the FXML file and set up the stage:
java public class Main extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) throws IOException { FXMLLoader loader = new FXMLLoader(getClass().getResource("/com/myapp/main.fxml")); Parent root = loader.load(); primaryStage.setScene(new Scene(root)); primaryStage.setTitle("My JavaFX App"); primaryStage.show(); } }
Congratulations! You’ve created your first JavaFX application. Run the program, and you’ll see your UI components displayed.
In the upcoming sections, we’ll explore more advanced features of JavaFX and Scene Builder, such as event handling, custom UI components, and packaging applications for distribution.
Conclusion
JavaFX and Scene Builder provide a powerful combination for building desktop applications with rich user interfaces. With JavaFX’s versatile UI controls and Scene Builder’s visual design capabilities, developers can create applications that are not only functional but also visually appealing.
Throughout this guide, we’ve touched on various aspects of building desktop applications with JavaFX and Scene Builder. From setting up your environment to designing UIs, handling events, and packaging applications, you now have a solid foundation to embark on your JavaFX journey.
As you continue your exploration of JavaFX and Scene Builder, remember that practice makes perfect. The more you experiment with different UI components, animations, and interactions, the more proficient you’ll become in creating stunning desktop applications. So go ahead, unleash your creativity, and build desktop applications that leave a lasting impression. Happy coding!
Table of Contents
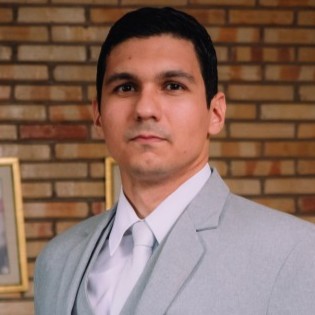
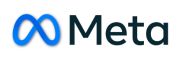