Java Networking: Building Distributed Applications
In today’s interconnected world, building distributed applications that seamlessly communicate across various devices and systems is essential. Java, with its robust networking capabilities, offers a versatile platform for creating such applications. In this blog post, we will delve into the world of Java networking and explore how to build distributed applications that facilitate efficient data exchange between different components.
Table of Contents
1. Understanding Java Networking Basics
At the heart of Java’s networking capabilities are the classes and APIs that enable communication over networks. Java provides the java.net package, which contains classes for working with networking elements such as sockets, URLs, and connections.
1.1. Sockets: The Foundation of Network Communication
Sockets serve as the foundation of network communication in Java. They allow two-way communication between a client and a server, enabling data to flow seamlessly between them. Java offers two types of sockets: the Socket class for client-side communication and the ServerSocket class for server-side communication.
Let’s take a look at a basic example of a client-server interaction using sockets:
Client Code:
java import java.io.*; import java.net.*; public class Client { public static void main(String[] args) { try { Socket socket = new Socket("localhost", 8080); OutputStream out = socket.getOutputStream(); PrintWriter writer = new PrintWriter(out, true); writer.println("Hello, server!"); socket.close(); } catch (IOException e) { e.printStackTrace(); } } }
Server Code:
java import java.io.*; import java.net.*; public class Server { public static void main(String[] args) { try { ServerSocket serverSocket = new ServerSocket(8080); System.out.println("Server listening on port 8080..."); Socket clientSocket = serverSocket.accept(); InputStream in = clientSocket.getInputStream(); BufferedReader reader = new BufferedReader(new InputStreamReader(in)); String message = reader.readLine(); System.out.println("Received message: " + message); clientSocket.close(); serverSocket.close(); } catch (IOException e) { e.printStackTrace(); } } }
This example demonstrates a simple client-server interaction using sockets. The client establishes a connection to the server and sends a message, which the server then receives and displays.
2. Networking APIs for Different Use Cases
Java provides various networking APIs to accommodate different networking scenarios. Let’s explore a few key APIs:
2.1. URL and HttpURLConnection
The URL class in Java is used to represent URLs, providing a convenient way to interact with web resources. The HttpURLConnection class, an extension of the URLConnection class, enables communication with HTTP resources. Here’s a brief example:
java import java.io.*; import java.net.*; public class URLExample { public static void main(String[] args) { try { URL url = new URL("https://www.example.com"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } connection.disconnect(); } catch (IOException e) { e.printStackTrace(); } } }
In this example, the program accesses the content of a web page using the URL and HttpURLConnection classes.
2.2. DatagramSocket and DatagramPacket
For communication scenarios where reliability is less critical, such as real-time applications or broadcasting, Java provides the DatagramSocket and DatagramPacket classes. These classes allow communication using the User Datagram Protocol (UDP). Here’s a basic usage example:
java import java.io.*; import java.net.*; public class UDPClient { public static void main(String[] args) { try { DatagramSocket socket = new DatagramSocket(); String message = "Hello, UDP Server!"; byte[] data = message.getBytes(); InetAddress serverAddress = InetAddress.getByName("localhost"); DatagramPacket packet = new DatagramPacket(data, data.length, serverAddress, 9876); socket.send(packet); socket.close(); } catch (IOException e) { e.printStackTrace(); } } } java import java.io.*; import java.net.*; public class UDPServer { public static void main(String[] args) { try { DatagramSocket socket = new DatagramSocket(9876); byte[] buffer = new byte[1024]; DatagramPacket packet = new DatagramPacket(buffer, buffer.length); socket.receive(packet); String receivedMessage = new String(packet.getData(), 0, packet.getLength()); System.out.println("Received message: " + receivedMessage); socket.close(); } catch (IOException e) { e.printStackTrace(); } } }
In this example, the client sends a message to the server using UDP, and the server receives and displays the message.
3. Best Practices for Java Networking
Building distributed applications with Java networking involves more than just writing code. It’s essential to follow best practices to ensure robust, secure, and efficient communication:
3.1. Use Threads for Concurrency
When building server applications that handle multiple client connections, using threads is crucial to ensure responsiveness. Each client connection can be handled in a separate thread, preventing one slow client from blocking others.
3.2. Implement Error Handling
Network communication is susceptible to errors, such as connection drops or timeouts. Implement comprehensive error handling mechanisms to gracefully handle such situations and provide meaningful feedback to users.
3.3. Consider Security
Network security is paramount, especially when transmitting sensitive data. Use secure protocols such as HTTPS and implement encryption mechanisms to protect data in transit.
3.4. Optimize Data Serialization
Efficient data serialization is essential for reducing the size of data transmitted over the network. Consider using protocols like Protocol Buffers or JSON for serialization, depending on the use case.
3.5. Monitor and Tune Performance
Regularly monitor network performance and identify bottlenecks or latency issues. Use tools and techniques to optimize network performance for the best user experience.
Conclusion
Java networking empowers developers to create distributed applications that communicate seamlessly over networks. By understanding the basics of sockets, exploring different networking APIs, and following best practices, you can build robust and efficient distributed applications that cater to a wide range of use cases. Whether you’re developing web applications, real-time systems, or IoT devices, Java’s networking capabilities have you covered. So, go ahead and leverage the power of Java to build your next generation of interconnected applications.
Table of Contents
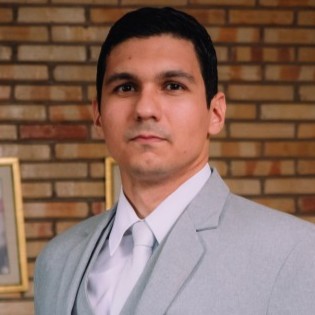
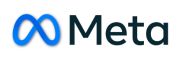